This document is for developers who want to use the AdSense Host API to get information about their AdSense account. This document assumes that you're familiar with web programming concepts and web data formats.
Before you start
Get an AdSense account
You need an AdSense account for testing purposes. If you already have a test account, then you're all set; you can visit the AdSense user interface to set up, edit, or view your test data.
Get familiar with AdSense
If you're not familiar with AdSense concepts read the introductory information on AdSense and experiment with the user interface before starting to code.
Register your application
To use the AdSense Host API you must register the application you're developing by creating a project and generating a Client ID.
Register your appEdit the configuration files for your client library project with the new credentials you just created. Check the client library documentation for more details.
Note: The Google account used for registration should be your developer account, that is, the account that you wish users of your application to see as the developer of the application. This account does not need to be tied to an AdSense login, as users will be granting access to their own accounts while using the application.
Understanding the AdSense Host API
Basic concepts
The AdSense Host API is built upon some basic AdSense concepts:
- Ad Clients: Ad clients represent an association between an AdSense account and an AdSense product, such as Content Ads or Search Ads. An AdSense account can have one or multiple ad clients.
- Channels: Channels are tools that let you track the performance of a specific page or domain.
- Reports: Reports give you insight into what you're earning, as well as what's having an impact on those earnings. They can be run on an entire account, or on a subset of your inventory, through the use of channels.
Supported operations
The operations currently supported by the API are described in the table below.
Operation | Description | REST HTTP mappings |
---|---|---|
list | Lists all resources within a collection. | GET on a collection URI. |
get | Gets a specific resource. | GET on a resource URI. |
generate | Generate a result set based on a specified resource. Used only for reports. | GET/POST on a collection URI, where you pass in the resource as
a definition for the generation. |
All operations require authentication.
Calling style
REST is a style of software architecture that provides a convenient and consistent approach to requesting and modifying data.
The term REST is short for "Representational State Transfer." In the context of Google APIs, it refers to using HTTP verbs to retrieve and modify representations of data stored by Google.
In a RESTful system, resources are stored in a data store; a client sends a request that the server perform a particular action (such as creating, retrieving, updating, or deleting a resource), and the server performs the action and sends a response, often in the form of a representation of the specified resource.
In Google's RESTful APIs, the client specifies an action using an HTTP verb such as POST
, GET
, PUT
, or DELETE
. It specifies a resource by a globally-unique URI of the following form:
https://www.googleapis.com/apiName/apiVersion/resourcePath?parameters
Because all API resources have unique HTTP-accessible URIs, REST enables data caching and is optimized to work with the web's distributed infrastructure.
You may find the method definitions in the HTTP 1.1 standards documentation useful; they include specifications for GET
, POST
, PUT
, and DELETE
.
REST in the AdSense Host API
The supported operations map directly to REST HTTP verbs, as described in AdSense Host API operations.
The specific format for AdSense Host API URIs are:
https://www.googleapis.com/adsensehost/v4.1/resourceID?parameters
where resourceID
is the identifier for an ad
client, url channel, custom channel or the reports collection, and
parameters
are any parameters to apply to
the query.
The format of the resourceID
path extensions
lets you identify the resource on which you are currently operating, for
example:
https://www.googleapis.com/adsensehost/v4.1/adclients https://www.googleapis.com/adsensehost/v4.1/adclients/adClientId https://www.googleapis.com/adsensehost/v4.1/adclients/adClientId/urlchannels ...
The full set of URIs used for each supported operation in the API is summarized in the AdSense Host API Reference document.
Here is an example of how this works in the AdSense Host API.
List ad clients:
GET https://www.googleapis.com/adsensehost/v4.1/adclients/
Data format
JSON (JavaScript Object Notation) is a common, language-independent data format that provides a simple text representation of arbitrary data structures. For more information, see json.org.
Making requests
Authorizing requests
Every request your application sends to the AdSense Host API must include an authorization token. The token also identifies your application to Google.
About authorization protocols
Your application must use OAuth 2.0 to authorize requests. No other authorization protocols are supported. If your application uses Sign In With Google, some aspects of authorization are handled for you.
Authorizing requests with OAuth 2.0
All requests to the AdSense Host API must be authorized by an authenticated user.
This process is facilitated with an OAuth client ID.
Get an OAuth client IDOr create one in the Credentials page.
The details of the authorization process, or "flow," for OAuth 2.0 vary somewhat depending on what kind of application you're writing. The following general process applies to all application types:
- When your application needs access to user data, it asks Google for a particular scope of access.
- Google displays a consent screen to the user, asking them to authorize your application to request some of their data.
- If the user approves, then Google gives your application a short-lived access token.
- Your application requests user data, attaching the access token to the request.
- If Google determines that your request and the token are valid, it returns the requested data.
Some flows include additional steps, such as using refresh tokens to acquire new access tokens. For detailed information about flows for various types of applications, see Google's OAuth 2.0 documentation.
Here's the OAuth 2.0 scope information for the AdSense Host API:
Scope | Meaning |
---|---|
https://www.googleapis.com/auth/adsensehost |
Read/write access to AdSense data. |
To request access using OAuth 2.0, your application needs the scope information, as well as information that Google supplies when you register your application (such as the client ID and the client secret).
Tip: The Google APIs client libraries can handle some of the authorization process for you. They are available for a variety of programming languages; check the page with libraries and samples for more details.
