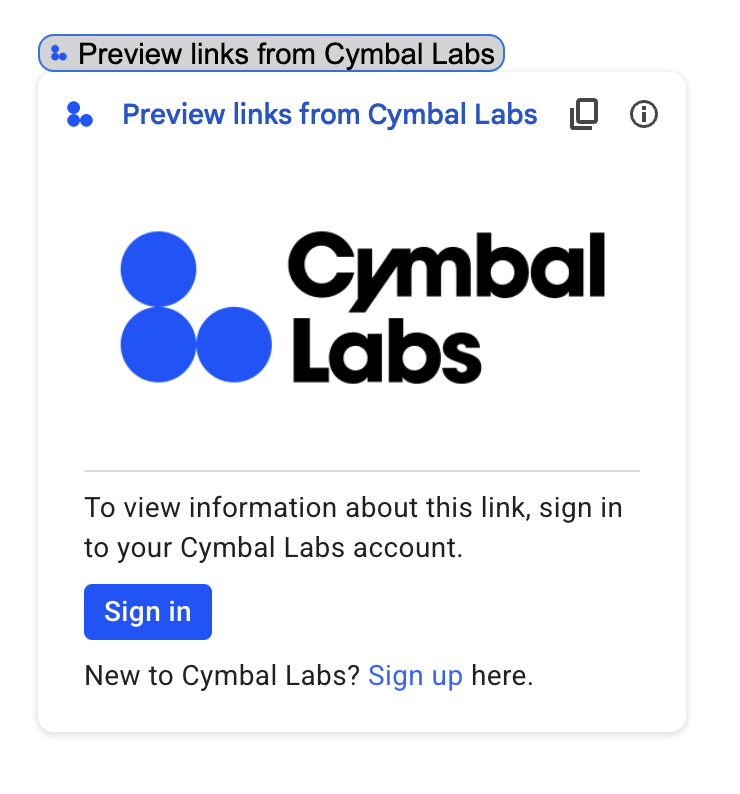
認証が必要なサードパーティのサービスまたは API に Google Workspace アドオンが接続すると、アドオンはユーザーにログインとアクセスの承認を求めるプロンプトを表示できます。
このページでは、以下の手順を含む認可フロー(OAuth など)を使用してユーザーを認証する方法について説明します。
- 承認が必要なときに検出します。
- ユーザーにサービスへのログインを求めるカード インターフェースを返す。
- ユーザーがサービスまたは保護されたリソースにアクセスできるように、アドオンを更新します。
アドオンでユーザー ID のみが必要な場合は、Google Workspace ID またはメールアドレスを使用してユーザーを直接認証できます。認証にメールアドレスを使用するには、JSON リクエストの検証をご覧ください。Google Apps Script を使用してアドオンを構築した場合は、アドオンをサードパーティのサービスに接続する方法について Apps Script のドキュメントをご覧ください。
承認が必要であることを検出する
アドオンを使用する場合、次のようなさまざまな理由で、保護されたリソースへのアクセスがユーザーに許可されていない場合があります。
- サードパーティ サービスに接続するためのアクセス トークンがまだ生成されていないか、期限切れです。
- アクセス トークンが、リクエストされたリソースを網羅していない。
- アクセス トークンがリクエストに必要なスコープを網羅していない。
アドオンでこれらのケースを検出し、ユーザーがサービスにログインしてアクセスできるようにする必要があります。
サービスへのログインを求めるメッセージをユーザーに表示する
アドオンは、認証が必要であることを検出すると、カード インターフェースを返し、ユーザーにサービスへのログインを求める必要があります。ログインカードからユーザーをリダイレクトし、インフラストラクチャでサードパーティの認証と認可を完了する必要があります。
移行先アプリを Google ログインで保護し、ログイン時に発行された ID トークンを使用してユーザー ID を取得することをおすすめします。サブクレームにはユーザーの一意の ID が含まれ、アドオンの ID と関連付けることができます。
ログインカードを作成して返す
サービスのログインカードでは、Google の基本認証カードを使用できます。また、カードをカスタマイズして、組織のロゴなどの追加情報を表示することもできます。アドオンを一般公開する場合は、カスタムカードを使用する必要があります。
基本認証カード
次の画像は、Google の基本認証カードの例を示しています。
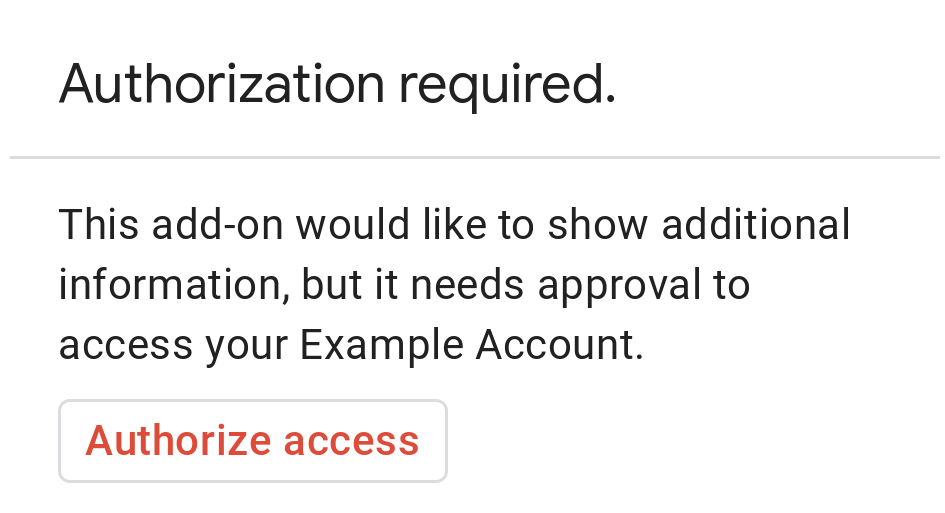
基本認証カードを使用するには、次の JSON レスポンスを返します。
{ "basic_authorization_prompt": { "authorization_url": "AUTHORIZATION_REDIRECT", "resource": "RESOURCE_DISPLAY_NAME" } }
次のように置き換えます。
AUTHORIZATION_REDIRECT
: 承認を処理するウェブアプリの URL。RESOURCE_DISPLAY_NAME
