This page explains implementation details for Topics API callers to observe and access topics. Before you start implementing your solution, make sure that your browser is set up correctly. Check the overview section to learn more about how callers observe and access users' topics.
Observe and access Topics
There are two ways to observe and access a user's topics: HTTP headers and JavaScript API.
HTTP headers
HTTP Headers is a recommended approach for observing and accessing user topics. Using this approach can be much more performant than using the JavaScript API. When using HTTP headers, the URL of the request provides the registrable domain that is recorded as the caller domain. This is the domain seen to have observed the user's topics.
Initiate Request
There are two ways to use Topics with headers:
- By accessing request and response headers on a
fetch()
request that includes abrowsingTopics: true
option. - By accessing headers for an iframe element that includes a
browsingtopics
attribute.
Initiate request with a fetch
Using fetch, the API caller makes a request that includes {browsingTopics: true}
in the options parameter. The origin of the fetch request's URL parameter is the origin seen to have observed topics.
fetch('<topics_caller_eTLD+1>', { browsingTopics: true })
.then((response) => {
// Process the response
});
Initiate request with an iframe
Add the browsingtopics
attribute to the <iframe>
element. The browser will include the Sec-Browsing-Topics
header in the iframe's request, with the iframe's origin as the caller.
<iframe src="https://adtech.example" browsingtopics></iframe>
Interpret request header values
For both approaches (fetch and iframe) topics observed for a user can be retrieved
on the server from the Sec-Browsing-Topics
request header. The Topics API will
include user topics in the header automatically on fetch()
or iframe request.
If the API returns one or more topics, a fetch request to the origin from which
the topics were observed will include a Sec-Browsing-Topics
header like this:
(325);v=chrome.1:1:1, ();p=P000000000
If no topics are returned by the API, the header looks like this:
();p=P0000000000000000000000000000000
Redirects will be followed, and the topics sent in the redirect request will be specific to the redirect URL.
Sec-Browsing-Topics
header values are padded, to mitigate the risk of an attacker learning the number of topics scoped to a caller based on the header length.
Handle server-side response
If the response to the request includes an Observe-Browsing-Topics: ?1
header, this signals that the browser should mark the topics from the accompanying request as observed, and include the current page visit in the user's next epoch topic calculation.
Include the Observe-Browsing-Topics: ?1
header in the response in your server-side code:
res.setHeader('Observe-Browsing-Topics', '?1');
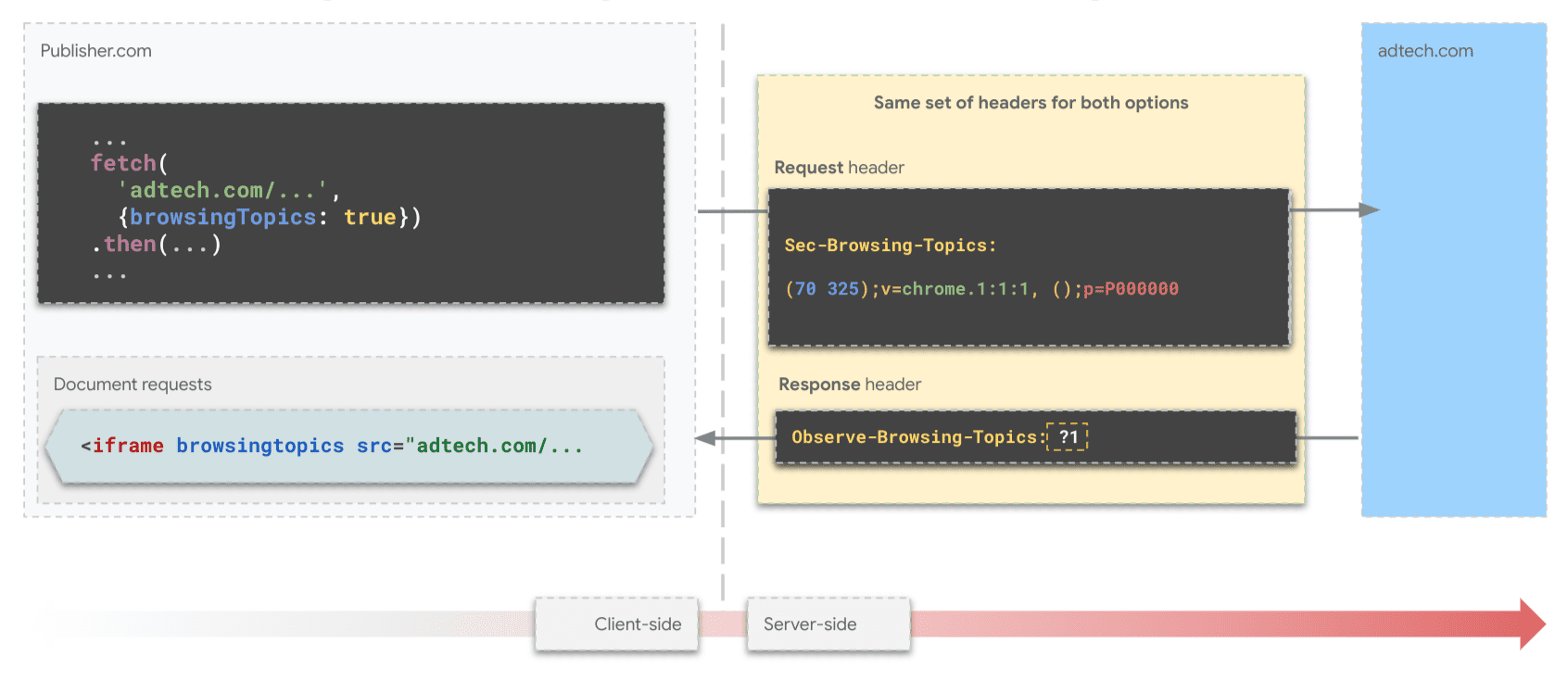
fetch()
.Share observed topics with partners
Since SSPs have presence only on the publisher's side, DSPs might want to share topics they observe on the advertiser's sites with their partner SSPs. They can do so by making a fetch()
request with the topics header to the SSPs from the advertiser's top-level context.
const response = await fetch("partner-ssp.example", {
browsingTopics: true
});
Observe and access Topics with JavaScript
The Topics JavaScript API method document.browsingTopics()
provides a way to both observe and retrieve a user's topics of interest within the browser environment:
- Record Observation: Informs the browser that the caller has observed the user visiting the current page. This observation contributes to the user's topic calculation for the caller in future epochs.
