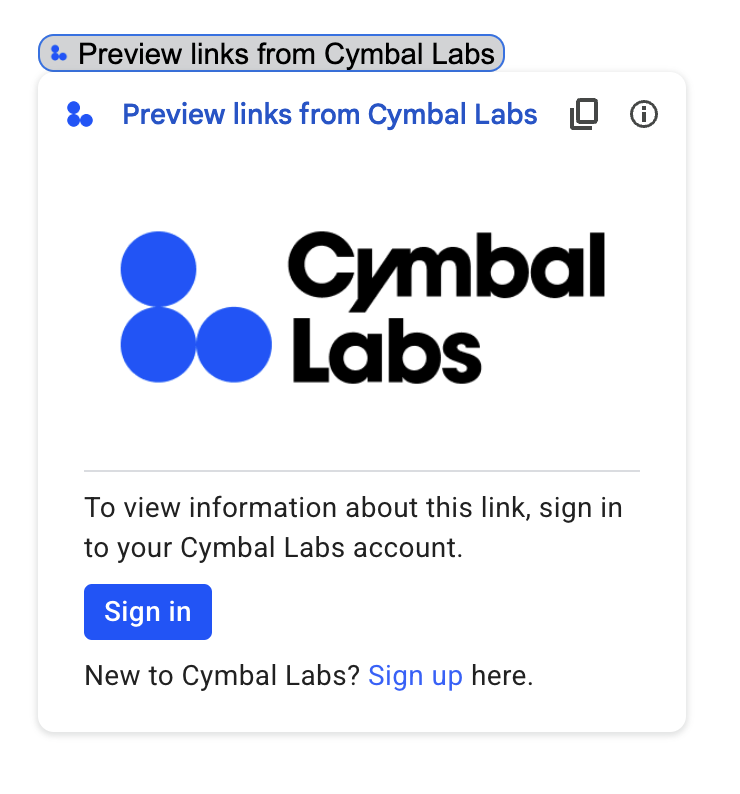
Google Workspace 부가기능이 승인이 필요한 서드 파티 서비스 또는 API에 연결되는 경우 부가기능에서 사용자에게 로그인하고 액세스를 승인하라는 메시지를 표시할 수 있습니다.
이 페이지에서는 다음 단계가 포함된 승인 흐름 (예: OAuth)을 사용하여 사용자를 인증하는 방법을 설명합니다.
- 승인이 필요한 경우 이를 감지합니다.
- 사용자에게 서비스에 로그인하라는 메시지를 표시하는 카드 인터페이스를 반환합니다.
- 사용자가 서비스 또는 보호되는 리소스에 액세스할 수 있도록 부가기능을 새로고침합니다.
부가기능에 사용자 ID만 필요한 경우 Google Workspace ID 또는 이메일 주소를 사용하여 사용자를 직접 인증할 수 있습니다. 인증에 이메일 주소를 사용하려면 JSON 요청 유효성 검사를 참조하세요. Google Apps Script를 사용하여 부가기능을 빌드한 경우 Apps Script 문서에서 부가기능을 타사 서비스에 연결하는 방법을 알아보세요.
승인이 필요함을 감지
부가기능을 사용할 때 다음과 같은 여러 가지 이유로 보호된 리소스에 액세스하도록 사용자에게 승인되지 않을 수 있습니다.
- 서드 파티 서비스에 연결하기 위한 액세스 토큰이 아직 생성되지 않았거나 만료되었습니다.
- 액세스 토큰이 요청된 리소스를 포함하지 않습니다.
- 액세스 토큰이 요청의 필수 범위를 포함하지 않습니다.
부가기능은 사용자가 로그인하고 서비스에 액세스할 수 있도록 이러한 사례를 감지해야 합니다.
사용자에게 서비스에 로그인하라는 메시지 표시
부가기능에서 승인이 필요함을 감지하면 사용자에게 서비스에 로그인하라는 메시지를 표시하는 카드 인터페이스를 반환해야 합니다. 인프라에서 타사 인증 및 승인 프로세스를 완료하도록 로그인 카드는 사용자를 리디렉션해야 합니다.
Google 로그인으로 대상 앱을 보호하고 로그인 중에 발급된 ID 토큰을 사용하여 사용자 ID를 가져오는 것이 좋습니다. 하위 클레임에는 사용자의 고유 ID가 포함되며 부가기능의 ID와 연결할 수 있습니다.
로그인 카드 빌드 및 반환
서비스 로그인 카드에 Google의 기본 승인 카드를 사용하거나 카드를 맞춤설정하여 조직 로고와 같은 추가 정보를 표시할 수 있습니다. 부가기능을 공개적으로 게시하는 경우 맞춤 카드를 사용해야 합니다.
기본 승인 카드
다음 이미지는 Google의 기본 승인 카드의 예를 보여줍니다.
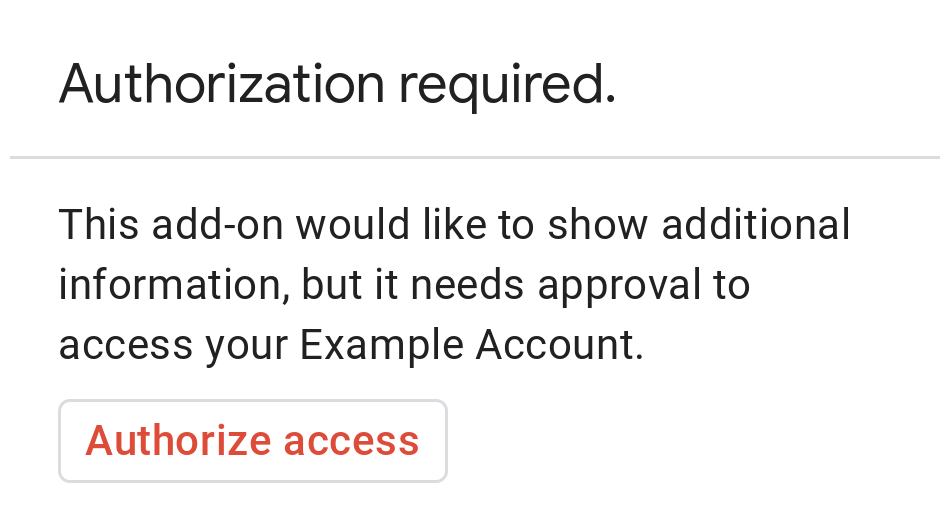
기본 승인 카드를 사용하려면 다음 JSON 응답을 반환합니다.
