本页面介绍了 Chat 应用如何打开对话框来响应用户。
对话框是基于卡片的窗口式界面,可从 Chat 聊天室或消息中打开。对话框及其内容仅对打开它的用户可见。
Chat 应用可以使用对话框向 Chat 用户请求信息并收集其信息,包括多步骤表单。如需详细了解如何构建表单输入,请参阅收集和处理用户信息。
前提条件
Node.js
一款已启用互动功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Python
启用了交互功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Java
启用了交互功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Apps 脚本
启用了交互功能的 Google Chat 应用。如需在 Apps 脚本中创建交互式 Chat 应用,请完成此快速入门。
打开对话框
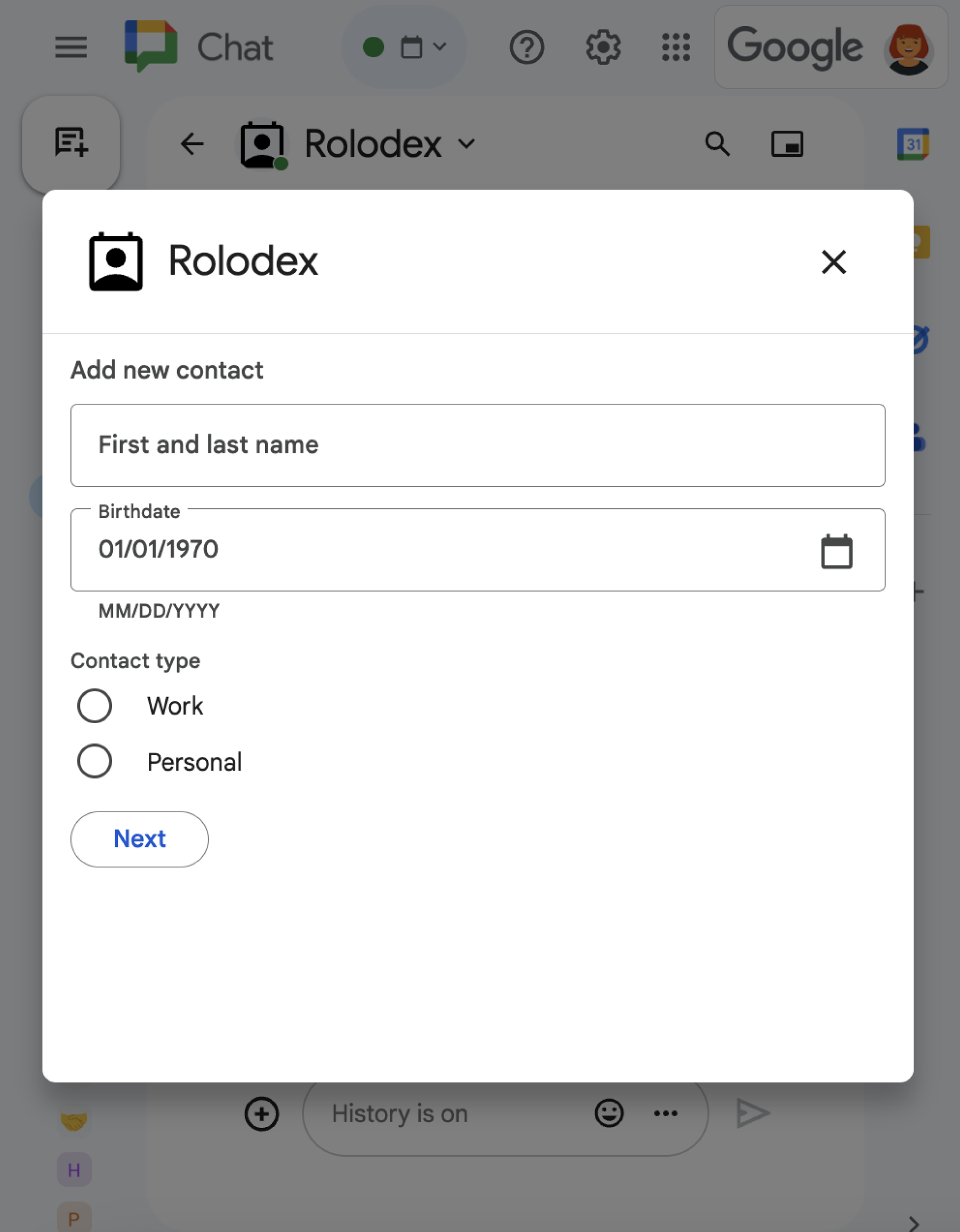
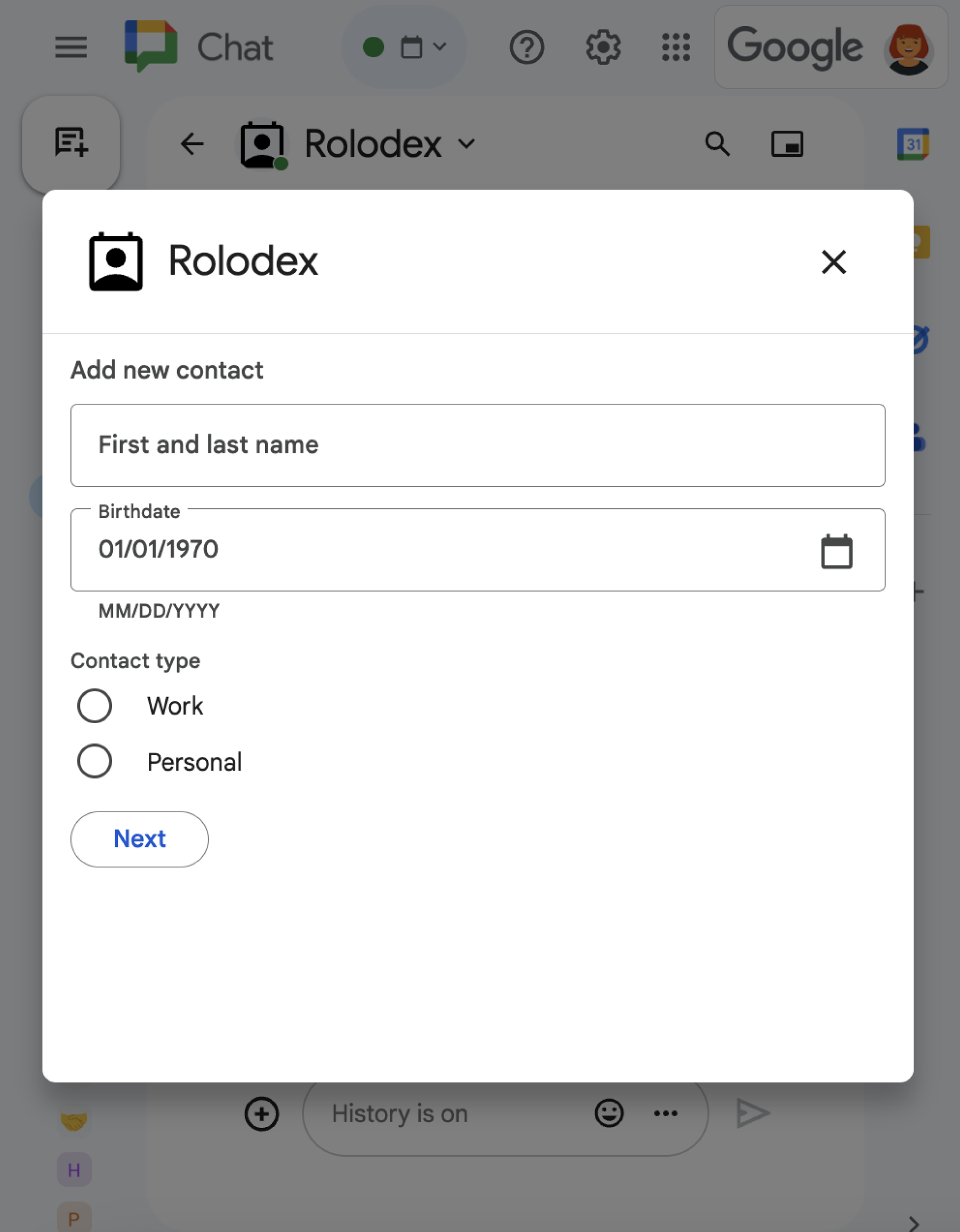
本部分介绍了如何通过执行以下操作来响应和设置对话框:
- 通过用户互动触发对话框请求。
- 通过返回并打开一个对话框来处理请求。
- 用户提交信息后,通过关闭对话框或返回另一个对话框来处理提交内容。
触发对话请求
Chat 应用只能打开对话框来响应用户互动,例如使用斜杠命令或点击卡片消息中的按钮。
如需通过对话框响应用户,Chat 应用必须构建触发对话框请求的互动,例如:
- 响应斜杠命令。如需通过斜杠命令触发请求,您必须在配置命令时勾选打开对话框复选框。
- 响应消息中的按钮点击,可以在卡片中或消息底部进行响应。如需通过消息中的按钮触发请求,您可以通过将按钮的
interaction
设置为OPEN_DIALOG
来配置按钮的onClick
操作。 - 响应 Chat 应用首页中的按钮点击。 如需了解如何从首页打开对话框,请参阅为 Google Chat 应用构建首页。
