Questa pagina descrive in che modo l'app Chat può aprire finestre di dialogo per rispondere agli utenti.
Le finestre di dialogo sono interfacce basate su schede con finestre che si aprono da uno spazio di Chat o da un messaggio. La finestra di dialogo e i relativi contenuti sono visibili solo all'utente che l'ha aperta.
Le app di chat possono utilizzare le finestre di dialogo per richiedere e raccogliere informazioni dagli utenti di Chat, inclusi i moduli in più passaggi. Per maggiori dettagli sulla creazione di input dei moduli, vedi Raccogliere ed elaborare informazioni dagli utenti.
Prerequisiti
Node.js
Un'app Google Chat per cui sono abilitate le funzionalità interattive. Per creare un'app Chat interattiva utilizzando un servizio HTTP, completa questa guida rapida.
Python
Un'app Google Chat per cui sono abilitate le funzionalità interattive. Per creare un'app Chat interattiva utilizzando un servizio HTTP, completa questa guida rapida.
Java
Un'app Google Chat per cui sono abilitate le funzionalità interattive. Per creare un'app Chat interattiva utilizzando un servizio HTTP, completa questa guida rapida.
Apps Script
Un'app Google Chat per cui sono abilitate le funzionalità interattive. Per creare un'app Chat interattiva in Apps Script, completa questa guida rapida.
Apri una finestra di dialogo
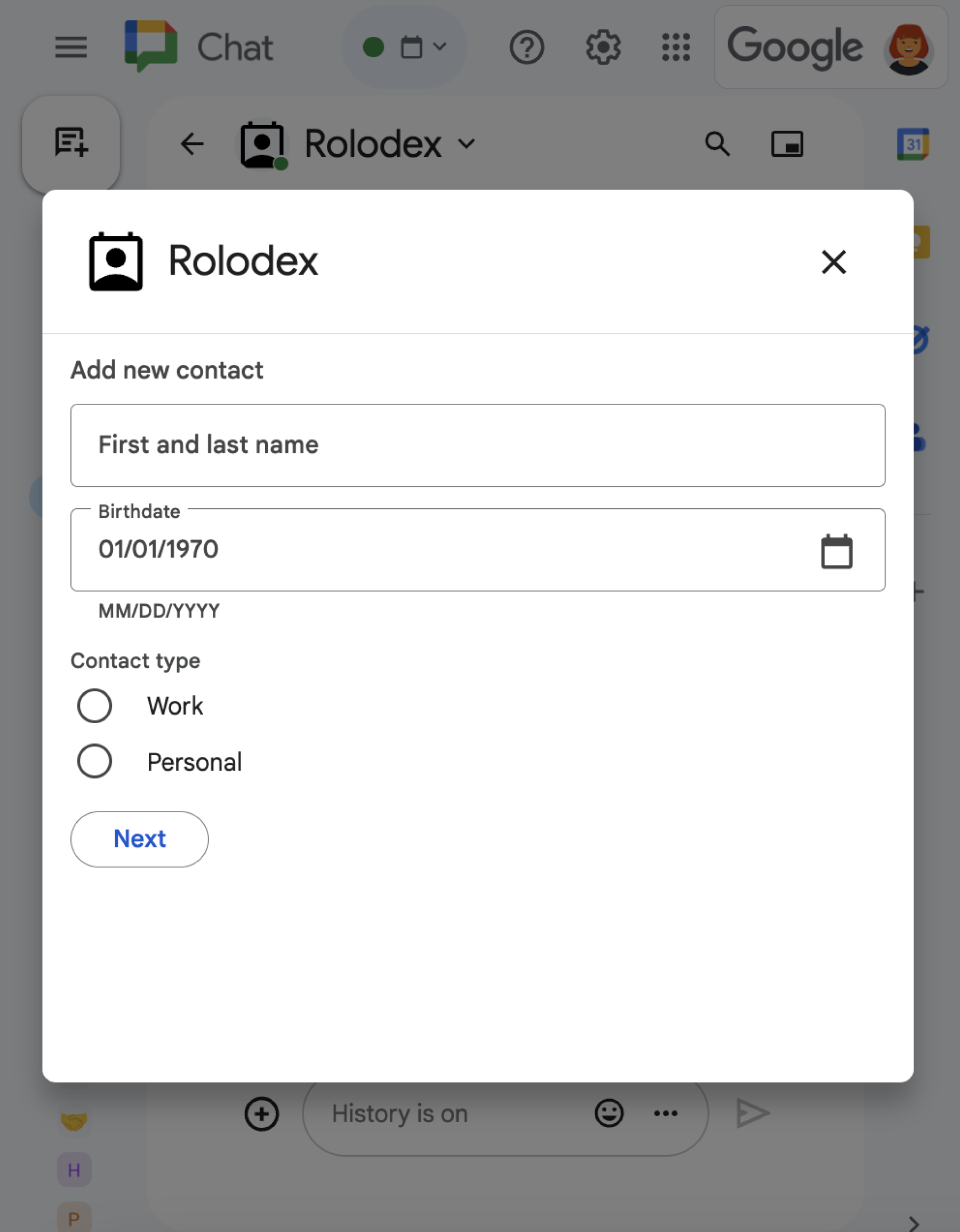
