このページでは、Google Chat アプリのダイレクト メッセージのホームページを作成する方法について説明します。ホームページ(Google Chat API では「アプリホーム」)は、ユーザーと Chat アプリ間のダイレクト メッセージ スペースの [ホーム] タブに表示される、カスタマイズ可能なカード インターフェースです。

アプリホームを使用すると、Chat アプリの操作方法や、ユーザーが Chat から外部サービスやツールにアクセスして使用できるようにする方法に関するヒントを共有できます。
カードビルダーを使用して、Chat 用アプリのメッセージとユーザー インターフェースを設計し、プレビューします。
カードビルダーを開く前提条件
Node.js
インタラクティブ機能が有効になっている Google Chat アプリ。HTTP サービスを使用してインタラクティブな Chat アプリを作成するには、このクイックスタートを完了してください。
Python
インタラクティブ機能が有効になっている Google Chat アプリ。HTTP サービスを使用してインタラクティブな Chat アプリを作成するには、このクイックスタートを完了してください。
Java
インタラクティブ機能が有効になっている Google Chat アプリ。HTTP サービスを使用してインタラクティブな Chat アプリを作成するには、このクイックスタートを完了してください。
Apps Script
インタラクティブ機能が有効になっている Google Chat アプリ。Apps Script でインタラクティブな Chat アプリを作成するには、このクイックスタートを完了してください。
Chat アプリのアプリホームを構成する
アプリホームをサポートするには、APP_HOME
インタラクション イベントを受信するように Chat アプリを構成する必要があります。Chat アプリは、ユーザーが Chat アプリのダイレクト メッセージから [ホーム] タブをクリックするたびに、このイベントを受信します。
Google Cloud コンソールで構成設定を更新する手順は次のとおりです。
Google Cloud コンソールで、メニュー アイコン > [その他のプロダクト] > [Google Workspace] > [プロダクト ライブラリ] > [Google Chat API] に移動します。
[管理]、[構成] タブの順にクリックします。
[インタラクティブ機能] の [機能] セクションに移動して、アプリのホームを構成します。
- [1 対 1 のメッセージを受信する] チェックボックスをオンにします。
- [App Home をサポートする] チェックボックスをオンにします。
Chat アプリが HTTP サービスを使用している場合は、[接続設定] に移動し、[アプリのホームページ URL] フィールドにエンドポイントを指定します。[HTTP エンドポイント URL] フィールドで指定した URL を使用できます。
[保存] をクリックします。
アプリのホームカードを作成する
ユーザーがアプリのホームを開いたとき、Chat アプリは、pushCard
ナビゲーションと Card
を使用して RenderActions
のインスタンスを返すことにより、APP_HOME
インタラクション イベントを処理する必要があります。インタラクティブなエクスペリエンスを作成するには、カードにボタンやテキスト入力などのインタラクティブなウィジェットを含めることができます。Chat アプリは、これらのウィジェットを処理して、追加のカードやダイアログで応答できます。
次の例では、Chat アプリの最初のアプリのホームカードに、カードの作成日時とボタンが表示されています。ユーザーがボタンをクリックすると、更新されたカードが返され、更新されたカードの作成時間が表示されます。
Node.js
Python
Java
Apps Script
すべての APP_HOME
インタラクション イベントの後に呼び出される onAppHome
関数を実装します。
