در Dialogflow کاوش کنید
برای وارد کردن نمونه پاسخ های ما در Dialogflow روی Continue کلیک کنید. سپس، مراحل زیر را برای استقرار و آزمایش نمونه دنبال کنید:
- یک نام عامل وارد کنید و یک عامل Dialogflow جدید برای نمونه ایجاد کنید.
- پس از وارد کردن عامل، روی Go to agent کلیک کنید.
- از منوی پیمایش اصلی، به Fulfillment بروید.
- ویرایشگر درون خطی را فعال کنید، سپس روی Deploy کلیک کنید. ویرایشگر حاوی کد نمونه است.
- از منوی پیمایش اصلی، به Integrations بروید، سپس روی Google Assistant کلیک کنید.
- در پنجره مدال که ظاهر میشود، پیشنمایش خودکار تغییرات را فعال کنید و روی Test کلیک کنید تا شبیهساز Actions باز شود.
- در شبیه ساز برای تست نمونه وارد
Talk to my test app
شوید!
پاسخهای ساده از نظر بصری به شکل حباب چت هستند و از متن به گفتار (TTS) یا زبان نشانهگذاری ترکیبی گفتار (SSML) برای صدا استفاده میکنند.
متن TTS به طور پیش فرض به عنوان محتوای حباب چت استفاده می شود. اگر جنبه بصری آن متن نیازهای شما را برآورده کند، نیازی به تعیین متن نمایشی برای حباب چت نخواهید داشت.
همچنین میتوانید دستورالعملهای طراحی مکالمه ما را مرور کنید تا یاد بگیرید چگونه این عناصر بصری را در Action خود بگنجانید.
خواص
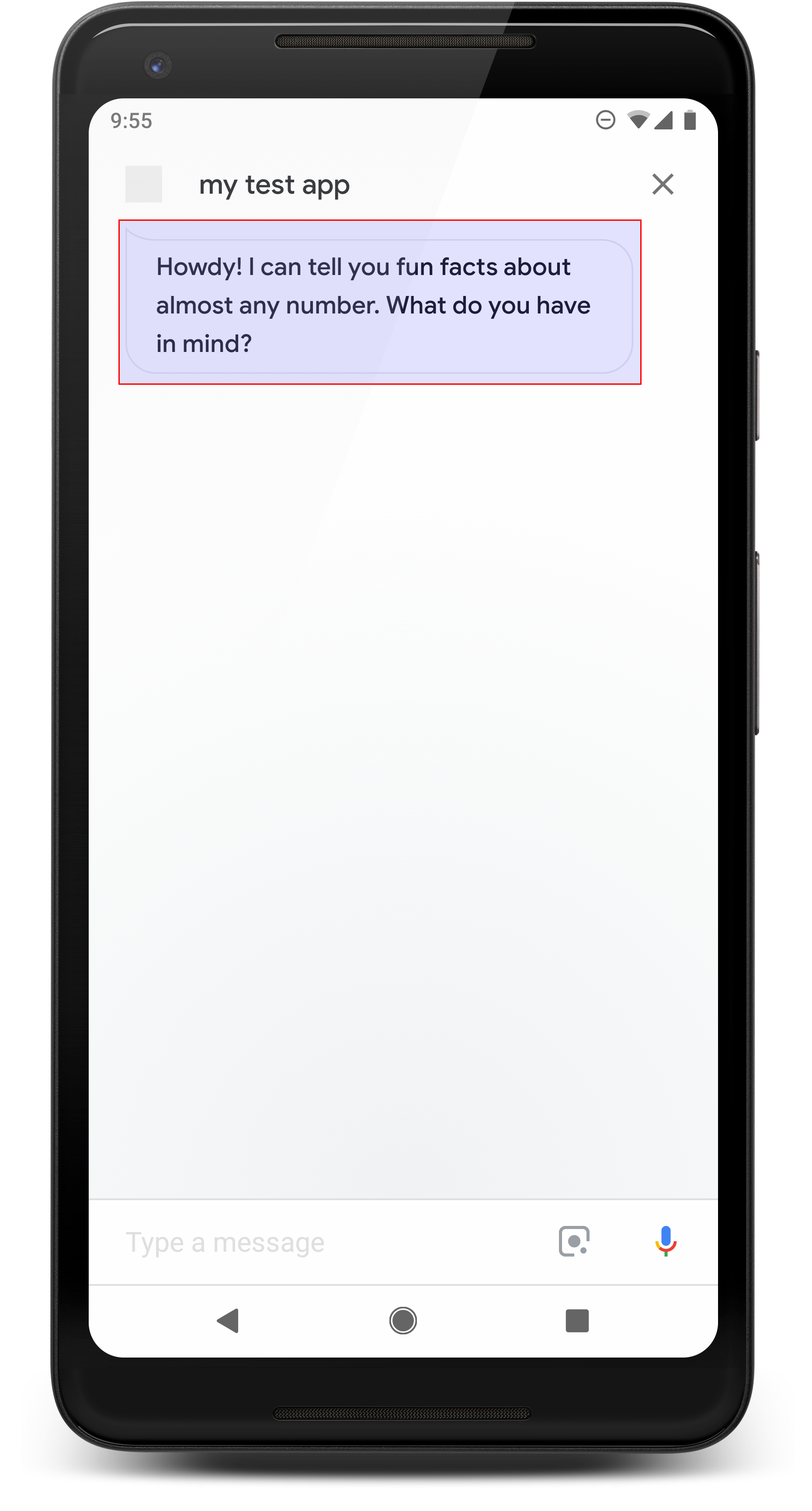
پاسخ های ساده دارای الزامات و ویژگی های اختیاری زیر هستند که می توانید آنها را پیکربندی کنید:
- روی سطوحی با قابلیتهای
actions.capability.AUDIO_OUTPUT
یاactions.capability.SCREEN_OUTPUT
پشتیبانی میشود. محدودیت 640 نویسه در هر حباب چت. رشته های طولانی تر از حد مجاز در اولین شکست کلمه (یا فضای خالی) قبل از 640 کاراکتر کوتاه می شوند.
محتوای حباب چت باید زیر مجموعه آوایی یا رونوشت کامل خروجی TTS/SSML باشد. این به کاربران کمک می کند تا آنچه را که می گویید ترسیم کنند و درک مطلب را در شرایط مختلف افزایش می دهد.
حداکثر دو حباب چت در هر نوبت.
سر چت (لوگو) که به Google ارسال می کنید باید 192x192 پیکسل باشد و قابل انیمیشن نباشد.
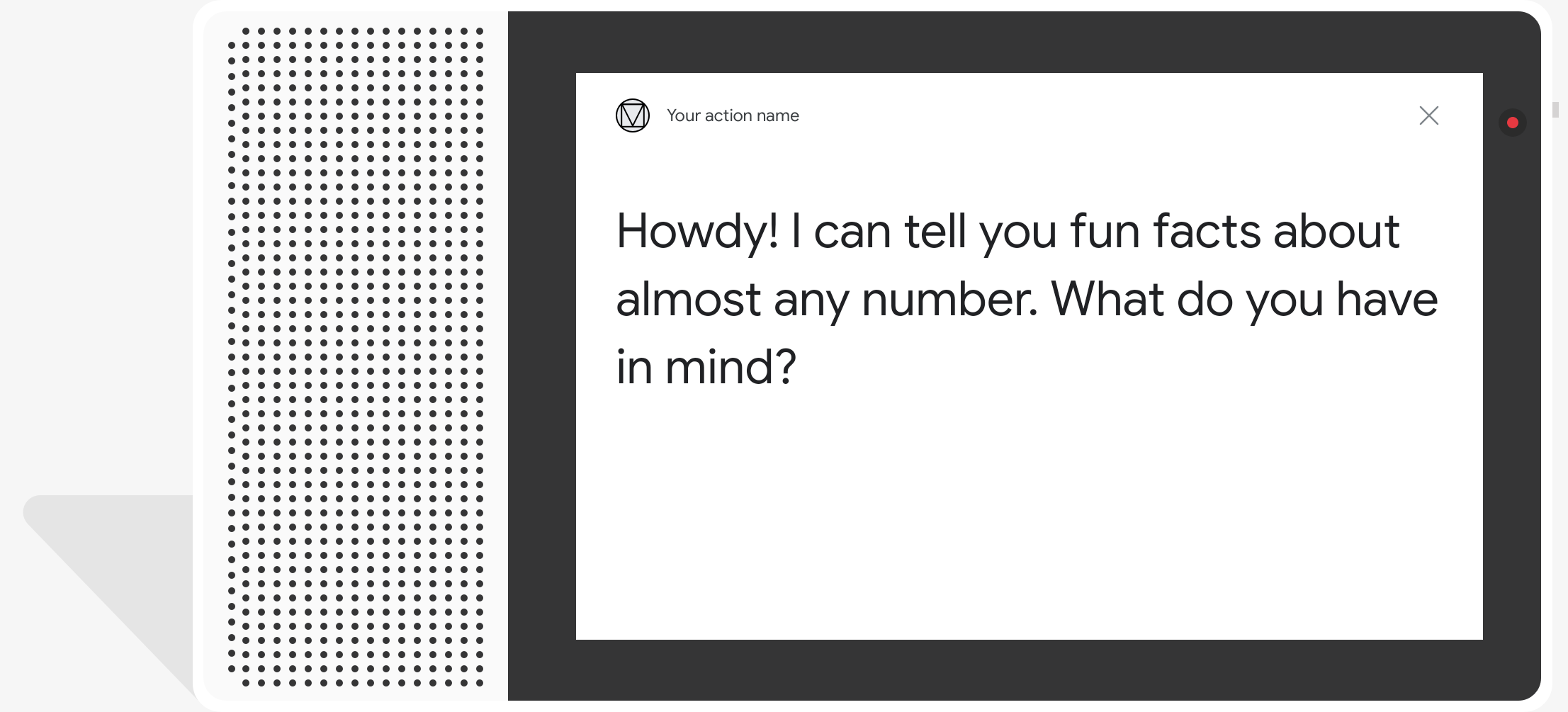
کد نمونه
Node.js
app.intent('Simple Response', (conv) => { conv.ask(new SimpleResponse({ speech: `Here's an example of a simple response. ` + `Which type of response would you like to see next?`, text: `Here's a simple response. ` + `Which response would you like to see next?`, })); });
جاوا
@ForIntent("Simple Response") public ActionResponse welcome(ActionRequest request) { ResponseBuilder responseBuilder = getResponseBuilder(request); responseBuilder.add( new SimpleResponse() .setTextToSpeech( "Here's an example of a simple response. " + "Which type of response would you like to see next?") .setDisplayText( "Here's a simple response. Which response would you like to see next?")); return responseBuilder.build(); }
Node.js
conv.ask(new SimpleResponse({ speech: `Here's an example of a simple response. ` + `Which type of response would you like to see next?`, text: `Here's a simple response. ` + `Which response would you like to see next?`, }));
جاوا
ResponseBuilder responseBuilder = getResponseBuilder(request); responseBuilder.add( new SimpleResponse() .setTextToSpeech( "Here's an example of a simple response. " + "Which type of response would you like to see next?") .setDisplayText( "Here's a simple response. Which response would you like to see next?")); return responseBuilder.build();
JSON
توجه داشته باشید که JSON زیر یک پاسخ وب هوک را توضیح می دهد.
{ "payload": { "google": { "expectUserResponse": true, "richResponse": { "items": [ { "simpleResponse": { "textToSpeech": "Here's an example of a simple response. Which type of response would you like to see next?", "displayText": "Here's a simple response. Which response would you like to see next?" } } ] } } } }
JSON
توجه داشته باشید که JSON زیر یک پاسخ وب هوک را توضیح می دهد.