- Access Topics: Retrieves topics that the caller has previously observed for the user. The method returns an array of up to three topic objects, one for each of the most recent epochs, in random order.
We recommend you fork either the Topics JavaScript API demo and use it as a starting point for your code.
API availability
Before using the API, make sure it's supported and available:
if ('browsingTopics' in document && document.featurePolicy.allowsFeature('browsing-topics')) {
console.log('document.browsingTopics() is supported on this page');
} else {
console.log('document.browsingTopics() is not supported on this page');
}
Embed an iframe
A cross-origin iframe must be used for the call, because the context from which the API is invoked is used to ensure the browser returns the topics appropriate to the caller. Include an <iframe>
element in your HTML:
<iframe src="https://example.com" browsingtopics></iframe>
You can also create an iframe dynamically using JavaScript:
const iframe = document.createElement('iframe');
iframe.setAttribute('src', 'https://adtech.example/');
document.body.appendChild(iframe);
Call the API from within the iframe
try {
// Get the array of top topics for this user.
const topics = await document.browsingTopics();
// Request an ad creative, providing topics information.
const response = await fetch('https://ads.example/get-creative', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(topics)
})
// Get the JSON from the response.
const creative = await response.json();
// Display ad.
} catch (error) {
// Handle error.
}
By default, the document.browsingTopics()
method also causes the browser to record the current page visit as observed by the caller, so it can later be used in topics calculation. The method can be passed an optional argument to skip the page visit from being recorded: {skipObservation:true}
.
// current page won't be included in the calculation of topics:
const topics = await document.browsingTopics({skipObservation:true});
Understand response
A maximum of three topics is returned: one or zero for each of the last three weeks, depending on whether topics were observed or not. Only topics observed by the caller for the current user are returned. Here's an example of what the API returns:
[{
'configVersion': chrome.2,
'modelVersion': 4,
'taxonomyVersion': 4,
'topic': 309,
'version': chrome.2:2:4
}]
- configVersion: a string identifying the browser's topics algorithm configuration version.
- modelVersion: a string identifying the machine-learning classifier used to infer topics.
- taxonomyVersion: a string identifying the set of topics in use by the browser.
- topic: a number identifying the topic in the taxonomy.
- version: a string concatenating
configVersion
,taxonomyVersion
, andmodelVersion
. The parameters described in this guide, and details of the API (such as taxonomy size, the number of topics calculated per week and the number of topics returned per call) are subject to change as we incorporate ecosystem feedback and iterate on the API.
See the Test & go live page to learn which response to expect and how to use Topics as an additional signal for more relevant ads.
Next steps
Test & go live
Tooling
See also
Check out our resources to better understand the Topics API on the Web.
- Check out Topics demos, collab and walkthrough videos.
- See the list of Chrome flags that allow developers to customize the Topics API for testing.
- See how users and developers can control the API.
- Check out the resources for technical explainers and support. Ask questions, engage and share feedback.