This page explains how to configure a Google Chat app to respond to slash commands.
In Google Chat, add-ons appear to users as Google Chat apps. To learn more, see the Extend Google Chat overview.
A slash command is a common way that users invoke and interact with a Chat app. Slash commands also help users discover and use key features of a Chat app. To decide whether you should set up slash commands, and to understand how to design user interactions, see Define all user journeys in the Chat API documentation.
How users use slash commands
To use a slash command, users type a slash (/
) and then a short text command,
such as /about
to get information about the Chat app.
Users can discover available slash commands by typing a slash into
Google Chat, which displays a window that lists the available commands for the
Chat app:
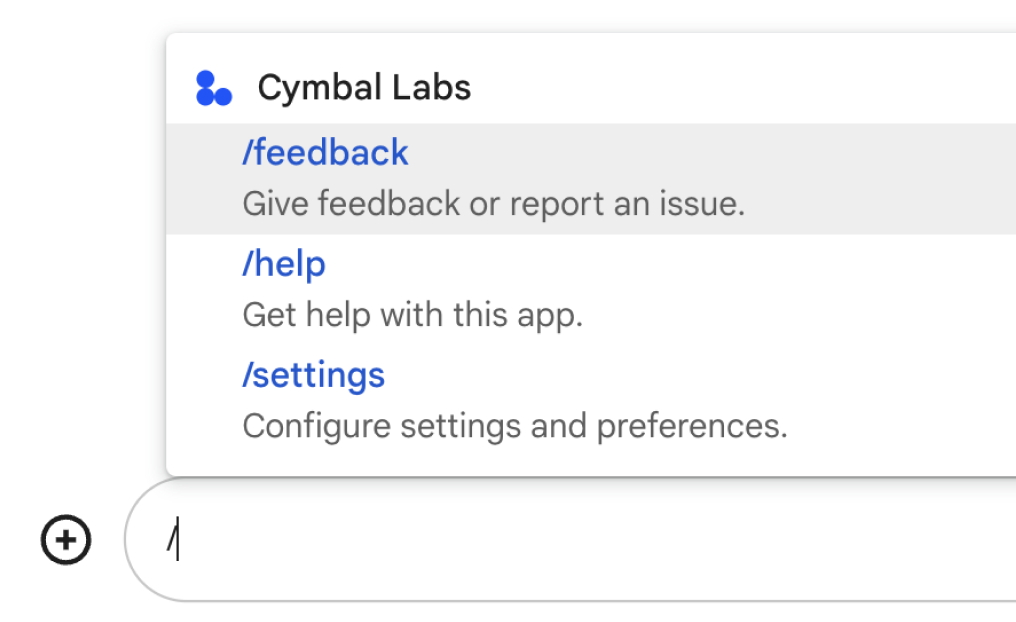
When a user sends a message that contains a slash command, the message is only visible to the user and the Chat app. If you've configured your Chat app to be added to spaces with multiple people, you might consider responding to the slash command privately, to keep the interaction private between the user and the Chat app.
For example, to learn about a Chat app that they
discover in a space, users could use commands such as /about
or /help
.
To avoid notifying everyone else in the space, the
Chat app can respond privately with information about
how to use the Chat app and get support.
Prerequisites
Node.js
A Google Workspace add-on that extends Google Chat. To build one, complete the HTTP quickstart.
Apps Script
A Google Workspace add-on that extends Google Chat. To build one, complete the Apps Script quickstart.
Set up a slash command
This section explains how to complete the following steps to set up a slash command:
- Create a name for your slash command.
- Configure the slash command in the Google Chat API.
Name your slash command
The name of a slash command is what users type in a Chat message to invoke the Chat app. A short description also appears below the name, to prompt users further about how to use the command:
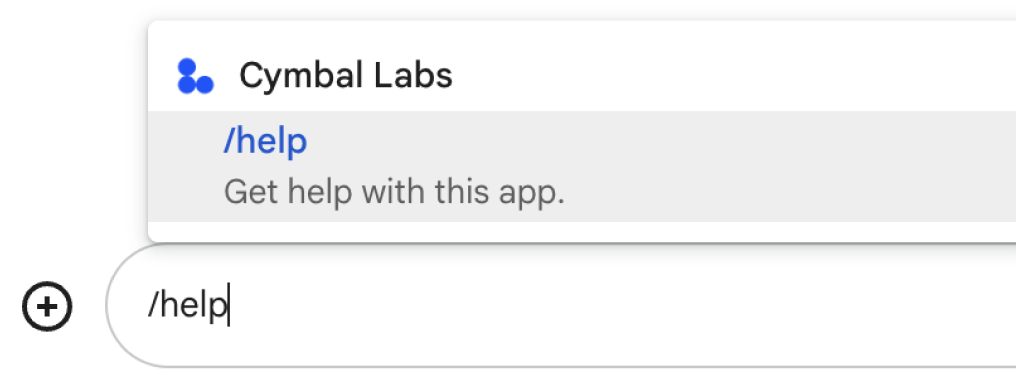
When choosing a name and description for your slash command, consider the following recommendations:
To name your slash command:
- Use short, descriptive, and actionable words or phrases to make the
commands clear and simple to the user. For example, instead of saying
/createAReminder
, use/remindMe
. - If your command contains more than one word, help users read the command
by using all lowercase for the first word and then capitalizing the first
letter of additional words. For example, instead of
/updatecontact
, use/updateContact
. - Consider whether to use a unique or common name for your command. If
your command describes a typical interaction or feature, you can use a
common name that users recognize and expect, such as
/settings
or/feedback
. Otherwise, try to use unique command names, because if your command name is the same for other Chat apps, the user must filter through similar commands to find and use yours.
