Cada solicitud que envía tu aplicación a las APIs de Perfil de Negocio debe incluir un token de autorización. El token de autorización identifica al usuario o la aplicación para Google, lo que permite el acceso a las APIs de Perfil de Negocio. Tu aplicación debe usar el protocolo OAuth 2.0 para autorizar solicitudes.
En esta guía, se explican los diferentes métodos que puedes usar para implementar OAuth 2.0 en tu plataforma. Google Identity Platform proporciona la funcionalidad de Acceso con Google y OAuth que se usa en esta guía.
Para comprender mejor cómo usar OAuth 2.0 para aplicaciones de servidor web, consulta la guía aquí.
La implementación de OAuth 2.0 proporciona los siguientes beneficios:
- Protege el acceso a los datos del propietario de la empresa.
- Establece la identidad del propietario de la empresa cuando accede a su Cuenta de Google.
- Establece que una plataforma o aplicación de socios puede acceder a los datos de ubicación y modificarlos con el consentimiento explícito del propietario de la empresa. El propietario puede revocar este acceso más adelante.
- Establece la identidad de la plataforma del socio.
- Permite que las plataformas asociadas realicen acciones en línea o sin conexión en nombre del propietario de la empresa. Esto incluye las respuestas a las opiniones, la creación de publicaciones y las actualizaciones de los elementos del menú.
Acceso a la API con OAuth 2.0
Antes de comenzar, debes configurar tu proyecto de Google Cloud y habilitar las APIs de Business Profile. Para obtener más información, consulta la documentación sobre la configuración básica.
Configura OAuth y la pantalla de consentimiento
Sigue estos pasos para crear credenciales y la pantalla de consentimiento:
- En la página Credenciales de la consola de la API, haz clic en Crear credenciales y selecciona "ID de cliente de OAuth" en la lista desplegable.
- Selecciona tu tipo de aplicación, completa la información relevante y haz clic en Crear.
- Haz clic en Guardar.
- Actualiza la configuración de la pantalla de consentimiento de OAuth. Desde allí, puedes actualizar el nombre y el logotipo de la aplicación, así como incluir un vínculo a tus condiciones del servicio y política de privacidad.
En la siguiente imagen, se muestran los campos de nombre y logotipo de la aplicación en la pantalla de consentimiento de OAuth:
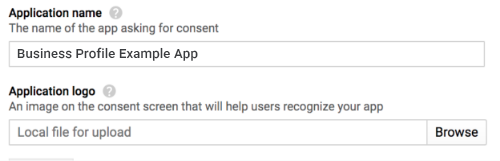
En la siguiente imagen, se muestran los campos adicionales que aparecen en la pantalla de consentimiento de OAuth:
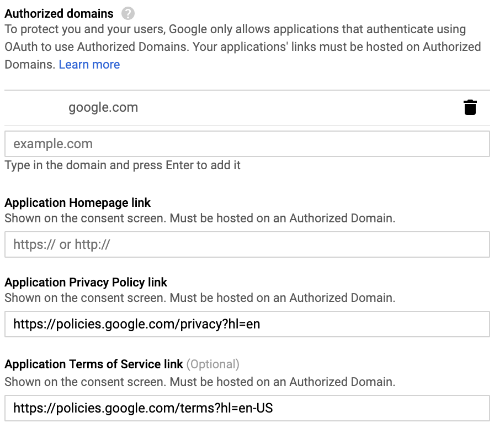
La siguiente imagen es un ejemplo de lo que el usuario podría ver antes de dar su consentimiento:
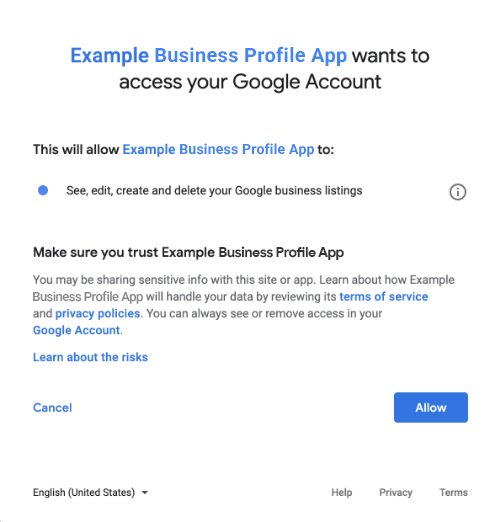
Métodos para incorporar OAuth 2.0
Puedes usar los siguientes métodos para implementar OAuth 2.0:
En el siguiente contenido, se proporciona información sobre estos métodos para incorporar OAuth 2.0 en tu aplicación.
Alcances de la autorización
Se necesita uno de los siguientes permisos de OAuth:
- https://www.googleapis.com/auth/business.manage
- https://www.googleapis.com/auth/plus.business.manage
El permiso plus.business.manage dejó de estar disponible y está disponible para mantener la retrocompatibilidad con las implementaciones existentes.
