将动态呈现作为临时解决方法
在某些网站上,在浏览器中执行 JavaScript 时,JavaScript 会在网页上生成其他内容。这称为客户端呈现。虽然 Google 搜索执行 JavaScript,但 Google 搜索中的 JavaScript 功能存在限制,因此某些网页的内容可能无法在所呈现的 HTML 中显示。其他搜索引擎可能会选择忽略 JavaScript 而看不到 JavaScript 生成的内容。
对于 JavaScript 生成内容不可供搜索引擎访问的网站,动态呈现是一种临时解决方法。动态呈现服务器会检测 JavaScript 生成内容时可能存在问题的漫游器,并向这些漫游器提供未启用 JavaScript 的服务器呈现版本,同时向用户显示客户端呈现的内容版本。
动态呈现是一种临时解决方法,而非建议的解决方案,因为它会带来额外的复杂性和资源要求。
应使用动态呈现的网站
动态呈现是一种临时解决方法,适用于由 JavaScript 生成、可编入索引且快速变化的公开内容,或使用您关注的抓取工具不支持的 JavaScript 功能的内容。并非所有网站都需要使用动态呈现,并且有比动态呈现更好的解决方案,如这篇关于在网页上呈现的文章中所述。
了解动态呈现的运作方式
要采用动态呈现,您的网络服务器必须能够检测抓取工具(例如,通过检查用户代理)。来自抓取工具的请求将路由到呈现器,来自用户的请求将以正常方式进行处理。如果需要,动态呈现器会提供适合抓取工具的内容版本,例如,它可能会提供静态 HTML 版本。您可以选择为所有网页启用动态呈现器,也可以逐个网页启用动态呈现器。
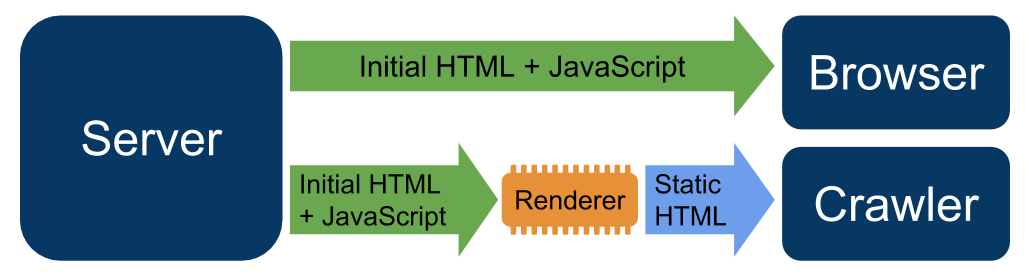
动态呈现不属于伪装真实内容
Googlebot 通常不会将动态呈现视为伪装真实内容。 只要您的动态呈现显示的是类似内容,Googlebot 便不会将动态呈现视为伪装真实内容。
设置动态呈现后,您的网站可能会显示错误页面。Googlebot 不会将这些错误页面视为伪装真实内容,也不会将错误视为任何其他错误页面。
使用动态呈现为用户和抓取工具提供完全不同的内容会被视为伪装真实内容。例如,某个网站向用户提供有关猫的页面,但向抓取工具提供有关狗的页面,则该网站会被视为伪装真实内容。
实现动态呈现
要为您的内容设置动态呈现,请遵循我们的通用指南。您需要参考具体的配置详情,因为不同实现方式的配置详情会有很大差别。
- 安装并配置动态渲染器(例如 Puppeteer、Rendertron 或 prerender.io),以便将内容转换为更便于抓取工具使用的静态 HTML。
- 选择您希望接收您的静态 HTML 的用户代理,并参考具体的配置详情,了解如何更新或添加用户代理。以下示例显示了 Rendertron 中间件中常见用户代理的列表:
export const botUserAgents = [ 'googlebot', 'bingbot', 'linkedinbot', 'mediapartners-google', ];
- 如果预呈现会拖慢服务器速度,或者预呈现请求非常多,请考虑为预呈现的内容实现缓存功能,或验证相应请求是否来自合法的抓取工具。
- 确定用户代理需要桌面版内容还是移动版内容。使用动态提供内容,以便根据情况提供桌面版本或移动版本。请参阅以下示例,了解配置如何确定用户代理需要桌面版内容还是移动版内容:
isPrerenderedUA = userAgent.matches(botUserAgents) isMobileUA = userAgent.matches(['mobile', 'android'])