- Use short, descriptive, and actionable words or phrases to make the
commands clear and simple to the user. For example, instead of saying
To describe your slash command:
- Keep the description short and clear so that users know what to expect when they invoke the command.
- Let users know if there are any formatting requirements for the command.
For example, if you create a
/remindMe
command that requires argument text, set the description to something likeRemind me to do [something] at [time]
. - Let users know if the Chat app replies to
everyone in the space, or privately to the user who invokes the command.
For example, for the slash command
/about
, you could describe it asLearn about this app (Only visible to you)
.
Configure your slash command in the Google Chat API
To create a slash command, you need to specify information about the command in your Chat app's configuration for the Google Chat API.
To configure a slash command in the Google Chat API, complete the following steps:
In the Google Cloud console, click Menu > APIs & Services > Enabled APIs & Services > Google Chat API
Click Configuration.
Under Advanced settings, go to Triggers and check that the MESSAGE field contains a trigger, such as an HTTP endpoint or Apps Script function. You must use this trigger to in the following section to respond to the slash command.
Under Slash commands, click Add a slash command.
Enter a name, command ID, and description for the command:
- Name: the display name for the command, and what users type to invoke your app. Must start with a slash, contain only text, and can be up to 50 characters.
- Description: the text that describes how to use and format the command. Descriptions can be up to 50 characters.
- Command ID: a number from 1 to 1000 that your Chat app uses to recognize the slash command and return a response.
Optional: If you want your Chat app to respond to the command with a dialog, select the Open a dialog checkbox.
Click Save.
The slash command is now configured for the Chat app.
Respond to a slash command
When users create a Chat message, your Chat app receives an event object that contains information about the message. The event object contains a payload with details about the command used in the message (including the command ID), so that you can return an appropriate response.
To respond to a slash command, you must implement the Message trigger so that your Chat app can handle any event objects that contain slash command metadata.

/help
to explain how to get support.The following code shows an example of a Chat app
that replies to the /about
slash command with a text message. To respond to
slash commands, the Chat app handles event objects from
a Message trigger. When the payload of an event object contains a slash
command ID, Chat app returns the action DataActions
with a createMessageAction
object:
Node.js
// The ID of the slash command "/about".
// It's not enabled by default, set to the actual ID to enable it. You must
// use the same ID as set in the Google Chat API configuration.
const ABOUT_COMMAND_ID = 0;
/**
* Google Cloud Function that responds to messages sent from a
* Google Chat space.
*
* @param {Object} req Request sent from Google Chat space
* @param {Object} res Response to send back
*/
exports.avatarApp = function avatarApp(req, res) {
if (req.method === 'GET' || !req.body.chat) {
return res.send('Hello! This function is meant to be used ' +
'in a Google Chat Space.');
}
// Stores the Google Chat event as a variable.
const chatMessage = req.body.chat.messagePayload.message;
if (chatMessage.slashCommand) {
// Executes the slash command logic based on its ID.
// Slash command IDs are set in the Google Chat API configuration.
switch (chatMessage.slashCommand.commandId) {
case ABOUT_COMMAND_ID:
return res.send({ hostAppDataAction: { chatDataAction: { createMessageAction: { message: {
text: 'The Avatar app replies to Google Chat messages.'
}}}}});
}
}
// Replies with the sender's avatar in a card otherwise.
const displayName = chatMessage.sender.displayName;
const avatarUrl = chatMessage.sender.avatarUrl;
res.send({ hostAppDataAction: { chatDataAction: { createMessageAction: { message: {
text: 'Here\'s your avatar',
cardsV2: [{
cardId: 'avatarCard',
card: {
name: 'Avatar Card',
header: {
title: `Hello ${displayName}!`,
},
sections: [{
widgets: [{
textParagraph: { text: 'Your avatar picture: ' }
}, {
image: { imageUrl: avatarUrl }
}]
}]
}
}]
}}}}});
};
Apps Script
// The ID of the slash command "/about".
// It's not enabled by default, set to the actual ID to enable it. You must
// use the same ID as set in the Google Chat API configuration.
const ABOUT_COMMAND_ID = 0;
/**
* Responds to a MESSAGE event in Google Chat.
*
* @param {Object} event the event object from Google Chat
*/
function onMessage(event) {
// Stores the Google Chat event as a variable.
const chatMessage = event.chat.messagePayload.message;
if (chatMessage.slashCommand) {
// Executes the slash command logic based on its ID.
// Slash command IDs are set in the Google Chat API configuration.
switch (chatMessage.slashCommand.commandId) {
case ABOUT_COMMAND_ID:
return { hostAppDataAction: { chatDataAction: { createMessageAction: { message: {
text: 'The Avatar app replies to Google Chat messages.'
}}}}};
}
}
// Replies with the sender's avatar in a card otherwise.
const displayName = chatMessage.sender.displayName;
const avatarUrl = chatMessage.sender.avatarUrl;
return { hostAppDataAction: { chatDataAction: { createMessageAction: { message: {
text: 'Here\'s your avatar',
cardsV2: [{
cardId: 'avatarCard',
card: {
name: 'Avatar Card',
header: {
title: `Hello ${displayName}!`,
},
sections: [{
widgets: [{
textParagraph: { text: 'Your avatar picture: ' }
}, {
image: { imageUrl: avatarUrl }
}]
}]
}
}]
}}}}};
}
To use this code sample, replace ABOUT_COMMAND_ID
with the
command ID that you specified when you
configured the slash command in the Chat API.