この例では、カード JSON を返すことでカード メッセージを送信します。Apps Script カードサービスを使用することもできます。
アプリのホーム画面の操作に応答する
最初のアプリのホームカードにボタンや選択入力などのインタラクティブなウィジェットが含まれている場合、Chat アプリは updateCard
ナビゲーションを使用して RenderActions
のインスタンスを返すことで、関連するインタラクション イベントを処理する必要があります。インタラクティブ ウィジェットの処理の詳細については、ユーザーが入力した情報を処理するをご覧ください。
上の例では、最初のアプリのホームカードにボタンが含まれていました。ユーザーがボタンをクリックするたびに、CARD_CLICKED
インタラクション イベントが関数 updateAppHome
をトリガーして、アプリのホームカードを更新します。次のコードをご覧ください。
Node.js
Python
Java
Apps Script
この例では、カード JSON を返すことでカード メッセージを送信します。Apps Script カードサービスを使用することもできます。
ダイアログを開く
Chat アプリは、ダイアログを開いて、アプリのホームでの操作に応答することもできます。
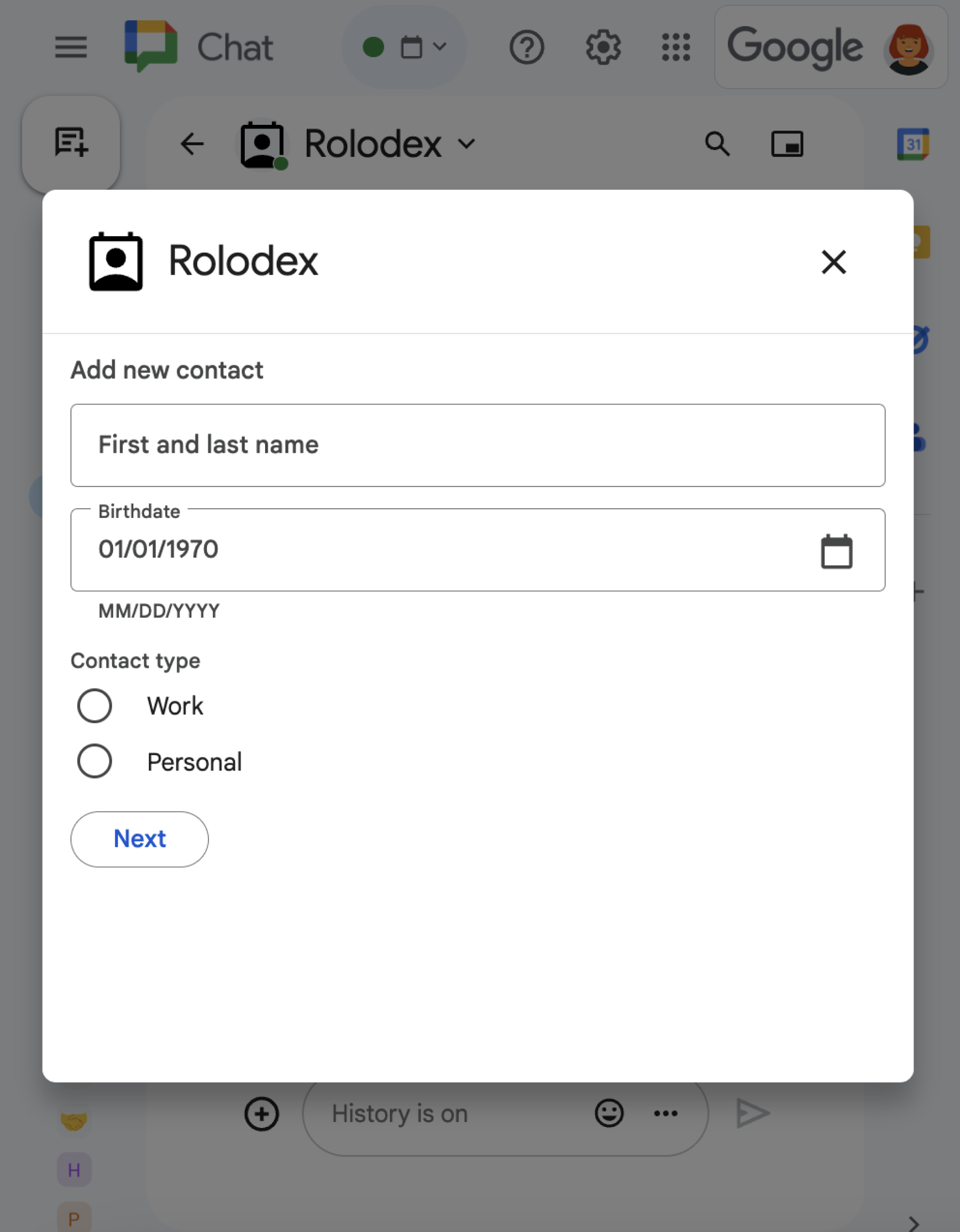
アプリのホームからダイアログを開くには、Card
オブジェクトを含む updateCard
ナビゲーションで renderActions
を返すことにより、関連する操作イベントを処理します。次の例では、CARD_CLICKED
インタラクション イベントを処理してダイアログを開くことで、アプリのホームカードからのボタンクリックに Chat アプリが応答します。
{ renderActions: { action: { navigations: [{ updateCard: { sections: [{
header: "Add new contact",
widgets: [{ "textInput": {
label: "Name",
type: "SINGLE_LINE",
name: "contactName"
}}, { textInput: {
label: "Address",
type: "MULTIPLE_LINE",
name: "address"
}}, { decoratedText: {
text: "Add to favorites",
switchControl: {
controlType: "SWITCH",
name: "saveFavorite"
}
}}, { decoratedText: {
text: "Merge with existing contacts",
switchControl: {
controlType: "SWITCH",
name: "mergeContact",
selected: true
}
}}, { buttonList: { buttons: [{
text: "Next",
onClick: { action: { function: "openSequentialDialog" }}
}]}}]
}]}}]}}}
ダイアログを閉じるには、次のインタラクション イベントを処理します。
CLOSE_DIALOG
: ダイアログを閉じて、Chat アプリの最初のアプリのホームカードに戻ります。CLOSE_DIALOG_AND_EXECUTE
: ダイアログを閉じて、アプリのホームカードを更新します。
次のコードサンプルでは、CLOSE_DIALOG
を使用してダイアログを閉じて、アプリのホームカードに戻ります。
{ renderActions: { action: {
navigations: [{ endNavigation: { action: "CLOSE_DIALOG" }}]
}}}
ユーザーから情報を収集するには、連続的なダイアログを作成することもできます。連続したダイアログを作成する方法については、ダイアログを開いて応答するをご覧ください。
関連トピック
- アプリのホームを使用する Chat アプリのサンプルを確認する。
- ダイアログを開いて応答する。