: 保護されたリソースまたはサービスの表示名。この名前は、認可プロンプトにユーザーに表示されます。たとえば、RESOURCE_DISPLAY_NAME
がExample Account
の場合、「このアドオンは追加情報を表示しますが、Example Account の利用には承認が必要です」というメッセージが表示されます。
承認が完了すると、ユーザーは、保護されたリソースにアクセスするためにアドオンを更新するよう求められます。
カスタム承認カード
認証プロンプトを変更するには、サービスのログイン エクスペリエンス用のカスタムカードを作成します。
アドオンを公開する場合は、カスタムの承認カードを使用する必要があります。Google Workspace Marketplace の公開要件については、アプリの審査についてをご覧ください。
次の画像は、アドオンのホームページのカスタム認証カードの例を示しています。カードには、ロゴ、説明、ログインボタンが含まれています。
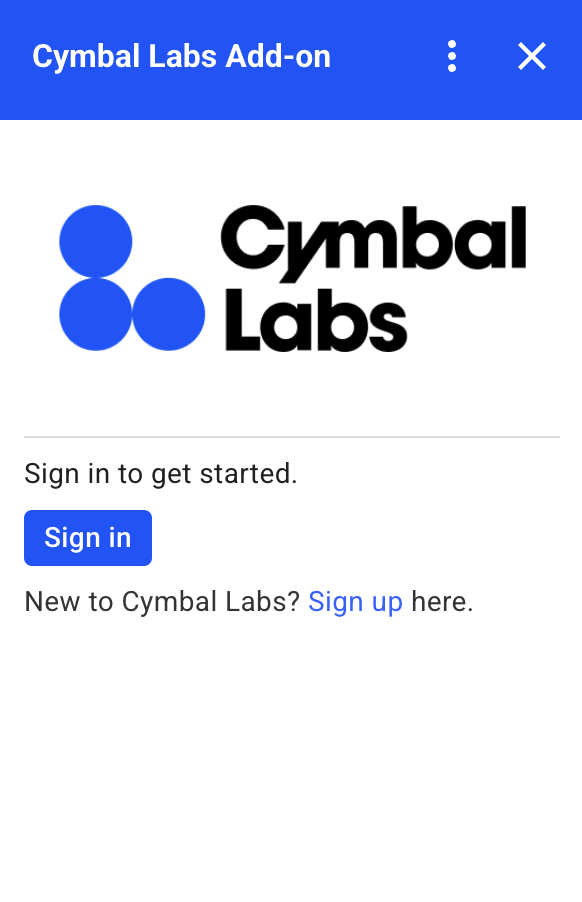
このカスタムカードの例を使用するには、次の JSON レスポンスを返します。
{ "custom_authorization_prompt": { "action": { "navigations": [ { "pushCard": { "sections": [ { "widgets": [ { "image": { "imageUrl": "LOGO_URL", "altText": "LOGO_ALT_TEXT" } }, { "divider": {} }, { "textParagraph": { "text": "DESCRIPTION" } }, { "buttonList": { "buttons": [ { "text": "Sign in", "onClick": { "openLink": { "url": "AUTHORIZATION_REDIRECT", "onClose": "RELOAD", "openAs": "OVERLAY" } }, "color": { "red": 0, "green": 0, "blue": 1, "alpha": 1, } } ] } }, { "textParagraph": { "text": "TEXT_SIGN_UP" } } ] } ] } } ] } } }
次のように置き換えます。
LOGO_URL
: ロゴまたは画像の URL。公開 URL であることが必要です。LOGO_ALT_TEXT
: ロゴまたは画像の代替テキスト(Cymbal Labs Logo
など)。DESCRIPTION
: ユーザーにログインを促すフレーズ(Sign in to get started
など)。- ログインボタンを更新するには:
AUTHORIZATION_REDIRECT
: 承認を処理するウェブアプリの URL。- 省略可: ボタンの色を変更するには、
color
フィールドの RGBA 浮動小数点値を更新します。
TEXT_SIGN_UP
: アカウントをお持ちでない場合に、アカウントの作成を求めるテキスト。例:New to Cymbal Labs? <a href=\"https://www.example.com/signup\">Sign up</a> here