{ "expectUserResponse": true, "expectedInputs": [ { "possibleIntents": [ { "intent": "actions.intent.TEXT" } ], "inputPrompt": { "richInitialPrompt": { "items": [ { "simpleResponse": { "textToSpeech": "Here's an example of a simple response. Which type of response would you like to see next?", "displayText": "Here's a simple response. Which response would you like to see next?" } } ] } } } ] }
SSML و صداها
استفاده از SSML و صداها در پاسخهای شما به آنها جلا بیشتری میدهد و تجربه کاربر را افزایش میدهد. قطعه کد زیر به شما نشان می دهد که چگونه پاسخی ایجاد کنید که از SSML استفاده می کند:
Node.js
app.intent('SSML', (conv) => { conv.ask(`<speak>` + `Here are <say-as interpet-as="characters">SSML</say-as> examples.` + `Here is a buzzing fly ` + `<audio src="https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg"></audio>` + `and here's a short pause <break time="800ms"/>` + `</speak>`); conv.ask('Which response would you like to see next?'); });
جاوا
@ForIntent("SSML") public ActionResponse ssml(ActionRequest request) { ResponseBuilder responseBuilder = getResponseBuilder(request); responseBuilder.add( "<speak>" + "Here are <say-as interpet-as=\"characters\">SSML</say-as> examples." + "Here is a buzzing fly " + "<audio src=\"https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg\"></audio>" + "and here's a short pause <break time=\"800ms\"/>" + "</speak>"); return responseBuilder.build(); }
Node.js
conv.ask(`<speak>` + `Here are <say-as interpet-as="characters">SSML</say-as> examples.` + `Here is a buzzing fly ` + `<audio src="https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg"></audio>` + `and here's a short pause <break time="800ms"/>` + `</speak>`); conv.ask('Which response would you like to see next?');
جاوا
ResponseBuilder responseBuilder = getResponseBuilder(request); responseBuilder.add( "<speak>" + "Here are <say-as interpet-as=\"characters\">SSML</say-as> examples." + "Here is a buzzing fly " + "<audio src=\"https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg\"></audio>" + "and here's a short pause <break time=\"800ms\"/>" + "</speak>"); return responseBuilder.build();
JSON
توجه داشته باشید که JSON زیر یک پاسخ وب هوک را توضیح می دهد.
{ "payload": { "google": { "expectUserResponse": true, "richResponse": { "items": [ { "simpleResponse": { "textToSpeech": "<speak>Here are <say-as interpet-as=\"characters\">SSML</say-as> examples.Here is a buzzing fly <audio src=\"https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg\"></audio>and here's a short pause <break time=\"800ms\"/></speak>" } }, { "simpleResponse": { "textToSpeech": "Which response would you like to see next?" } } ] } } } }
JSON
توجه داشته باشید که JSON زیر یک پاسخ وب هوک را توضیح می دهد.
{ "expectUserResponse": true, "expectedInputs": [ { "possibleIntents": [ { "intent": "actions.intent.TEXT" } ], "inputPrompt": { "richInitialPrompt": { "items": [ { "simpleResponse": { "textToSpeech": "<speak>Here are <say-as interpet-as=\"characters\">SSML</say-as> examples.Here is a buzzing fly <audio src=\"https://actions.google.com/sounds/v1/animals/buzzing_fly.ogg\"></audio>and here's a short pause <break time=\"800ms\"/></speak>" } }, { "simpleResponse": { "textToSpeech": "Which response would you like to see next?" } } ] } } } ] }
برای اطلاعات بیشتر به مستندات مرجع SSML مراجعه کنید.
کتابخانه صدا
ما انواع صداهای رایگان و کوتاه را در کتابخانه صوتی خود ارائه می دهیم. این صداها برای شما میزبانی می شوند، بنابراین تنها کاری که باید انجام دهید این است که آنها را در SSML خود بگنجانید.