Dialogflow で探索
[続行] をクリックして、Dialogflow に Responses サンプルをインポートします。次に、 サンプルをデプロイしてテストする手順は次のとおりです。
- エージェント名を入力し、サンプルの新しい Dialogflow エージェントを作成します。
- エージェントのインポートが完了したら、[Go to agent] をクリックします。
- メインのナビゲーション メニューから [Fulfillment] に移動します。
- [Inline Editor] を有効にして、[Deploy] をクリックします。エディタにはサンプルが含まれています。 できます。
- メイン ナビゲーション メニューから [Integrations] に移動し、[Google] をクリックします アシスタント。
- 表示されたモーダル ウィンドウで、[変更の自動プレビュー] を有効にして [テスト] をクリックします。 Actions シミュレータを開きます
- シミュレータで「
Talk to my test app
」と入力して、サンプルをテストします。
視覚的なチャットのふきだし形式で、テキスト読み上げを使用する 音声については、音声合成マークアップ言語(SSML)を使用します。
TTS テキストはデフォルトでチャットふきだしのコンテンツとして使用されます。視覚的要素が 表示テキストを指定する必要はありません。 クリックします。
会話の設計ガイドラインもあわせてご覧ください。 これらの視覚要素をアクションに組み込む方法を紹介します
プロパティ
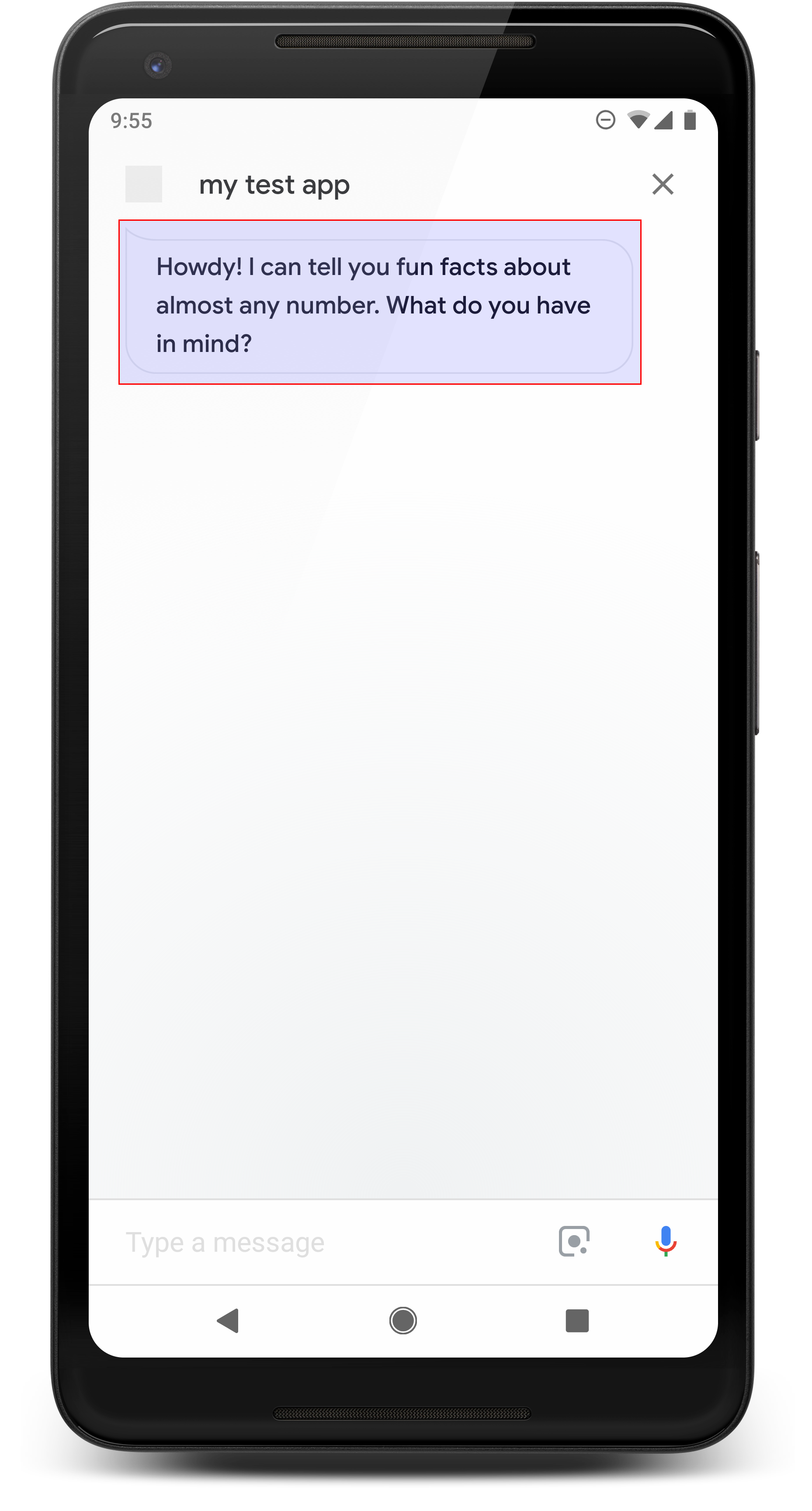
シンプル レスポンスには以下の要件があり、必要に応じてオプションのプロパティを設定できます。
actions.capability.AUDIO_OUTPUT
またはactions.capability.SCREEN_OUTPUT
機能。チャットふきだしあたりの文字数制限は 640 文字です。この制限より長い文字列は、640 番目の文字より前にある最初の単語の区切り(または空白)以降が切り捨てられます。
チャットふきだしの内容は音声のサブセットにするか、TTS / SSML 出力全体を文字に起こしたものにする必要があります。そうすることで、アクションの発言の筋道をとらえやすくなり、さまざまな状況でユーザーの理解が向上します。
チャットふきだしの数は、1 ターンあたり多くても 2 つまでにします。
Google に提出するチャットヘッド(ロゴ)はサイズを 192x192 ピクセルにする必要があり、アニメーション化することはできません。
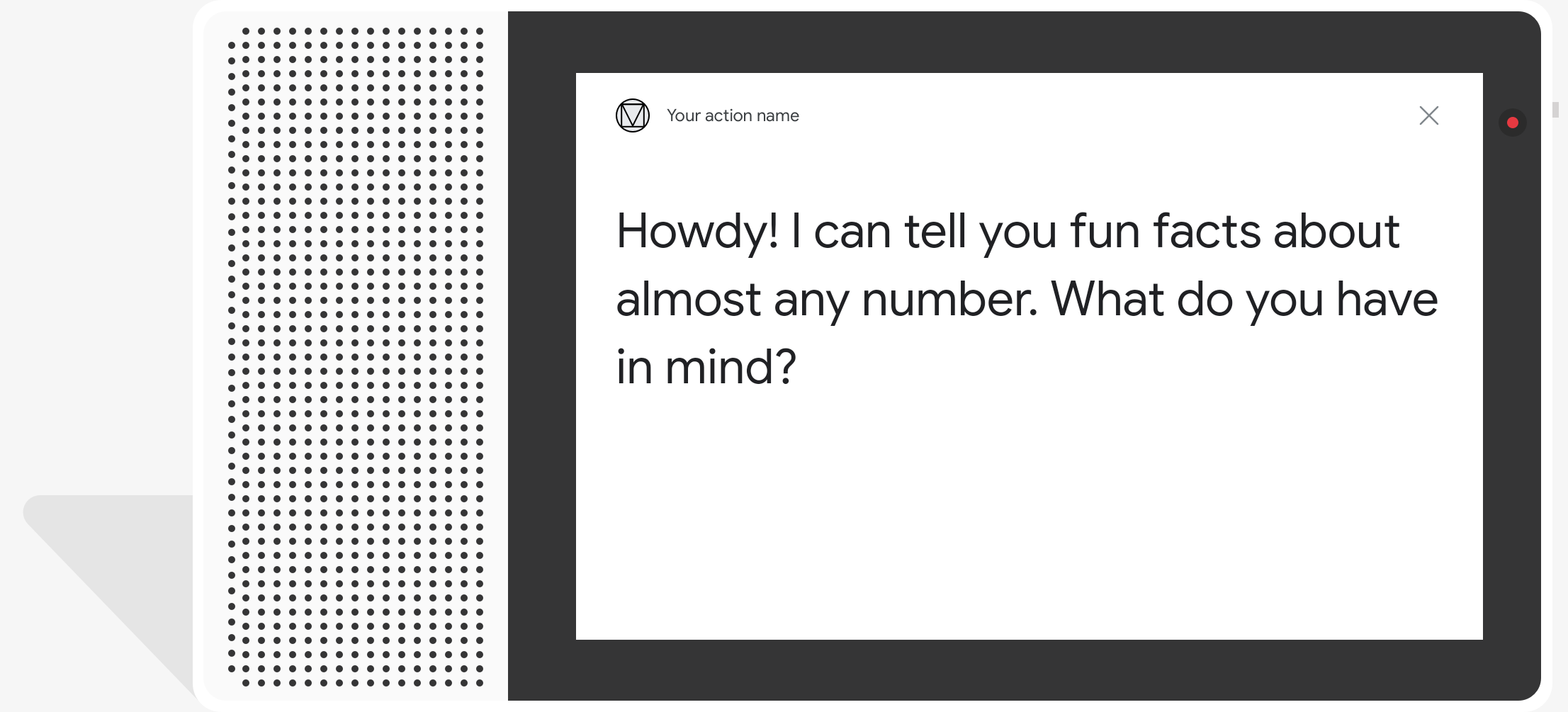
サンプルコード
Node.js
app.intent('Simple Response', (conv) => { conv.ask(new SimpleResponse({ speech: `Here's an example of a simple response. ` + `Which type of response would you like to see next?`, text: `Here's a simple response. ` + `Which response would you like to see next?`, })); });
Java
@ForIntent("Simple Response") public ActionResponse welcome(ActionRequest request) { ResponseBuilder responseBuilder = getResponseBuilder(request); responseBuilder.add( new SimpleResponse() .setTextToSpeech( "Here's an example of a simple response. " + "Which type of response would you like to see next?") .setDisplayText( "Here's a simple response. Which response would you like to see next?")); return responseBuilder.build(); }
Node.js
conv.ask(new SimpleResponse({ speech: `Here's an example of a simple response. ` + `Which type of response would you like to see next?`, text: `Here's a simple response. ` + `Which response would you like to see next?`, }));
