This page explains how to set up and respond to quick commands for your Google Chat app.
A quick command is a way that users can invoke and interact with a
Chat app. To use a quick command, open the integration
menu by clicking Google Workspace Tools
/
). By selecting a quick command, the
Chat app is directly invoked without further inputs
from the user, allowing for fast user interactions.
Users can also invoke a Chat app through a slash command. In comparison to slash commands, quick commands are formatted with a more user-friendly name in the Chat UI, they are invoked immediately upon selection, and they don't require the user to remember or type out the name of the slash command.
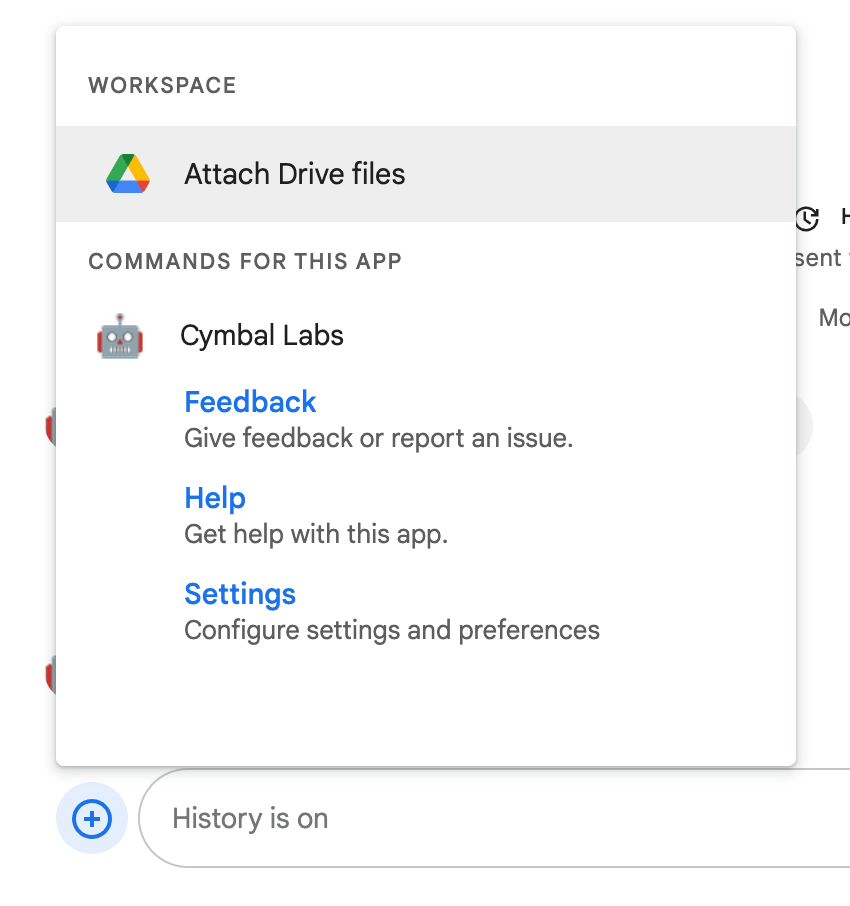
Set up a quick command in the Google Chat API
To create a quick command or update any existing slash commands to quick commands, you must specify information about the command in your Chat app's configuration for the Google Chat API.
To configure a quick command in the Google Chat API, complete the following steps:
In the Google Cloud console, click Menu > APIs & Services > Enabled APIs & Services > Google Chat API
Click Configuration.
Under Advanced settings, go to Triggers and check that the App command field contains a trigger, such as an HTTP endpoint or Apps Script function. You must use this trigger in the following section to respond to the quick command.
Under Commands, click Add a command.
Enter a command ID, name, description, and command type for the command:
- Command ID: a number from 1 to 1000 that your Chat app uses to recognize the command and return a response.
- Name: the display name for the command. Names can be up to 50
characters and can include special characters.
- Use short, descriptive, and actionable words or phrases to make the commands clear to the user. For example, use Update contact for a command that modifies a contact record.
- Description: the text that describes what the command does.
Descriptions can be up to 50 characters and can include special
characters.
- Keep the description short and clear so that users know what to expect when they invoke the command.
- Let users know if the Chat app replies to everyone in the space, or privately to the user who invokes the command. For example, for the quick command About, you could describe it as Learn about this app (Only visible to you).
- Command type: select Quick command.
Optional: If you want your Chat app to respond to the command with a dialog, select the Open a dialog checkbox.
Click Save.
The quick command is now configured for the Chat app.
Respond to a quick command
When users invoke a quick command, your Chat app
receives an event object that contains information about the quick command.
The event object contains an AppCommand
payload
with details about the command invoked (including the command ID), so that you
can return an appropriate response.
To respond to a quick command, you must implement the App command trigger so that your Chat app can handle any event objects that contain app command metadata.
The following code shows an example of a Chat app that
replies to the Help command. To respond to quick commands, the
Chat app handles event objects from an App command
trigger. When the payload of an event object contains a quick command ID,
Chat app returns the action DataActions
with a
createMessageAction
object:
Apps Script
// The ID of the slash command "/about".
// It's not enabled by default, set to the actual ID to enable it. You must
// use the same ID as set in the Google Chat API configuration.
const HELP_COMMAND_ID = 0;
/**
* Responds to an APP_COMMAND event in Google Chat.
*
* @param {Object} event the event object from Google Chat
*/
function onAppCommand(event) {
// Stores the Google Chat app command metadata as a variable.
const appCommandMetadata = event.chat.appCommandPayload.appCommandMetadata;
if (appCommandMetadata.appCommandType == "QUICK_COMMAND") {
// Executes the quick command logic based on its ID.
// Quick command IDs are set in the Google Chat API configuration.
switch (appCommandMetadata.appCommandId) {
case HELP_COMMAND_ID:
return { hostAppDataAction: { chatDataAction: { createMessageAction: { message: {
text: 'The Avatar app replies to Google Chat messages.'
}}}}};
}
}
}
To use this code sample, replace HELP_COMMAND_ID
with the
command ID that you specified when you
configured the quick command in the Chat API.
To test a quick command in Chat, open the integration menu by clicking
Google Workspace Tools and then
selecting your quick command.