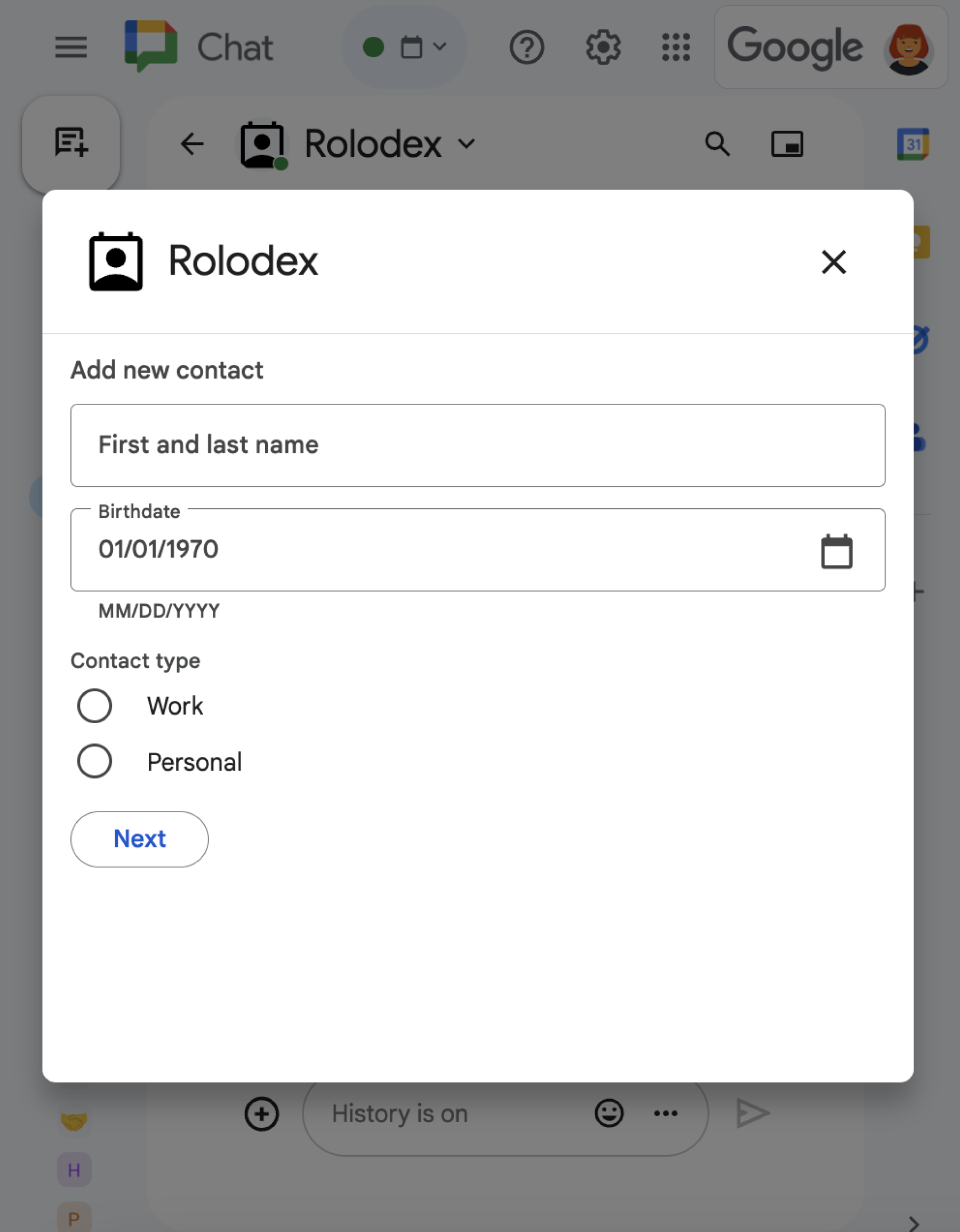
Questa sezione spiega come rispondere e configurare un dialogo nel seguente modo:
- Attiva la richiesta di dialogo da un'interazione dell'utente.
- Gestisci la richiesta restituendo un valore e aprendo una finestra di dialogo.
- Dopo che gli utenti hanno inviato le informazioni, elabora l'invio chiudendo la finestra di dialogo o restituendo un'altra finestra di dialogo.
Attivare una richiesta di dialogo
Un'app di chat può aprire finestre di dialogo solo per rispondere a un'interazione dell'utente, ad esempio un comando con barra o un clic su un pulsante da un messaggio in una scheda.
Per rispondere agli utenti con una finestra di dialogo, un'app di chat deve creare un'interazione che attivi la richiesta di dialogo, ad esempio:
- Rispondere a un comando slash. Per attivare la richiesta da un comando con barra, devi selezionare la casella di controllo Apre una finestra di dialogo durante la configurazione del comando.
- Rispondi a un clic su un pulsante in un
messaggio,
all'interno di una scheda o nella parte inferiore del messaggio. Per attivare la richiesta da un pulsante in un messaggio, configura l'azione
onClick
del pulsante impostandointeraction
suOPEN_DIALOG
. - Rispondere a un clic sul pulsante nella home page di un'app Chat. Per scoprire come aprire le finestre di dialogo dalle home page, consulta Creare una home page per l'app Google Chat.
