本指南介绍了 Google Chat 应用如何通过在基于卡片的界面中构建表单输入项来收集和处理用户信息。
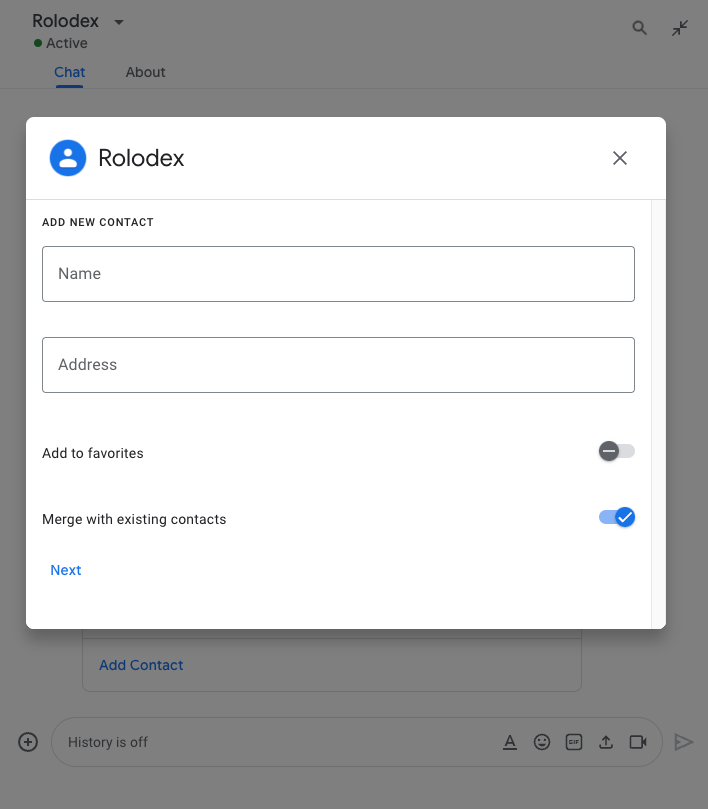
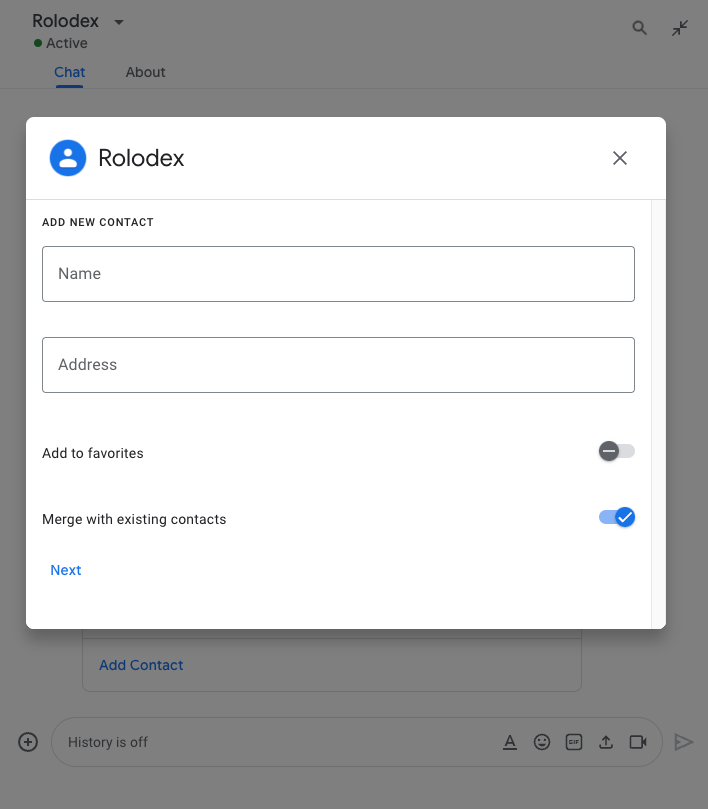
Chat 应用会向用户请求信息,以便在 Chat 中或之外执行操作,包括通过以下方式:
- 配置设置。例如,让用户自定义通知设置,或配置并将 Chat 应用添加到一个或多个聊天室。
- 在其他 Google Workspace 应用中创建或更新信息。例如,允许用户创建 Google 日历活动。
- 允许用户访问和更新其他应用或 Web 服务中的资源。例如,Chat 应用可以帮助用户直接在 Chat 聊天室中更新支持服务工单的状态。
前提条件
Node.js
启用了交互功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Python
启用了交互功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Java
启用了交互功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Apps 脚本
启用了交互功能的 Google Chat 应用。如需在 Apps 脚本中创建交互式 Chat 应用,请完成此快速入门。
使用卡片构建表单
为了收集信息,Chat 应用会设计表单及其输入内容,并将其构建为卡片。如需向用户显示卡片,Chat 应用可以使用以下 Chat 界面:
Chat 应用可以使用以下微件构建卡片:
用于向用户请求信息的表单输入 widget。(可选)您可以为表单输入 widget 添加验证,以确保用户正确输入和设置信息格式。聊天应用可以使用以下表单输入 widget:
在以下示例中,卡片使用文本输入框、日期时间选择器和选择输入框来收集联系信息:
如需查看使用此联系表单的 Chat 应用示例,请参阅以下代码:
Node.js
Python
Java
Apps 脚本
如需查看更多可用于收集信息的交互式 widget 示例,请参阅设计交互式卡片或对话框。
接收来自互动 widget 的数据
每当用户点击按钮时,聊天应用都会收到包含交互相关信息的 CARD_CLICKED
互动事件。CARD_CLICKED
互动事件的载荷包含一个 common.formInputs
对象,其中包含用户输入的任何值。
您可以从对象 common.formInputs.WIDGET_NAME
检索值,其中 WIDGET_NAME 是您为该 widget 指定的 name
字段。这些值会以 widget 的特定数据类型(表示为 Inputs
对象)的形式返回。
以下是 CARD_CLICKED
互动事件的一部分,其中用户为每个 widget 输入了值:
HTTP
{
"type": "CARD_CLICKED",
"common": { "formInputs": {
"contactName": { "stringInputs": {
"value": ["Kai 0"]
}},
"contactBirthdate": { "dateInput": {
"msSinceEpoch": 1000425600000
}},
"contactType": { "stringInputs": {
"value": ["Personal"]
}}
}}
}
Apps 脚本
{
"type": "CARD_CLICKED",
"common": { "formInputs": {
