本指南介绍如何调用 Google Chat API 的
messages.create()
方法执行以下任何操作:
- 发送包含文本、卡片和互动微件的消息。
- 向特定 Chat 用户私下发送消息。
- 发起或回复消息串。
- 为消息命名,以便在其他 Chat API 中指定 请求。
<ph type="x-smartling-placeholder">
邮件(包括任何文字或卡片)的大小上限为 32,000 字节。 如要发送超出此大小的消息,您的 Chat 扩展应用 必须改为发送多条消息。
除了调用 messages.create()
方法之外,Chat 扩展应用
可以创建和发送消息来回复用户互动,如发布
用户在将 Chat 应用添加到
空间。在响应互动时,Chat 扩展应用可以使用
消息功能的类型,包括互动对话框和链接预览
界面。为了回复用户,Chat 应用会返回
无需调用 Chat API 即可同步处理消息。学习内容
有关如何发送消息来响应互动的信息,请参阅
在 Google Chat 应用中接收和回复互动信息。
Chat 如何显示和归因使用 Chat API 创建的消息
您可以使用以下代码调用 messages.create()
方法:
应用身份验证
和用户身份验证。
Chat 对消息发送者的界定有所不同
具体取决于您使用的身份验证类型。
以 Chat 应用的身份进行身份验证时, Chat 应用发送消息。
<ph type="x-smartling-placeholder">App
。您作为用户进行身份验证后,Chat 应用会将 。Chat 还会将 Chat 应用通过显示消息名称来发送消息。
<ph type="x-smartling-placeholder">身份验证类型还决定了哪些消息传递功能和界面 您可以包含在消息中的名称借助应用身份验证 聊天应用可以发送包含富文本、 基于卡片的界面和交互式小部件。 由于 Chat 用户只能在消息中发送短信,因此您可以 使用用户身份验证创建邮件时仅包含文本。 详细了解消息功能 功能的详细信息,请参阅 Google Chat 消息概览。
本指南介绍了如何使用这两种身份验证类型来发送邮件 使用 Chat API。
前提条件
Node.js
- Business 或 Enterprise 有权访问以下内容的 Google Workspace 账号: Google Chat。
- 设置您的环境:
<ph type="x-smartling-placeholder">
- </ph>
- 创建 Google Cloud 项目。
- 配置 OAuth 同意屏幕。
- 启用并配置 Google Chat API,指定一个名称, 图标和说明。
- 安装 Node.js Cloud 客户端库。
- 根据您希望在 Google Chat API 中进行身份验证的方式创建访问凭据
请求:
<ph type="x-smartling-placeholder">
- </ph>
- 如需以 Chat 用户身份进行身份验证,请按以下步骤操作:
创建 OAuth 客户端 ID
凭据,然后将凭据保存为名为
client_secrets.json
复制到您的本地目录。 - 如需以 Chat 应用的身份进行身份验证,请执行以下操作:
创建服务账号
凭据,然后将凭据保存为名为
credentials.json
。
- 如需以 Chat 用户身份进行身份验证,请按以下步骤操作:
创建 OAuth 客户端 ID
凭据,然后将凭据保存为名为
- <ph type="x-smartling-placeholder"></ph> 选择授权范围取决于您是想以用户身份还是 Chat 应用。
- 一个 Google Chat 聊天室,其中经过身份验证的用户或 呼叫 Chat 应用是成员。要以 聊天应用,请添加 Chat 扩展应用。
Python
- Business 或 Enterprise 有权访问以下内容的 Google Workspace 账号: Google Chat。
- 设置您的环境:
<ph type="x-smartling-placeholder">
- </ph>
- 创建 Google Cloud 项目。
- 配置 OAuth 同意屏幕。
- 启用并配置 Google Chat API,指定一个名称, 图标和说明。
- 安装 Python Cloud 客户端库。
- 根据您希望在 Google Chat API 中进行身份验证的方式创建访问凭据
请求:
<ph type="x-smartling-placeholder">
- </ph>
- 如需以 Chat 用户身份进行身份验证,请按以下步骤操作:
创建 OAuth 客户端 ID
凭据,然后将凭据保存为名为
client_secrets.json
复制到您的本地目录。 - 如需以 Chat 应用的身份进行身份验证,请执行以下操作:
创建服务账号
凭据,然后将凭据保存为名为
credentials.json
。
- 如需以 Chat 用户身份进行身份验证,请按以下步骤操作:
创建 OAuth 客户端 ID
凭据,然后将凭据保存为名为
- <ph type="x-smartling-placeholder"></ph> 选择授权范围取决于您是想以用户身份还是 Chat 应用。
