本页介绍了如何使用 Google Chat 应用构建私信首页。首页(在 Google Chat API 中称为应用首页)是一种可自定义的卡片界面,会显示在用户与 Chat 应用之间的私信聊天室的首页标签页中。

您可以使用应用首页分享与 Chat 应用互动或让用户通过 Chat 访问和使用外部服务或工具的提示。
使用卡片制作工具设计和预览 Chat 应用的消息和界面:
打开卡片制作工具前提条件
Node.js
启用了交互功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Python
一款已启用互动功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Java
启用了交互功能的 Google Chat 应用。如需使用 HTTP 服务创建交互式 Chat 应用,请完成此快速入门。
Apps 脚本
启用了交互功能的 Google Chat 应用。如需使用 Apps 脚本创建交互式 Chat 应用,请完成此快速入门。
为 Chat 应用配置应用首页
如需支持应用主屏幕,您必须将 Chat 应用配置为接收 APP_HOME
互动事件。每当用户通过与 Chat 应用的私信点击首页标签页时,您的 Chat 应用就会收到此事件。
如需在 Google Cloud 控制台中更新配置设置,请执行以下操作:
在 Google Cloud 控制台中,依次点击菜单 > 更多产品 > Google Workspace > 产品库 > Google Chat API。
点击管理,然后点击配置标签页。
在互动功能下,前往功能部分以配置应用主屏幕:
- 选中接收 1 对 1 消息复选框。
- 选中支持应用主屏幕复选框。
如果您的 Chat 应用使用 HTTP 服务,请前往连接设置,然后为应用首页网址字段指定端点。您可以使用在 HTTP 端点网址字段中指定的网址。
点击保存。
构建应用首页卡片
当用户打开应用主屏幕时,您的 Chat 应用必须通过返回包含 pushCard
导航和 Card
的 RenderActions
实例来处理 APP_HOME
互动事件。为了打造互动式体验,卡片可以包含互动式 widget,例如 Chat 应用可以处理的按钮或文本输入,并使用其他卡片或对话框进行响应。
在以下示例中,Chat 应用会显示初始应用首页卡片,其中显示了卡片的创建时间和一个按钮。当用户点击该按钮时,Chat 应用会返回更新后的卡片,其中会显示更新后的卡片的创建时间。
Node.js
Python
Java
Apps 脚本
实现在所有 APP_HOME
互动事件之后调用的 onAppHome
函数:
此示例通过返回卡片 JSON 来发送卡片消息。您还可以使用 Apps Script 卡片服务。
响应应用主屏幕互动
如果您的初始应用首页卡片包含互动式微件(例如按钮或选择输入),则 Chat 应用必须通过返回带有 updateCard
导航的 RenderActions
实例来处理相关互动事件。如需详细了解如何处理 Interactive Widget,请参阅处理用户输入的信息。
在上一个示例中,初始应用首页卡片包含一个按钮。每当用户点击该按钮时,CARD_CLICKED
互动事件都会触发函数 updateAppHome
以刷新应用主屏幕卡片,如以下代码所示:
打开对话框
Chat 应用还可以通过打开对话框来响应应用首页中的互动。
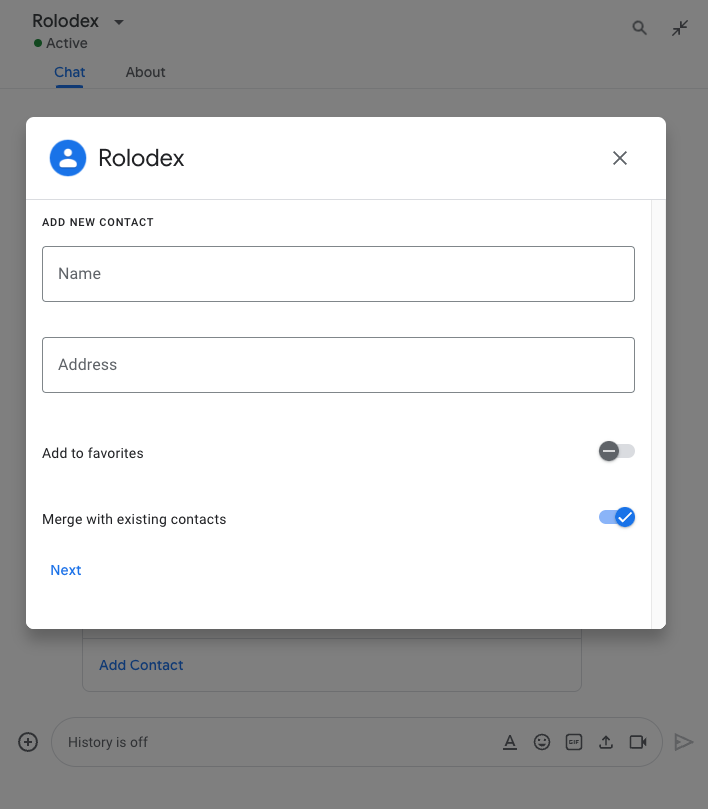
如需从应用主屏幕打开对话框,请通过返回 renderActions
(包含 Card
对象的 updateCard
导航)来处理相关的互动事件。在以下示例中,聊天应用通过处理 CARD_CLICKED
互动事件并打开对话框来响应应用主屏幕卡片中的按钮点击:
{ renderActions: { action: { navigations: [{ updateCard: { sections: [{
header: "Add new contact",
widgets: [{ "textInput": {
label: "Name",
type: "SINGLE_LINE",
name: "contactName"
}}, { textInput: {
label: "Address",
type: "MULTIPLE_LINE",
name: "address"
}}, { decoratedText: {
text: "Add to favorites",
switchControl: {
controlType: "SWITCH",
name: "saveFavorite"
}
}}, { decoratedText: {
text: "Merge with existing contacts",
switchControl: {
controlType: "SWITCH",
name: "mergeContact",
selected: true
}
}}, { buttonList: { buttons: [{
text: "Next",
onClick: { action: { function: "openSequentialDialog" }}
}]}}]
}]}}]}}}
如需关闭对话框,请处理以下互动事件:
CLOSE_DIALOG
:关闭对话框并返回到 Chat 应用的初始应用首页卡片。CLOSE_DIALOG_AND_EXECUTE
:关闭对话框并刷新应用首页卡片。
以下代码示例使用 CLOSE_DIALOG
关闭对话框并返回到应用首页卡片:
{ renderActions: { action: {
navigations: [{ endNavigation: { action: "CLOSE_DIALOG" }}]
}}}
如需从用户收集信息,您还可以构建顺序对话框。如需了解如何构建顺序对话框,请参阅打开和响应对话框。