Java
ResponseBuilder responseBuilder = getResponseBuilder(request); responseBuilder.add( new SimpleResponse() .setTextToSpeech( "Here's an example of a simple response. " + "Which type of response would you like to see next?") .setDisplayText( "Here's a simple response. Which response would you like to see next?")); return responseBuilder.build();
JSON
下記の JSON は Webhook レスポンスを示します。
{ "payload": { "google": { "expectUserResponse": true, "richResponse": { "items": [ { "simpleResponse": { "textToSpeech": "Here's an example of a simple response. Which type of response would you like to see next?", "displayText": "Here's a simple response. Which response would you like to see next?" } } ] } } } }
JSON
下記の JSON は Webhook レスポンスを示します。
{ "expectUserResponse": true, "expectedInputs": [ { "possibleIntents": [ { "intent": "actions.intent.TEXT" } ], "inputPrompt": { "richInitialPrompt": { "items": [ { "simpleResponse": { "textToSpeech": "Here's an example of a simple response. Which type of response would you like to see next?", "displayText": "Here's a simple response. Which response would you like to see next?" } } ] } } } ] }
SSML と音声
レスポンスに SSML と音声を使用すると、より洗練され、 向上させることができます次のコード スニペットは、レスポンスを作成する方法を示しています。 :
Node.js
app.intent('SSML', (conv) => { conv.ask(`<speak>` + `Here are <say-as interpet-as="characters">SSML</say-as> examples.` + `Here is a buzzing fly ` + `<audio src="https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg"></audio>` + `and here's a short pause <break time="800ms"/>` + `</speak>`); conv.ask('Which response would you like to see next?'); });
Java
@ForIntent("SSML") public ActionResponse ssml(ActionRequest request) { ResponseBuilder responseBuilder = getResponseBuilder(request); responseBuilder.add( "<speak>" + "Here are <say-as interpet-as=\"characters\">SSML</say-as> examples." + "Here is a buzzing fly " + "<audio src=\"https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg\"></audio>" + "and here's a short pause <break time=\"800ms\"/>" + "</speak>"); return responseBuilder.build(); }
