概览
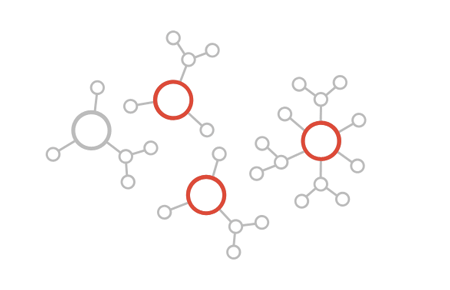
Freebase Search API 允许通过自由文本查询访问 Freebase 数据。查询结果进行排序,并具有数值相关性得分。
开发者可以应用过滤条件,将搜索结果限制为特定类型的数据。如需详细了解如何构造详细的搜索查询,请参阅搜索实战宝典。
开发者可以使用 Search API 的部分示例如下:
- 自动建议实体(例如 Freebase Suggestion 微件)
- 获取具有指定名称且最著名的实体的排名列表。
- 使用 Search Metaschema 查找实体。
以下代码示例支持多种语言,展示了如何搜索与文本“Cee Lo Green”相符的音乐人。另一个限制是他们创建了一个名为“The Lady Killer”的内容。
Python
import json import urllib api_key = open(".api_key").read() query = 'blue bottle' service_url = 'https://www.googleapis.com/freebase/v1/search' params = { 'query': query, 'key': api_key } url = service_url + '?' + urllib.urlencode(params) response = json.loads(urllib.urlopen(url).read()) for result in response['result']: print(result['name'] + ' (' + str(result['score']) + ')')
Ruby
require 'rubygems' require 'cgi' require 'httparty' require 'json' require 'addressable/uri' API_KEY = open(".freebase_api_key").read() url = Addressable::URI.parse('https://www.googleapis.com/freebase/v1/search') url.query_values = { 'query' => 'Blue Bottle', 'key'=> API_KEY } response = HTTParty.get(url, :format => :json) response['result'].each { |topic| puts topic['name'] }此示例使用 Httparty 和 Addressable 库。
Java
package com.freebase.samples; import com.google.api.client.http.GenericUrl; import com.google.api.client.http.HttpRequest; import com.google.api.client.http.HttpRequestFactory; import com.google.api.client.http.HttpResponse; import com.google.api.client.http.HttpTransport; import com.google.api.client.http.javanet.NetHttpTransport; import com.jayway.jsonpath.JsonPath; import java.io.FileInputStream; import java.util.Properties; import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.parser.JSONParser; public class SearchExample { public static Properties properties = new Properties(); public static void main(String[] args) { try { properties.load(new FileInputStream("freebase.properties")); HttpTransport httpTransport = new NetHttpTransport(); HttpRequestFactory requestFactory = httpTransport.createRequestFactory(); JSONParser parser = new JSONParser(); GenericUrl url = new GenericUrl("https://www.googleapis.com/freebase/v1/search"); url.put("query", "Cee Lo Green"); url.put("filter", "(all type:/music/artist created:\"The Lady Killer\")"); url.put("limit", "10"); url.put("indent", "true"); url.put("key", properties.get("API_KEY")); HttpRequest request = requestFactory.buildGetRequest(url); HttpResponse httpResponse = request.execute(); JSONObject response = (JSONObject)parser.parse(httpResponse.parseAsString()); JSONArray results = (JSONArray)response.get("result"); for (Object result : results) { System.out.println(JsonPath.read(result,"$.name").toString()); } } catch (Exception ex) { ex.printStackTrace(); } } }
JavaScript
<!DOCTYPE html> <html> <head> <script src="https://www.gstatic.com/external_hosted/jquery2.min.js"></script> </head> <body><aside class="warning"><strong>Warning: </strong>The Freebase API will be retired on June 30, 2015.</aside> <script> var service_url = 'https://www.googleapis.com/freebase/v1/search'; var params = { 'query': 'Cee Lo Green', 'filter': '(all type:/music/artist created:"The Lady Killer")', 'limit': 10, 'indent': true }; $.getJSON(service_url + '?callback=?', params).done(function(response) { $.each(response.result, function(i, result) { $('<div>', {text:result['name']}).appendTo(document.body); }); }); </script> </body> </html>此示例使用 jQuery 库。
PHP
<!DOCTYPE html> <html> <body> <?php include('.freebase-api-key'); $service_url = 'https://www.googleapis.com/freebase/v1/search'; $params = array( 'query' => 'Blue Bottle', 'key' => $freebase_api_key ); $url = $service_url . '?' . http_build_query($params); $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $response = json_decode(curl_exec($ch), true); curl_close($ch); foreach($response['result'] as $result) { echo $result['name'] . '<br/>'; } ?> </body> </html>
另请参阅客户端库页面,查看是否支持您喜爱的语言。
Search API 文档
另请参阅以下文档:
- 如需详细了解如何使用该 API,请参阅搜索参考文档。
- 如需详细了解如何构造详细的搜索查询,请参阅搜索实战宝典。
- 请参阅搜索 Metaschema 文档,了解实体与属性之间的关系。
安全注意事项
Search API 会将 Freebase 图表中存储的用户生成的内容编入索引并进行搜索。也就是说,如果不先进行安全转义,就不能直接使用网页上的内容。
如需了解详情,请参阅使用入门:安全性。
高级过滤
Search API 支持大量过滤器限制条件,以让搜索更容易定位到正确的实体。
例如,使用“类型”过滤条件,我们可以显示 Freebase 中最值得关注的人员列表。
filter=(any type:/people/person)
过滤条件限制接受各种输入:
- 简单易懂的架构实体或用户的 ID,例如:
/people/person
(适用于类型约束)/film
(针对网域限制条件)
- Freebase MID,例如:
- 针对同一
/people/person
类型约束条件使用/m/01g317
- 针对上述
/film
网域限制的/m/010s
- 针对同一
- 实体名称,例如:
"person"
,适用于不太精确的/people/person
类型约束条件"film"
,表示/film
网域限制不精确
过滤器限制可以分为几个类别。如需了解详情,请参阅搜索实战宝典。
过滤器限制条件可以直接在 SearchRequest
中随意组合和重复使用。重复的过滤器约束参数将合并为 OR 查询。将多个过滤条件限制参数或组合并为 AND 查询。
例如:
要搜索名为“Gore”的人物或城市,请尝试以下操作:
query=gore &filter=(any type:/people/person type:/location/citytown)
您可以使用 filter 参数替换和更好地控制这种组合行为,该参数提供了更丰富的用于组合约束条件的接口。这是一个 s 表达式,可能是任意嵌套的,其中运算符是以下项之一:
any
,逻辑“或”all
,逻辑与not
should
,只能在顶级对象中使用,表示约束条件是可选的。在评分过程中,对于与可选限制条件不匹配的匹配项,系统会将其不匹配的每个可选限制条件的分数分成两半。
例如:
要匹配 /people/person
类型或 /film
网域,请尝试以下操作:
query=gore &filter=(any type:/people/person domain:/film)