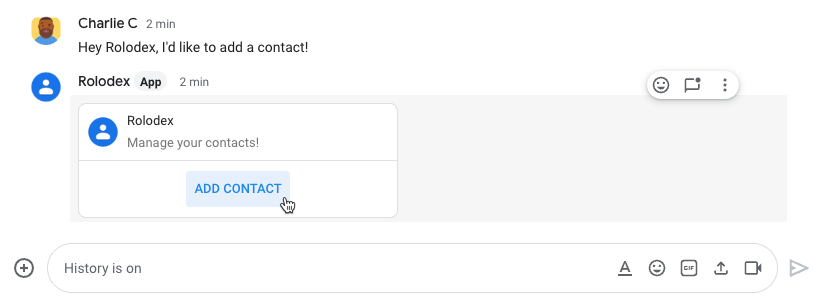
/addContact
斜杠命令。该消息还包含一个按钮,用户可以点击该按钮来触发相应命令。
以下代码示例展示了如何通过卡片消息中的按钮触发对话框请求。如需打开该对话框,请将 button.interaction
字段设置为 OPEN_DIALOG
:
打开初始对话框
当用户触发对话框请求时,您的 Chat 应用会收到互动事件,该事件在 Chat API 中表示为 event
类型。如果互动触发了对话请求,事件的 dialogEventType
字段会设置为 REQUEST_DIALOG
。
如需打开对话框,Chat 应用可以通过返回 actionResponse
对象(将 type
设为 DIALOG
)和 Message
对象来响应请求。如需指定对话框的内容,请添加以下对象:
- 一个
actionResponse
对象,其type
设置为DIALOG
。 dialogAction
对象。body
字段包含要在卡片中显示的界面 (UI) 元素,包括一个或多个sections
widget。如需从用户处收集信息,您可以指定表单输入 widget 和按钮 widget。如需详细了解如何设计表单输入,请参阅收集和处理用户信息。
以下代码示例展示了 Chat 应用如何返回打开对话框的响应:
处理对话框提交内容
当用户点击用于提交对话框的按钮时,您的 Chat 应用会收到 CARD_CLICKED
互动事件,其中 dialogEventType
为 SUBMIT_DIALOG
。
您的 Chat 应用必须通过执行以下操作之一来处理互动事件:
可选:返回其他对话框
用户提交初始对话框后,Chat 应用可以返回一个或多个其他对话框,以帮助用户在提交前查看信息、填写多步骤表单或动态填充表单内容。
为了处理用户输入的数据,Chat 应用会使用 event.common.formInputs
对象。如需详细了解如何从输入 widget 检索值,请参阅收集和处理用户信息。
如需跟踪用户从初始对话框输入的任何数据,您必须向用于打开下一个对话框的按钮添加参数。如需了解详情,请参阅将数据转移到其他卡。