Node.js
conv.ask(`<speak>` + `Here are <say-as interpet-as="characters">SSML</say-as> examples.` + `Here is a buzzing fly ` + `<audio src="https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg"></audio>` + `and here's a short pause <break time="800ms"/>` + `</speak>`); conv.ask('Which response would you like to see next?');
Java
ResponseBuilder responseBuilder = getResponseBuilder(request); responseBuilder.add( "<speak>" + "Here are <say-as interpet-as=\"characters\">SSML</say-as> examples." + "Here is a buzzing fly " + "<audio src=\"https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg\"></audio>" + "and here's a short pause <break time=\"800ms\"/>" + "</speak>"); return responseBuilder.build();
JSON
下記の JSON は Webhook レスポンスを示します。
{ "payload": { "google": { "expectUserResponse": true, "richResponse": { "items": [ { "simpleResponse": { "textToSpeech": "<speak>Here are <say-as interpet-as=\"characters\">SSML</say-as> examples.Here is a buzzing fly <audio src=\"https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg\"></audio>and here's a short pause <break time=\"800ms\"/></speak>" } }, { "simpleResponse": { "textToSpeech": "Which response would you like to see next?" } } ] } } } }
JSON
下記の JSON は Webhook レスポンスを示します。
{ "expectUserResponse": true, "expectedInputs": [ { "possibleIntents": [ { "intent": "actions.intent.TEXT" } ], "inputPrompt": { "richInitialPrompt": { "items": [ { "simpleResponse": { "textToSpeech": "<speak>Here are <say-as interpet-as=\"characters\">SSML</say-as> examples.Here is a buzzing fly <audio src=\"https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg\"></audio>and here's a short pause <break time=\"800ms\"/></speak>" } }, { "simpleResponse": { "textToSpeech": "Which response would you like to see next?" } } ] } } } ] }
詳細については、SSML リファレンス ドキュメントをご覧ください。
サウンド ライブラリ
YouTube のサウンド ライブラリでは、さまざまな短いサウンドを無料で提供しています。これらの サウンドは自動でホストされるため、必要な作業は SSML に含めることだけです。