Bibliotecas cliente
En los ejemplos específicos de cada lenguaje de esta página, se usan bibliotecas cliente de la API de Google para implementar la autorización de OAuth 2.0. Para ejecutar las muestras de código, primero debes instalar la biblioteca cliente de tu lenguaje.
Las bibliotecas cliente están disponibles para los siguientes idiomas:
Acceso con Google
Acceso con Google es la forma más rápida de integrar OAuth en tu plataforma. Está disponible para Android, iOS, la Web y mucho más.
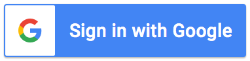
El Acceso con Google es un sistema de autenticación seguro que permite a los usuarios acceder con su Cuenta de Google, que es la misma que usan para acceder a otros servicios de Google. Una vez que el usuario haya accedido, podrá otorgar consentimiento para que tu aplicación llame a las APIs de Perfil de Negocio y cambie el código de autorización que se usa para obtener tokens de actualización y acceso.
Acceso sin conexión
Es posible que desees llamar a las APIs de Perfil de Negocio en nombre de un usuario, incluso cuando este no tenga conexión. Se recomienda que las plataformas incorporen esta funcionalidad, ya que puedes editar, ver y administrar las fichas en cualquier momento después de que el usuario haya accedido y otorgado su consentimiento.
Google supone que el usuario ya accedió con su Cuenta de Google, que otorgó consentimiento para que tu aplicación llame a las APIs de Perfil de Negocio y que intercambió un código de autorización que luego se usa para obtener un token de actualización y, más adelante, un token de acceso. El usuario puede almacenar el token de actualización de forma segura y usarlo más adelante para obtener un token de acceso nuevo en cualquier momento. Para obtener más información, lee Acceso con Google para apps del servidor.
En el siguiente fragmento de código, se muestra cómo implementar el acceso sin conexión en tu aplicación. Para ejecutar este código, consulta Cómo ejecutar la muestra.
<!-- The top of file index.html --> <html itemscope itemtype="http://schema.org/Article"> <head> <!-- BEGIN Pre-requisites --> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.2/jquery.min.js"> </script> <script src="https://apis.google.com/js/client:platform.js?onload=start" async defer> </script> <!-- END Pre-requisites --> <!-- Continuing the <head> section --> <script> function start() { gapi.load('auth2', function() { auth2 = gapi.auth2.init({ client_id: 'YOUR_CLIENT_ID.apps.googleusercontent.com', // Scopes to request in addition to 'profile' and 'email' scope: 'https://www.googleapis.com/auth/business.manage', immediate: true }); }); } </script> </head> <body> <!-- Add where you want your sign-in button to render --> <!-- Use an image that follows the branding guidelines in a real app, more info here: https://developers.google.com/identity/branding-guidelines --> <h1>Business Profile Offline Access Demo</h1> <p> This demo demonstrates the use of Google Identity Services and OAuth to gain authorization to call the Business Profile APIs on behalf of the user, even when the user is offline. When a refresh token is acquired, store this token securely on your database. You will then be able to use this token to refresh the OAuth credentials and make offline API calls on behalf of the user. The user may revoke access at any time from the <a href='https://myaccount.google.com/permissions'>Account Settings</a> page. </p> <button id="signinButton">Sign in with Google</button><br> <input id="authorizationCode" type="text" placeholder="Authorization Code" style="width: 60%"><button id="accessTokenButton" disabled>Retrieve Access/Refresh Token</button><br> <input id="refreshToken" type="text" placeholder="Refresh Token, never expires unless revoked" style="width: 60%"><button id="refreshSubmit">Refresh Access Token</button><br> <input id="accessToken" type="text" placeholder="Access Token" style="width: 60%"><button id="gmbAccounts">Get Business Profile Accounts</button><br> <p>API Responses:</p> <script> //Will be populated after sign in. var authCode; $('#signinButton').click(function() { // signInCallback auth2.grantOfflineAccess().then(signInCallback); }); $('#accessTokenButton').click(function() { // signInCallback defined in step 6. retrieveAccessTokenAndRefreshToken(authCode); }); $('#refreshSubmit').click(function() { // signInCallback defined in step 6. retrieveAccessTokenFromRefreshToken($('#refreshToken').val()); }); $('#gmbAccounts').click(function() { // signInCallback defined in step 6. retrieveGoogleMyBusinessAccounts($('#accessToken').val()); }); function signInCallback(authResult) { //the 'code' field from the response, used to retrieve access token and bearer token if (authResult['code']) { // Hide the sign-in button now that the user is authorized, for example: $('#signinButton').attr('style', 'display: