This page explains how to set up and respond to slash commands for your Google Chat app.
A slash command is a common way that users invoke and interact with a Chat app. Slash commands also help users discover and use key features of a Chat app.
To use a slash command, users type a slash (/
) and then a short text command,
such as /about
to get information about the Chat app.
Users can discover available slash commands by typing a slash into
Google Chat, which displays a window that lists the available commands for the
Chat app:
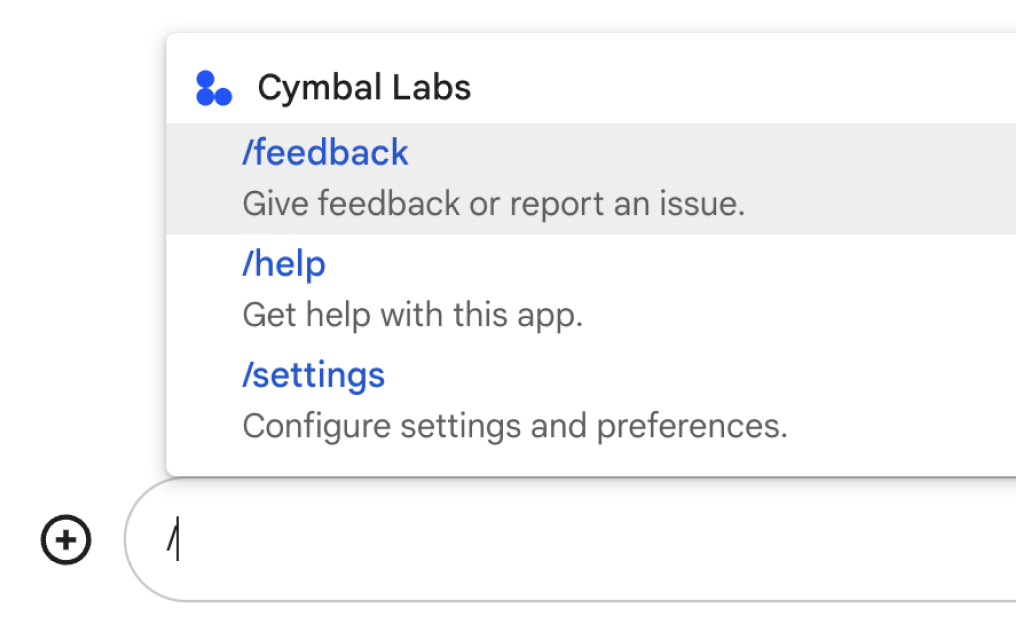
To decide whether you should set up slash commands, and to understand how to design user interactions, see Define all user journeys.
Slash commands with private responses
When a user sends a message that contains a slash command, the message is only visible to the user and the Chat app. If you've configured your Chat app to be added to spaces with multiple people, you might consider responding to the slash command privately, to keep the interaction private between the user and the Chat app.
For example, to learn about a Chat app that they
discover in a space, users could use commands such as /about
or /help
.
To avoid notifying everyone else in the space, the
Chat app can respond privately with information about
how to use the Chat app and get support.
Prerequisites
Node.js
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Apps Script
A Google Chat app that's enabled for interactive features. To create an interactive Chat app in Apps Script, complete this quickstart.
Python
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Java
A Google Chat app that's enabled for interactive features. To create an interactive Chat app using an HTTP service, complete this quickstart.
Set up a slash command
This section explains how to complete the following steps to set up a slash command:
- Create a name for your slash command.
- Configure the slash command in the Google Chat API.