See the OAuth 2.0 section for more information.
Making a request
The final step is making the API request. If you're not using any client libraries, you can take a look at the reference documentation.
If you're using the client libraries, this task is significantly easier. See Samples and Libraries for a complete guide of how to make your first request with the Java, Python, PHP or JavaScript client library.
OAuth 2.0
Authentication and authorization in the AdSense Host API are handled by OAuth 2.0. However, due to a few particularities of the AdSense Host usage model, things may be handled a little bit differently than what you’re used to in other Google APIs.
Participants
There are three entities involved in an OAuth 2.0 request:
- the user, who’s granting access to personal data
- the developer, who through the application is requesting access to the user data
- Google, who both authenticates the parties and provides the API service.
In the case of the AdSense Host API, however, the user and the developer are actually the same person, since you as a developer will be using the API to get access to your own AdSense account and, through it, your publishers’ accounts.
Creating a project
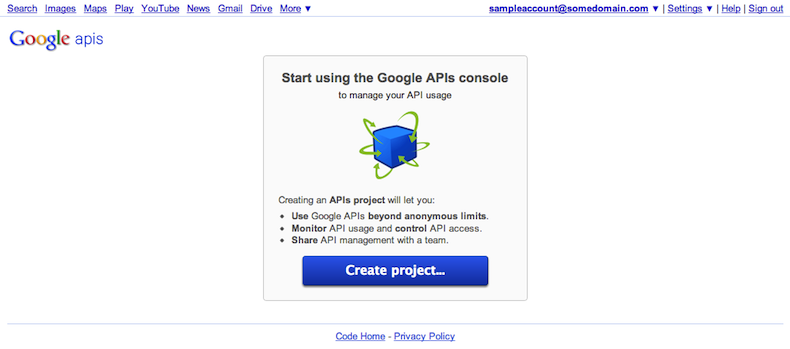
Before you start, you’ll need to create a new project on your APIs console, as you would for any other Google API you use. The Google account you use to create the developer project needs to be whitelisted for access to the AdSense Host API, so be sure you’re either using the sandbox account we created for you during signup, or your production AdSense account if you’ve already finished implementing the API and gone live.
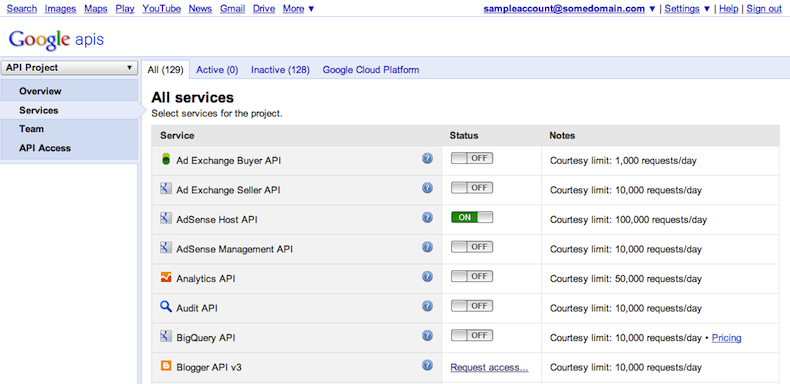
You can find more information on creating Google APIs projects in the APIs Console documentation.
Setting up API access
Once you have a project, you need to enable API access on it by creating an OAuth 2.0 client ID.
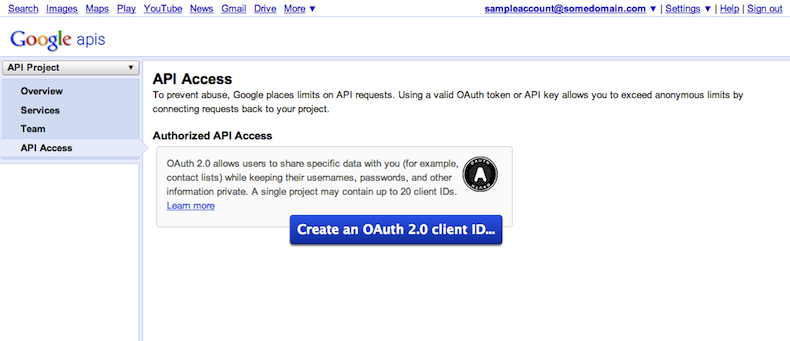
You have several choices here, but we recommend you create an Installed Application project, as it makes the next steps easier.
Authenticate once, store forever
You’ll now need to authenticate using your own AdSense account, which you can do with a small script or a test application (check our sample code for examples).
After authenticating, make sure you store the refresh token you got back from the authorization step. This is a long-lived token which won’t expire unless access is explicitly revoked, and since you are the user, you shouldn’t be revoking access to your own application!
You should save the refresh token in your application’s data store for all future authentication requests.
Using the saved token in your application
Once you have a refresh token, it no longer matters whether it was generated in a Web Server Application flow or an Installed Application flow. You can simply use it to make token refresh requests for new access tokens as needed. If you’re using one of our client libraries, check the documentation to see how to specify your own tokens; you may need to edit an automatically-generated file or a database entry.
Wrapping it up
The end result is that the OAuth 2.0 flow is only executed once, and after that all requests happen in the background, without any manual intervention by the end user of your site (the publisher) or you, as the developer.