- 一个 Google Chat 聊天室,其中经过身份验证的用户或 呼叫 Chat 应用是成员。要以 聊天应用,请添加 Chat 扩展应用。
Java
- Business 或 Enterprise 有权访问以下内容的 Google Workspace 账号: Google Chat。
- 设置您的环境:
<ph type="x-smartling-placeholder">
- </ph>
- 创建 Google Cloud 项目。
- 配置 OAuth 权限请求页面。
- 启用并配置 Google Chat API,指定一个名称, 图标和说明。
- 安装 Java Cloud 客户端库。
- 根据您希望在 Google Chat API 中进行身份验证的方式创建访问凭据
请求:
<ph type="x-smartling-placeholder">
- </ph>
- 如需以 Chat 用户身份进行身份验证,请按以下步骤操作:
创建 OAuth 客户端 ID
凭据,然后将凭据保存为名为
client_secrets.json
复制到您的本地目录。 - 如需以 Chat 应用的身份进行身份验证,请执行以下操作:
创建服务账号
凭据,然后将凭据保存为名为
credentials.json
。
- 如需以 Chat 用户身份进行身份验证,请按以下步骤操作:
创建 OAuth 客户端 ID
凭据,然后将凭据保存为名为
- <ph type="x-smartling-placeholder"></ph> 选择授权范围取决于您是想以用户身份还是 Chat 应用。
- 一个 Google Chat 聊天室,其中经过身份验证的用户或 呼叫 Chat 应用是成员。要以 聊天应用,请添加 Chat 扩展应用。
Apps 脚本
- Business 或 Enterprise 有权访问以下内容的 Google Workspace 账号: Google Chat。
- 设置您的环境:
<ph type="x-smartling-placeholder">
- </ph>
- 创建 Google Cloud 项目。
- 配置 OAuth 同意屏幕。
- 启用并配置 Google Chat API,指定一个名称, 图标和说明。
- 创建一个独立的 Apps 脚本项目, 然后启用高级聊天服务。
- 在本指南中,您必须使用用户 或应用身份验证。如需以 Chat 应用的身份进行身份验证,请创建 服务账号凭据。有关具体步骤,请参阅 以用户身份进行身份验证和授权 Google Chat 应用。
- <ph type="x-smartling-placeholder"></ph> 选择授权范围取决于您是想以用户身份还是 Chat 应用。
- 一个 Google Chat 聊天室,其中经过身份验证的用户或 呼叫 Chat 应用是成员。要以 聊天应用,请添加 Chat 扩展应用。
以 Chat 应用的身份发送消息
本部分介绍如何发送包含文本、卡片和 交互式配件微件 应用身份验证。
若要使用应用身份验证调用 messages.create()
,您必须指定
以下字段:
chat.bot
授权范围。Space
资源 。Chat 应用必须 聊天室成员。Message
要创建的资源。要定义消息的内容,您可以在 富文本 (text
), 一个或多个卡片接口 (cardsV2
), 或者两者兼有
您还可以选择添加以下内容:
- 要包含的
accessoryWidgets
字段 邮件底部的互动按钮。 privateMessageViewer
字段 向指定用户以私密方式发送消息。messageId
字段,可让您 为消息命名以便在其他 API 请求中使用。thread.threadKey
和messageReplyOption
字段 发起或回复讨论帖。如果聊天室没有 使用线程处理,系统会忽略此字段。
以下代码展示了 Chat 应用如何 可以发送通过 Chat 应用发布的消息,消息中包含以下内容: 文本、卡片以及消息底部的可点击按钮:
Node.js
Python
Java
Apps 脚本
如需运行此示例,请将 SPACE_NAME
替换为
空间的
name
字段。
可通过调用
spaces.list()
方法
或者通过聊天室网址添加
在邮件底部添加互动微件
在本指南的第一个代码示例中, Chat 应用消息在 称为配件微件。配件微件 。您可以使用这些微件 用户可通过多种方式与您的消息互动,包括:
- 对消息的准确性或满意度进行评分。
- 报告消息或 Chat 应用存在的问题。
- 打开指向相关内容(例如文档)的链接。
- 在 Chat 应用中关闭或延后类似消息 特定时间段内的效果
如需添加配件微件,请添加
accessoryWidgets[]
字段,然后指定所需的一个或多个微件
。
下图显示了将 包含配件微件的短信,以便用户对自己的体验进行评分 使用 Chat 应用。
<ph type="x-smartling-placeholder">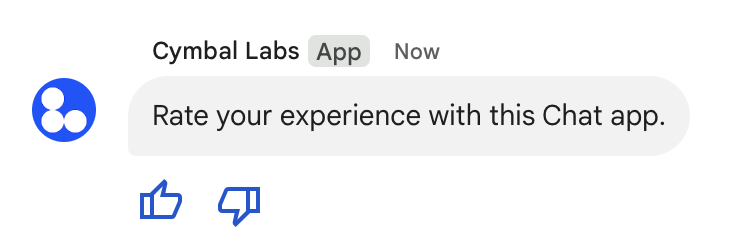
下面显示了使用
两个配件按钮。当用户点击某个按钮时,
函数(例如 doUpvote
)处理交互:
{
text: "Rate your experience with this Chat app.",