{ "basic_authorization_prompt": { "authorization_url": "AUTHORIZATION_REDIRECT", "resource": "RESOURCE_DISPLAY_NAME" } }
다음을 바꿉니다.
AUTHORIZATION_REDIRECT
: 승인을 처리하는 웹 앱의 URL입니다.RESOURCE_DISPLAY_NAME
: 보호되는 리소스 또는 서비스의 표시 이름입니다. 이 이름은 승인 메시지에서 사용자에게 표시됩니다. 예를 들어RESOURCE_DISPLAY_NAME
가Example Account
이면 '이 부가기능에서 추가 정보를 표시하려고 하지만 Example 계정에 액세스하려면 승인이 필요합니다'라는 메시지가 표시됩니다.
승인을 완료한 후 보호되는 리소스에 액세스하려면 부가기능을 새로고침하라는 메시지가 표시됩니다.
맞춤 승인 카드
서비스 로그인 환경을 위한 커스텀 카드를 만들면 승인 메시지를 수정할 수 있습니다.
부가기능을 공개적으로 게시하는 경우 맞춤 승인 카드를 사용해야 합니다. Google Workspace Marketplace의 게시 요구사항에 관한 자세한 내용은 앱 검토 정보를 참고하세요.
다음 이미지는 부가기능 홈페이지의 커스텀 승인 카드 예시를 보여줍니다. 카드에는 로고, 설명, 로그인 버튼이 포함됩니다.
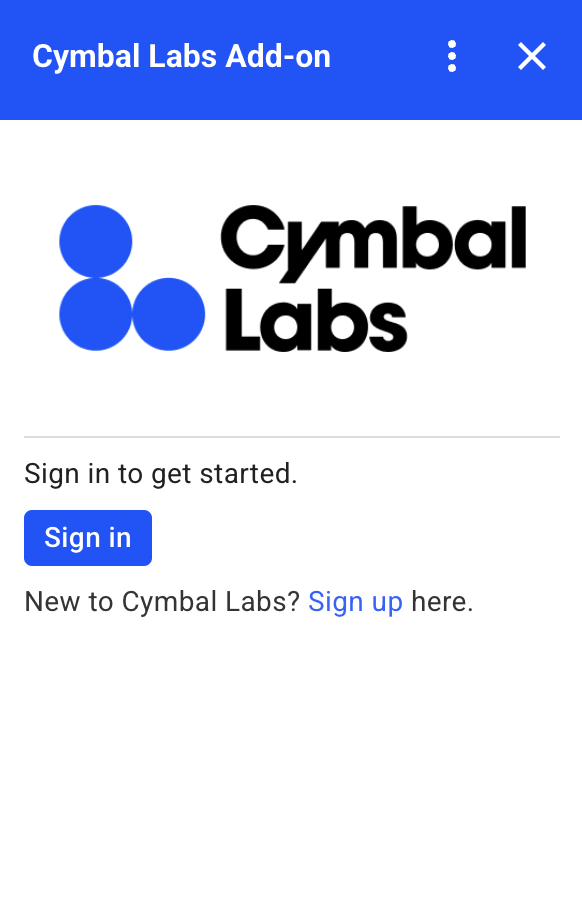
이 맞춤 카드 예를 사용하려면 다음 JSON 응답을 반환합니다.
{ "custom_authorization_prompt": { "action": { "navigations": [ { "pushCard": { "sections": [ { "widgets": [ { "image": { "imageUrl": "LOGO_URL", "altText": "LOGO_ALT_TEXT" } }, { "divider": {} }, { "textParagraph": { "text": "DESCRIPTION" } }, { "buttonList": { "buttons": [ { "text": "Sign in", "onClick": { "openLink": { "url": "AUTHORIZATION_REDIRECT", "onClose": "RELOAD", "openAs": "OVERLAY" } }, "color": { "red": 0, "green": 0, "blue": 1, "alpha": 1, } } ] } }, { "textParagraph": { "text": "TEXT_SIGN_UP" } } ] } ] } } ] } } }
다음을 바꿉니다.
LOGO_URL
: 로고 또는 이미지의 URL입니다. 공개 URL이어야 합니다.LOGO_ALT_TEXT
: 로고 또는 이미지의 대체 텍스트입니다(예:Cymbal Labs Logo
).DESCRIPTION
: 사용자의 로그인을 위한 클릭 유도 문구입니다(예:Sign in to get started
).- 로그인 버튼을 업데이트하려면 다음 안내를 따르세요.
AUTHORIZATION_REDIRECT
: 승인을 처리하는 웹 앱의 URL입니다.- 선택사항: 버튼 색상을 변경하려면
color
필드의 RGBA 부동 소수점 값을 업데이트합니다.
TEXT_SIGN_UP
: 계정이 없는 사용자에게 계정을 생성하라는 메시지를 표시하는 텍스트입니다. 예를 들면 다음과 같습니다.New to Cymbal Labs? <a href=\"https://www.example.com/signup\">Sign up</a> here