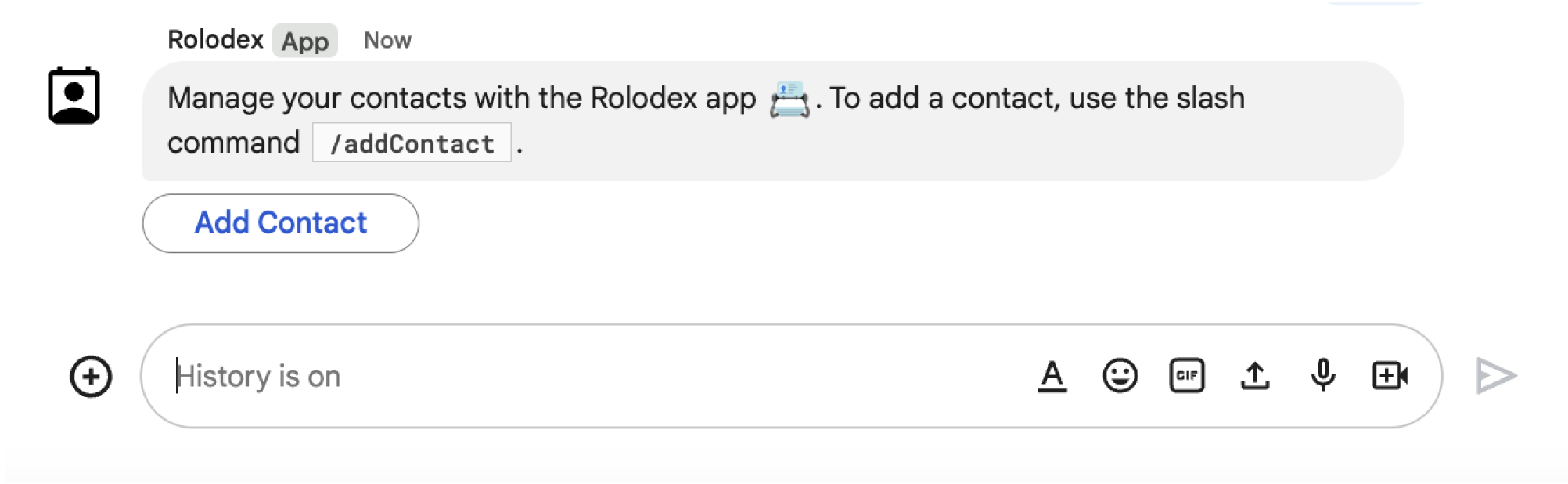
/addContact
. Il messaggio include anche un pulsante su cui gli utenti possono fare clic per attivare il comando.
Il seguente esempio di codice mostra come attivare una richiesta di dialogo da un pulsante in un messaggio della scheda. Per aprire la finestra di dialogo, il campo button.interaction
è impostato su OPEN_DIALOG
:
Node.js
Python
Java
Apps Script
Questo esempio invia un messaggio di una scheda restituendo card JSON. Puoi anche utilizzare il servizio di schede di Apps Script.
Apri la finestra di dialogo iniziale
Quando un utente attiva una richiesta di dialogo, l'app Chat riceve un evento di interazione, rappresentato come tipo event
nell'API Chat. Se l'interazione attiva una richiesta di dialogo, il campo dialogEventType
dell'evento viene impostato su REQUEST_DIALOG
.
Per aprire una finestra di dialogo, l'app Chat può rispondere alla richiesta restituendo un oggetto actionResponse
con type
impostato su DIALOG
e l'oggetto Message
. Per specificare i contenuti della finestra di dialogo, includi i seguenti oggetti:
- Un oggetto
actionResponse
contype
impostato suDIALOG
. - Un oggetto
dialogAction
. Il campobody
contiene gli elementi dell'interfaccia utente (UI) da visualizzare nella scheda, inclusi uno o piùsections