在此示例中,Chat 应用会打开一个初始对话框,该对话框会在提交之前打开第二个对话框以供确认:
关闭对话框
当用户点击对话框中的按钮时,您的 Chat 应用会执行其关联的操作,并为事件对象提供以下信息:
eventType
为CARD_CLICKED
。dialogEventType
为SUBMIT_DIALOG
。
Chat 应用应返回一个 ActionResponse
对象,并将其 type
设置为 DIALOG
和 dialogAction
。
可选:显示通知
关闭该对话框时,您还可以显示文本通知。
Chat 应用可以返回 ActionResponse
并设置 actionStatus
,从而返回成功或错误通知。
以下示例会检查参数是否有效,并根据结果通过文本通知关闭对话框:
如需详细了解如何在对话框之间传递参数,请参阅将数据传输到另一张卡片。
可选:发送确认消息
关闭该对话框时,您还可以发送新消息或更新现有消息。
如需发送新消息,请返回一个 ActionResponse
对象,并将 type
设置为 NEW_MESSAGE
。以下示例会通过文本通知和确认文本消息关闭对话框:
如需更新消息,请返回包含更新后消息的 actionResponse
对象,并将 type
设置为以下之一:
问题排查
当 Google Chat 应用或卡片返回错误时,Chat 界面会显示一条消息,提示“出了点问题”。或“无法处理您的请求”。有时,Chat 界面不会显示任何错误消息,但 Chat 应用或卡片会产生意外结果;例如,卡片消息可能不会显示。
虽然 Chat 界面中可能未显示错误消息,但当为 Chat 应用启用错误日志记录功能时,系统会显示描述性错误消息和日志数据,帮助您修复错误。如需有关查看、调试和修复错误的帮助,请参阅排查和修复 Google Chat 错误。
相关主题
- 查看联系人管理器示例,这是一款使用对话框收集联系信息的 Chat 应用。
- 从 Google Chat 应用首页打开对话。
- 设置和响应斜杠命令
- 处理用户输入的信息