if (!isPrerenderedUA) { } else { servePreRendered(isMobileUA) } - 将服务器配置为向您选择的抓取工具提供静态 HTML。您可以通过多种方法完成此项配置,具体取决于您的技术。以下是一些示例:
- 将来自抓取工具的请求重定向到动态呈现器。
- 在部署过程中进行预呈现,并使您的服务器向抓取工具提供静态 HTML 内容。
- 将动态呈现机制内置到自定义服务器代码中。
- 将来自预渲染服务的静态内容提供给抓取工具。
- 为服务器使用中间件(例如,rendertron 中间件)。
验证配置
实现动态渲染后,请对网址进行以下测试,验证一切是否如预期一样正常运作:
- 使用网址检查工具测试移动版和桌面版内容,确保在渲染的网页上也可以看到这些内容(渲染的网页是 Google 所看到的网页样貌)。
done 成功:桌面版和移动版内容与您期望用户看到的内容一致。
error 重试:如果您看到的内容与预期不符,请参阅“问题排查”部分。
- 如果您使用结构化数据,请使用富媒体搜索结果测试来测试结构化数据能否正确渲染。
done 成功:结构化数据与您预期的显示效果一致。
error 重试:如果结构化数据与您预期的显示效果不一致,请参阅“问题排查”部分。
问题排查
如果网址检查工具显示您的内容有错误,或者您的内容未显示在 Google 搜索结果中,请尝试解决最常见的问题。如果您仍遇到问题,请在 Google 搜索中心帮助社区内发新的主题帖提问。
内容不完整或看起来不同
error 导致问题的原因:您的渲染程序可能未正确配置,或者您的 Web 应用可能与您的渲染解决方案不兼容。有时,超时也可能会导致内容无法正确呈现。
done 解决问题:请参阅所用渲染解决方案的相关文档,调试您的动态渲染设置。
响应时间较长
error 导致问题的原因:使用无头浏览器按需渲染网页经常会导致响应时间较长,而这可能会导致抓取工具取消请求且不将您的内容编入索引。此外,响应时间较长还可能会导致抓取工具在抓取您的内容并将其编入索引时放慢抓取速度。
done 解决问题
- 在构建流程中,为预渲染的 HTML 设置缓存,或为内容创建静态 HTML 版本。
- 确保在配置中启用缓存(例如,通过将抓取工具指向缓存)。
- 借助富媒体搜索结果测试或 webpagetest 等测试工具(使用 Google 抓取工具用户代理列表中的自定义用户代理字符串),检查抓取工具能否快速获取您的内容。您的请求不应超时。
网络组件无法按预期呈现
error 导致问题的原因:Shadow DOM 已与网页的其余部分隔离开来。呈现解决方案(如 Rendertron)无法看到被隔离的 shadow DOM 内的内容。 如需了解详情,请参阅网络组件最佳做法。
done 解决问题
- 为自定义元素和 shadow DOM 加载 webcomponents.js polyfills。
- 使用富媒体搜索结果测试或网址检查工具检查相应内容是否显示在渲染解决方案所渲染的 HTML 中。
缺少结构化数据
error 导致问题的原因:如果缺少结构化数据用户代理,或者输出中未包含 JSON-LD 脚本标记,可能会导致结构化数据错误。
done 解决问题
- 使用富媒体搜索结果测试进行测试,确保结构化数据出现在网页上。然后配置用户代理,以便使用桌面版或移动版 Googlebot 测试预渲染的内容。
- 确保 JSON-LD 脚本标记包含在内容的动态渲染 HTML 中。有关详情,请参阅所用呈现解决方案的文档。