di widget. Per raccogliere informazioni dagli utenti, puoi specificare widget di input dei moduli e un widget di pulsante. Per scoprire di più sulla progettazione degli input per i moduli, consulta Raccogliere ed elaborare informazioni dagli utenti.
Il seguente esempio di codice mostra in che modo un'app di Chat restituisce una risposta che apre una finestra di dialogo:
Node.js
Python
Java
Apps Script
Questo esempio invia un messaggio di una scheda restituendo card JSON. Puoi anche utilizzare il servizio di schede Apps Script.
Gestire l'invio della finestra di dialogo
Quando gli utenti fanno clic su un pulsante che invia una finestra di dialogo, la tua app di chat riceve un evento di interazione CARD_CLICKED
in cui dialogEventType
è SUBMIT_DIALOG
.
L'app Chat deve gestire l'evento di interazione in uno dei seguenti modi:
- Ritorna a un'altra finestra di dialogo per compilare un'altra scheda o un altro modulo.
- Chiudi la finestra di dialogo dopo aver convalidato i dati inviati dall'utente e, facoltativamente, invia un messaggio di conferma.
(Facoltativo) Restituire un'altra finestra di dialogo
Dopo che gli utenti hanno inviato la finestra di dialogo iniziale, le app di chat possono restituire una o più finestre di dialogo aggiuntive per aiutare gli utenti a esaminare le informazioni prima di inviarle, a completare i moduli in più passaggi o a compilare i contenuti in modo dinamico.
