高级标记使用两个类来定义标记:GMSAdvancedMarker
类提供默认标记功能,GMSPinImageOptions
类包含进一步自定义的选项。本页面介绍了如何通过以下方式自定义标记:
- 更改背景颜色
- 更改边框颜色
- 更改字形颜色
- 更改字形文本
- 使用 iconView 属性支持自定义视图和动画
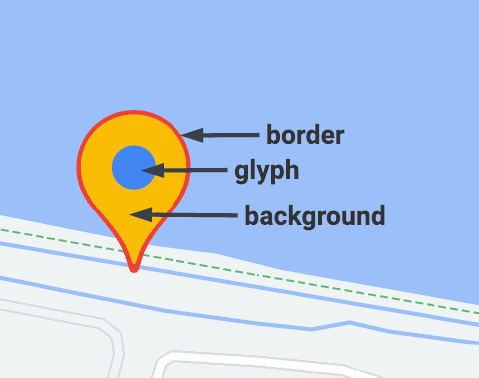
更改背景颜色
使用 GMSPinImageOptions.backgroundColor
选项可更改标记的背景颜色。
Swift
//... let options = GMSPinImageOptions() options.backgroundColor = .blue let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.backgroundColor = [UIColor blueColor]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; customTextMarker.icon = pin; customTextMarker.map = mapView;
更改边框颜色
使用 GMSPinImageOptions.borderColor
选项可更改标记的背景颜色。
Swift
//... let options = GMSPinImageOptions() options.borderColor = .blue let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.backgroundColor = [UIColor blueColor]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; advancedMarker.icon = pin; advancedMarker.map = mapView;
更改字形颜色
使用 GMSPinImageGlyph.glyphColor
可更改标记的背景颜色。
Swift
//... let options = GMSPinImageOptions() let glyph = GMSPinImageGlyph(glyphColor: .yellow) options.glyph = glyph let glyphColor = GMSPinImage(options: options) advancedMarker.icon = glyphColor advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.glyph = [[GMSPinImageGlyph alloc] initWithGlyphColor:[UIColor yellowColor]]; GMSPinImage *glyphColor = [GMSPinImage pinImageWithOptions:options]; advancedMarker.icon = glyphColor; advancedMarker.map = mapView;
更改字形文本
使用 GMSPinImageGlyph
可更改标记的字形文本。
Swift
//... let options = GMSPinImageOptions() let glyph = GMSPinImageGlyph(text: "ABC", textColor: .white) options.glyph = glyph let pinImage = GMSPinImage(options: options) advancedMarker.icon = pinImage advancedMarker.map = mapView
Objective-C
//... GMSPinImageOptions *options = [[GMSPinImageOptions alloc] init]; options.glyph = [[GMSPinImageGlyph alloc] initWithText:@"ABC" textColor:[UIColor whiteColor]]; GMSPinImage *pin = [GMSPinImage pinImageWithOptions:options]; customTextMarker.icon = pin; customTextMarker.map = mapView;
支持使用 iconView
属性的自定义视图和动画
与 GMSMarker
类似,GMSAdvancedMarker
也支持带有 iconView
的地图标记。iconView
属性支持具有 UIView
所有可设置动画的属性(除了 frame 和 center 以外)的动画。不支持在同一地图上显示 iconViews
和 icons
标记。
Swift
//... let advancedMarker = GMSAdvancedMarker(position: coordinate) advancedMarker.iconView = customView() advancedMarker.map = mapView func customView() -> UIView { // return your custom UIView. }
Objective-C
//... GMSAdvancedMarker *advancedMarker = [GMSAdvancedMarker markerWithPosition:kCoordinate]; advancedMarker.iconView = [self customView]; advancedMarker.map = self.mapView; - (UIView *)customView { // return custom view }
布局约束条件
GMSAdvancedMarker
不直接支持其 iconView
的布局约束条件。不过,您可以在 iconView
中为界面元素设置布局约束条件。创建视图后,应将对象 frame
或 size
传递给标记。
Swift
//do not set advancedMarker.iconView.translatesAutoresizingMaskIntoConstraints = false let advancedMarker = GMSAdvancedMarker(position: coordinate) let customView = customView() //set frame customView.frame = CGRect(0, 0, width, height) advancedMarker.iconView = customView
Objective-C
//do not set advancedMarker.iconView.translatesAutoresizingMaskIntoConstraints = NO; GMSAdvancedMarker *advancedMarker = [GMSAdvancedMarker markerWithPosition:kCoordinate]; CustomView *customView = [self customView]; //set frame customView.frame = CGRectMake(0, 0, width, height); advancedMarker.iconView = customView;