Name your slash command
The name of a slash command is what users type in a Chat message to invoke the Chat app. A short description also appears below the name, to prompt users further about how to use the command:
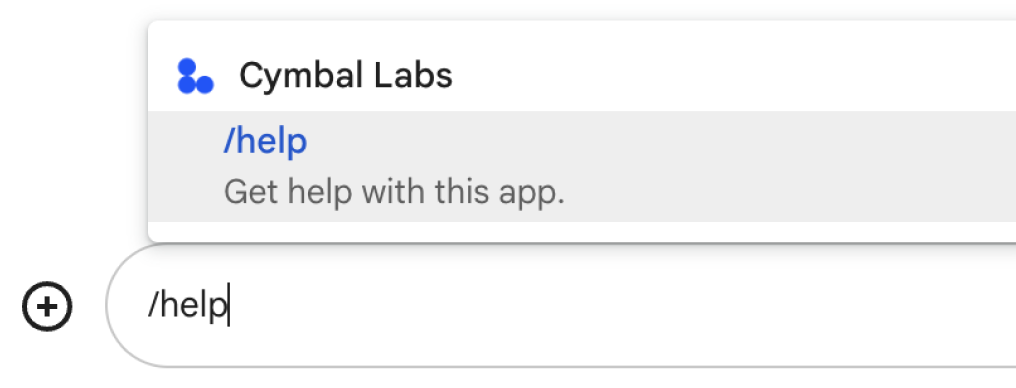
When choosing a name and description for your slash command, consider the following recommendations:
To name your slash command:
- Use short, descriptive, and actionable words or phrases to make the
commands clear and simple to the user. For example, instead of saying
/createAReminder
, use/remindMe
. - If your command contains more than one word, help users read the command
by using all lowercase for the first word and then capitalizing the first
letter of additional words. For example, instead of
/updatecontact
, use/updateContact
. - Consider whether to use a unique or common name for your command. If
your command describes a typical interaction or feature, you can use a
common name that users recognize and expect, such as
/settings
or/feedback
. Otherwise, try to use unique command names, because if your command name is the same for other Chat apps, the user must filter through similar commands to find and use yours.
- Use short, descriptive, and actionable words or phrases to make the
commands clear and simple to the user. For example, instead of saying
To describe your slash command:
- Keep the description short and clear so that users know what to expect when they invoke the command.
- Let users know if there are any formatting requirements for the command.
For example, if you create a
/remindMe
command that requires argument text, set the description to something likeRemind me to do [something] at [time]
. - Let users know if the Chat app replies to
everyone in the space, or privately to the user who invokes the command.
For example, for the slash command
/about
, you could describe it asLearn about this app (Only visible to you)
. To respond privately to a slash command, see the section Respond with a private message.
Configure your slash command in the Google Chat API
To create a slash command, you need to specify information about the command in your Chat app's configuration for the Google Chat API.
To configure a slash command in the Google Chat API, complete the following steps:
In the Google Cloud console, click Menu > APIs & Services > Enabled APIs & Services > Google Chat API
Click Configuration.
Under Slash commands, click Add a slash command.
Enter a name, command ID, and description for the command:
- Name: the display name for the command, and what users type to invoke your app. Must start with a slash, contain only text, and can be up to 50 characters.
- Description: the text that describes how to use and format the command. Descriptions can be up to 50 characters.
- Command ID: a number from 1 to 1000 that your Chat app uses to recognize the slash command and return a response.
Optional: If you want your Chat app to respond to the command with a dialog, select the Open a dialog checkbox.
Click Save.
The slash command is now configured for the Chat app.
Respond to a slash command
When users create a Chat message that contains a slash command,
your Chat app receives a MESSAGE
interaction event.
The event payload contains the slashCommand
and slashCommandMetadata
objects. These objects contain details about the command used in the message
(including the command ID), so that you can return an appropriate response.

/help
to explain how to get support.The following code shows an example of a Chat app
that replies to the /about
slash command by handling
MESSAGE
interaction events and detecting
whether the message contains the matching command ID. If the message contains
the command ID, the Chat app returns a private message:
Node.js
Apps Script
Python
Java
Set ABOUT_COMMAND_ID
with the command ID that you
specified when you
configured the slash command in the Chat API.
To test this code, see
Test interactive features for Google Chat apps.
Related topics
- View Chat app samples that use slash commands
- Send a message
- Send private messages
- Open interactive dialogs