none'); authCode = authResult['code']; $("#accessTokenButton").attr( "disabled", false ); //Pretty print response var e = document.createElement("pre") e.innerHTML = JSON.stringify(authResult, undefined, 2); document.body.appendChild(e); //autofill authorization code input $('#authorizationCode').val(authResult['code']) } else { // There was an error. } } //WARNING: THIS FUNCTION IS DISPLAYED FOR DEMONSTRATION PURPOSES ONLY. YOUR CLIENT_SECRET SHOULD NEVER BE EXPOSED ON THE CLIENT SIDE!!!! function retrieveAccessTokenAndRefreshToken(code) { $.post('https://www.googleapis.com/oauth2/v4/token', { //the headers passed in the request 'code' : code, 'client_id' : 'YOUR_CLIENT_ID.apps.googleusercontent.com', 'client_secret' : 'YOUR_CLIENT_SECRET', 'redirect_uri' : 'http://localhost:8000', 'grant_type' : 'authorization_code' }, function(returnedData) { console.log(returnedData); //pretty print JSON response var e = document.createElement("pre") e.innerHTML = JSON.stringify(returnedData, undefined, 2); document.body.appendChild(e); $('#refreshToken').val(returnedData['refresh_token']) }); } //WARNING: THIS FUNCTION IS DISPLAYED FOR DEMONSTRATION PURPOSES ONLY. YOUR CLIENT_SECRET SHOULD NEVER BE EXPOSED ON THE CLIENT SIDE!!!! function retrieveAccessTokenFromRefreshToken(refreshToken) { $.post('https://www.googleapis.com/oauth2/v4/token', { // the headers passed in the request 'refresh_token' : refreshToken, 'client_id' : 'YOUR_CLIENT_ID.apps.googleusercontent.com', 'client_secret' : 'YOUR_CLIENT_SECRET', 'redirect_uri' : 'http://localhost:8000', 'grant_type' : 'refresh_token' }, function(returnedData) { var e = document.createElement("pre") e.innerHTML = JSON.stringify(returnedData, undefined, 2); document.body.appendChild(e); $('#accessToken').val(returnedData['access_token']) }); } function retrieveGoogleMyBusinessAccounts(accessToken) { $.ajax({ type: 'GET', url: 'https://mybusinessaccountmanagement.googleapis.com/v1/accounts', headers: { 'Authorization' : 'Bearer ' + accessToken }, success: function(returnedData) { var e = document.createElement("pre") e.innerHTML = JSON.stringify(returnedData, undefined, 2); document.body.appendChild(e); } }); } </script> </body> </html>
Solo acceso en línea
Para facilitar la implementación, las llamadas de las APIs de Perfil de Negocio se pueden realizar sin almacenar en caché los tokens de actualización del usuario. Sin embargo, el usuario debe haber accedido para que la plataforma realice llamadas a la API como el usuario.
En el siguiente fragmento de código, se muestra la implementación del flujo de Acceso con Google y cómo realizar una llamada a la API específica del usuario. Después de que el usuario accede con su Cuenta de Google y otorga su consentimiento a tu aplicación, se le otorga un token de acceso. Este token de acceso identifica al usuario y se debe pasar como un encabezado en la solicitud a las APIs de Perfil de Negocio.
Para ejecutar este código, consulta Cómo ejecutar la muestra.
<!-- The top of file index.html --> <html lang="en"> <head> <meta name="google-signin-scope" content="profile email https://www.googleapis.com/auth/business.manage"> <meta name="google-signin-client_id" content="YOUR_CLIENT_ID.apps.googleusercontent.com"> <script src="https://apis.google.com/js/platform.js" async defer></script> </head> <body> <div class="g-signin2" data-onsuccess="onSignIn" data-theme="dark"></div> <script> var gmb_api_version = 'https://mybusinessaccountmanagement.googleapis.com/v1'; function onSignIn(googleUser) { // Useful data for your client-side scripts: var profile = googleUser.getBasicProfile(); console.log('Full Name: ' + profile.getName()); console.log("Email: " + profile.getEmail()); var access_token = googleUser.getAuthResponse().access_token; //Using the sign in data to make a Business Profile APIs call var req = gmb_api_version + '/accounts'; var xhr = new XMLHttpRequest(); xhr.open('GET', req); xhr.setRequestHeader('Authorization', 'Bearer ' + access_token); //Displaying the API response xhr.onload = function () { document.body.appendChild(document.createTextNode(xhr.responseText)); } xhr.send(); } </script> </body> </html>
Ejecuta la muestra
Realiza los siguientes pasos para ejecutar el código de muestra proporcionado:
- Guarda el fragmento de código en un archivo titulado
index.html
. Asegúrate de haber configurado tu ID de cliente en el archivo. Inicia el servidor web con el siguiente comando desde tu directorio de trabajo:
python -m SimpleHTTPServer 8000
python -m http.server 8000
En la página Credenciales de la Consola de API, selecciona el ID de cliente que se usó.
En el campo Orígenes autorizados de JavaScript, ingresa la URL de tu sitio web. Para ejecutar el código de muestra de esta guía, también debes agregar
http://localhost:8000
.Carga la siguiente URL en tu navegador:
http://localhost:8000/index.html