"contactName": { "": { "stringInputs": {
"value": ["Kai 0"]
}}},
"contactBirthdate": { "": { "dateInput": {
"msSinceEpoch": 1000425600000
}}},
"contactType": { "": { "stringInputs": {
"value": ["Personal"]
}}}
}}
}
如需接收数据,您的 Chat 应用需要处理互动事件,以获取用户输入到 widget 中的值。下表展示了如何获取给定表单输入 widget 的值。对于每个 widget,该表会显示 widget 接受的数据类型、值在互动事件中的存储位置,以及示例值。
表单输入微件 | 输入数据的类型 | 互动事件中的输入值 | 示例值 |
---|---|---|---|
textInput |
stringInputs |
event.common.formInputs.contactName.stringInputs.value[0] |
Kai O |
selectionInput |
stringInputs |
如需获取第一个或唯一值,请使用 event.common.formInputs.contactType.stringInputs.value[0] |
Personal |
仅接受日期的 dateTimePicker 。 |
dateInput |
event.common.formInputs.contactBirthdate.dateInput.msSinceEpoch 。 |
1000425600000 |
将数据转移到其他卡
用户提交卡片信息后,您可能需要返回其他卡片才能执行以下任一操作:
- 通过创建不同的部分,帮助用户填写较长的表单。
- 让用户预览并确认初始卡片中的信息,以便他们在提交前检查自己的回答。
- 动态填充表单的其余部分。例如,为了提示用户创建预约,Chat 应用可以显示一个初始卡片来请求预约原因,然后填充另一张卡片,根据预约类型提供空闲时间。
如需从初始卡片传输数据输入,您可以使用包含微件 name
和用户输入的值的 actionParameters
构建 button
微件,如以下示例所示:
Node.js
Python
Java
Apps 脚本
当用户点击该按钮时,您的 Chat 应用会收到 CARD_CLICKED
互动事件,您可以从中接收数据。
回复表单提交内容
从卡片消息或对话框收到数据后,Chat 应用会通过确认收到或返回错误来响应。
在以下示例中,Chat 应用发送一条短信,确认已成功收到通过对话框或卡片消息提交的表单。
Node.js
Python
Java
Apps 脚本
如需处理和关闭对话框,您需要返回一个 ActionResponse
对象,用于指定您是要发送确认消息、更新原始消息或卡片,还是只关闭对话框。如需了解相关步骤,请参阅关闭对话框。
问题排查
当 Google Chat 应用或卡片返回错误时,Chat 界面会显示“出了点问题”消息。或“无法处理您的请求”。有时,Chat 界面不会显示任何错误消息,但 Chat 应用或卡片会产生意外结果;例如,卡片消息可能不会显示。
虽然 Chat 界面中可能不会显示错误消息,但当 Chat 应用的错误日志记录功能处于开启状态时,描述性错误消息和日志数据可帮助您修正错误。如需有关查看、调试和修复错误的帮助,请参阅排查和修复 Google Chat 错误。
相关主题
- 查看“联系人管理器”示例,这是一个 Chat 应用,会提示用户通过卡片消息和对话框填写联系表单。
- 打开互动式对话框