סמנים מתקדמים משתמשים בשתי מחלקות כדי להגדיר סמנים: המחלקה GMSAdvancedMarker
מספקת את יכולות ברירת המחדל של הסמנים, והמחלקה GMSPinImageOptions
מכילה אפשרויות להתאמה אישית נוספת. הדף הזה מראה איך להתאים אישית את הסמנים בדרכים הבאות:
- שינוי צבע הרקע
- שינוי של צבע הגבולות
- שינוי צבע הגליף
- שינוי הטקסט בגליף
- תמיכה בתצוגות מותאמות אישית ובאנימציה באמצעות המאפיין iconView
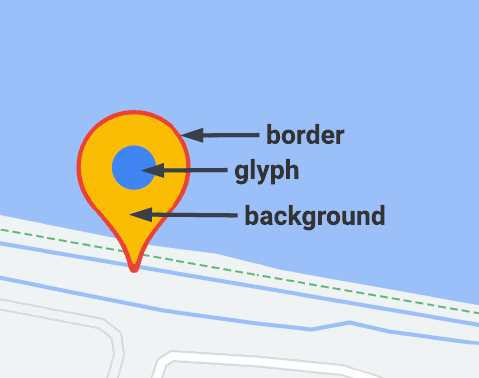
שינוי צבע הרקע
משתמשים באפשרות GMSPinImageOptions.backgroundColor
כדי לשנות את צבע הרקע של הסמן.
Swift
//... let options = GMSPinImageOptions() options.backgroundColor = .blue let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.backgroundColor = [UIColor blueColor]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; customTextMarker.icon = pin; customTextMarker.map = mapView;
שינוי של צבע הגבולות
משתמשים באפשרות GMSPinImageOptions.borderColor
כדי לשנות את צבע הרקע של הסמן.
Swift
//... let options = GMSPinImageOptions() options.borderColor = .blue let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.backgroundColor = [UIColor blueColor]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; advancedMarker.icon = pin; advancedMarker.map = mapView;
שינוי צבע הגליף
משתמשים ב-GMSPinImageGlyph.glyphColor
כדי לשנות את צבע הרקע של הסמן.
Swift
//... let options = GMSPinImageOptions() let glyph = GMSPinImageGlyph(glyphColor: .yellow) options.glyph = glyph let glyphColor = GMSPinImage(options: options) advancedMarker.icon = glyphColor advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.glyph = [[GMSPinImageGlyph alloc] initWithGlyphColor:[UIColor yellowColor]]; GMSPinImage *glyphColor = [GMSPinImage pinImageWithOptions:options]; advancedMarker.icon = glyphColor; advancedMarker.map = mapView;
שינוי הטקסט בגליף
כדי לשנות את טקסט הגליף של הסמן, משתמשים ב-GMSPinImageGlyph
.
Swift
//... let options = GMSPinImageOptions() let glyph = GMSPinImageGlyph(text: "ABC", textColor: .white) options.glyph = glyph let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.glyph = [[GMSPinImageGlyph alloc] initWithText:@"ABC" textColor:[UIColor whiteColor]]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; customTextMarker.icon = pin; customTextMarker.map = mapView;
תמיכה בתצוגות בהתאמה אישית ובאנימציה באמצעות המאפיין iconView
בדומה ל-GMSMarker
, GMSAdvancedMarker
תומך גם בסמנים עם iconView
.
המאפיין iconView
תומך באנימציה של כל המאפיינים מונפשים של UIView
, מלבד מסגרת ומרכז. הוא לא תומך בסמנים שבהם מוצגים iconViews
ו-icons
באותה מפה.
Swift
//... let advancedMarker = GMSAdvancedMarker(position: coordinate) advancedMarker.iconView = customView() advancedMarker.map = mapView func customView() -> UIView { // return your custom UIView. }
Objective-C
//... GMSAdvancedMarker *advancedMarker = [GMSAdvancedMarker markerWithPosition:kCoordinate]; advancedMarker.iconView = [self customView]; advancedMarker.map = self.mapView; - (UIView *)customView { // return custom view }
אילוצי פריסה
GMSAdvancedMarker
לא תומך ישירות במגבלות פריסה של iconView
. אבל אפשר להגדיר אילוצי פריסה לרכיבי ממשק משתמש בתוך iconView
. בזמן יצירת התצוגה, צריך להעביר את האובייקט frame
או size
לסמן.
Swift
//do not set advancedMarker.iconView.translatesAutoresizingMaskIntoConstraints = false let advancedMarker = GMSAdvancedMarker(position: coordinate) let customView = customView() //set frame customView.frame = CGRect(0, 0, width, height) advancedMarker.iconView = customView
Objective-C
//do not set advancedMarker.iconView.translatesAutoresizingMaskIntoConstraints = NO; GMSAdvancedMarker *advancedMarker = [GMSAdvancedMarker markerWithPosition:kCoordinate]; CustomView *customView = [self customView]; //set frame customView.frame = CGRectMake(0, 0, width, height); advancedMarker.iconView = customView;