Per elaborare i dati inseriti dagli utenti, l'app di Chat utilizza l'oggetto event.common.formInputs
. Per scoprire di più sul recupero dei valori dai widget di input, consulta
Raccogliere ed elaborare le informazioni degli utenti.
Per tenere traccia dei dati inseriti dagli utenti nella finestra di dialogo iniziale, devi aggiungere parametri al pulsante che apre la finestra di dialogo successiva. Per maggiori dettagli, consulta Trasferire i dati su un'altra scheda.
In questo esempio, un'app di chat apre una finestra di dialogo iniziale che porta a una seconda finestra di dialogo per la conferma prima dell'invio:
Node.js
Python
Java
Apps Script
Questo esempio invia un messaggio della scheda restituendo JSON della scheda. Puoi anche utilizzare il servizio di schede Apps Script.
Chiudi la finestra di dialogo
Quando gli utenti fanno clic su un pulsante in una finestra di dialogo, la tua app Chat esegue l'azione associata e fornisce all'oggetto evento le seguenti informazioni:
eventType
èCARD_CLICKED
.dialogEventType
èSUBMIT_DIALOG
.
L'app Chat deve restituire un
oggetto ActionResponse
con type
impostato su DIALOG
e dialogAction
.
(Facoltativo) Visualizzare una notifica
Quando chiudi la finestra di dialogo, puoi anche visualizzare una notifica di testo.
L'app Chat può rispondere con una notifica di esito positivo o di errore restituendo un messaggio ActionResponse
con actionStatus
impostato.
L'esempio seguente verifica che i parametri siano validi e chiude la finestra di dialogo con una notifica di testo in base al risultato:
Node.js
Python
Java
Apps Script
Questo esempio invia un messaggio della scheda restituendo JSON della scheda. Puoi anche utilizzare il servizio di schede di Apps Script.
Per informazioni dettagliate sul passaggio dei parametri tra le finestre di dialogo, consulta Trasferire i dati a un'altra scheda.
(Facoltativo) Invia un messaggio di conferma
Quando chiudi la finestra di dialogo, puoi anche inviare un nuovo messaggio o aggiornarne uno esistente.
Per inviare un nuovo messaggio, restituisci un oggetto
ActionResponse
con type
impostato su NEW_MESSAGE
. Nell'esempio seguente, la finestra di dialogo con notifica di testo e messaggio di conferma viene chiusa:
Node.js
Python
Java
Apps Script
Questo esempio invia un messaggio della scheda restituendo JSON della scheda. Puoi anche utilizzare il servizio di schede di Apps Script.
Per aggiornare un messaggio, restituisci un oggetto actionResponse
contenente il messaggio aggiornato e imposta type
su uno dei seguenti valori:
UPDATE_MESSAGE
: aggiorna il messaggio che ha attivato la richiesta di dialogo.UPDATE_USER_MESSAGE_CARDS
: aggiorna la scheda da un'anteprima del link.
Risoluzione dei problemi
Quando un'app o una scheda Google Chat restituisce un errore, l'interfaccia di Chat mostra il messaggio "Si è verificato un problema". o "Impossibile elaborare la tua richiesta". A volte l'interfaccia utente di Chat non mostra alcun messaggio di errore, ma l'app Chat o la scheda produce un risultato imprevisto; ad esempio, un messaggio della scheda potrebbe non essere visualizzato.
Sebbene un messaggio di errore potrebbe non essere visualizzato nell'interfaccia utente di Chat, sono disponibili messaggi di errore descrittivi e dati di log per aiutarti a correggere gli errori quando la registrazione degli errori per le app Chat è attivata. Per assistenza su come visualizzare, eseguire il debug e correggere gli errori, consulta la sezione Risolvere gli errori di Google Chat.
Argomenti correlati
- Visualizza l'esempio di Gestore contatti, che è un'app di chat che utilizza le finestre di dialogo per raccogliere i dati di contatto.
- Apri le finestre di dialogo dalla home page dell'app Google Chat.
- Configurare e rispondere ai comandi slash
- Elaborare le informazioni inserite dagli utenti