accessoryWidgets: [{ buttonList: { buttons: [{
icon: { material_icon: {
name: "thumb_up"
}},
color: { red: 0, blue: 255, green: 0 },
onClick: { action: {
function: "doUpvote"
}}
}, {
icon: { material_icon: {
name: "thumb_down"
}},
color: { red: 0, blue: 255, green: 0 },
onClick: { action: {
function: "doDownvote"
}}
}]}}]
}
私下发送消息
聊天应用可以私下发送消息 消息仅对聊天室中的特定用户可见。当 聊天应用会发送一条私信 会显示一个标签,通知用户相应邮件仅对他们可见。
要使用 Chat API 以私密方式发送消息,请指定
privateMessageViewer
字段。要指定用户,请将值设置为
User
资源,
代表 Chat 用户。您还可以使用
name
字段的
User
资源,如以下示例所示:
{
text: "Hello private world!",
privateMessageViewer: {
name: "users/USER_ID"
}
}
如需使用此示例,请将 USER_ID
用户的唯一 ID,例如 12345678987654321
或
hao@cymbalgroup.com
。如需详细了解如何指定用户,请参阅
识别并指定 Google Chat 用户。
要以私密方式发送消息,您必须在请求中省略以下内容:
代表用户发送短信
本部分介绍了如何使用 用户身份验证。 借助用户身份验证,邮件内容只能包含文本 并且必须省略仅适用于 聊天应用,包括卡片界面和交互式微件。
若要使用用户身份验证调用 messages.create()
,您必须指定
以下字段:
- 授权范围
此方法支持用户身份验证。以下示例使用
chat.messages.create
范围。 Space
资源 。通过身份验证的用户必须是 空间。Message
要创建的资源。要定义消息的内容,您必须在代码中添加text
字段。
您还可以选择添加以下内容:
messageId
字段,可让您 为消息命名以便在其他 API 请求中使用。thread.threadKey
和messageReplyOption
字段 发起或回复讨论帖。如果聊天室没有 使用线程处理,系统会忽略此字段。
以下代码展示了 Chat 应用如何 可以代表经过身份验证的用户在指定空间中发送短信:
Node.js
Python
Java
Apps 脚本
如需运行此示例,请将 SPACE_NAME
替换为
空间的
name
字段。可通过调用
spaces.list()
方法
或者通过聊天室网址添加
在消息串中发起或回复消息
对于使用话题的聊天室: 您可以指定新消息是发起会话还是回复 现有线程
默认情况下,您使用 Chat API 创建的消息会发起新的 线程。为方便识别该会话并稍后回复,您可以指定一个 线程键:
- 在请求正文中,指定
thread.threadKey
字段。 - 指定查询参数
messageReplyOption
以确定如果该键已存在会发生什么情况。
如需创建回复现有会话的消息,请执行以下操作:
以下代码展示了 Chat 应用如何 可以发送短信,而相应短信会发起或回复由 键:
Node.js
Python
Java
Apps 脚本
如需运行此示例,请替换以下内容:
THREAD_KEY
:聊天室中的现有消息串键,或 来创建新线程,这是线程的唯一名称。SPACE_NAME
:聊天室的 IDname
字段。可通过调用spaces.list()
方法 或者通过聊天室网址添加
为消息命名
如需在将来的 API 调用中检索或指定消息,您可以为消息命名
方法是在 messages.create()
请求中设置 messageId
字段。
通过为消息命名,您可以指定消息,而无需存储
消息的资源名称中系统分配的 ID(以
name
字段)。
例如,如需使用 get()
方法检索消息,您可以使用
资源名称指定要检索的消息。资源名称为
格式为 spaces/{space}/messages/{message}
,其中 {message}
表示
系统分配的 ID 或您在创建
消息。
要命名消息,请在
messageId
字段。messageId
字段用于设置
clientAssignedMessageId
Message
资源的指定字段。
您只能在创建消息时为其命名。您不能命名或 修改现有消息的自定义 ID。自定义 ID 必须符合以下要求 要求:
- 以
client-
开头。例如,client-custom-name
是有效的自定义 但custom-name
不是。 - 最多包含 63 个字符,且只能包含小写字母、数字和 连字符。
- 在聊天室中是唯一的。Chat 应用无法使用 为不同的消息使用相同的自定义 ID。
以下代码展示了 Chat 应用如何 可以代表 经过身份验证的用户:
Node.js
Python
Java
Apps 脚本
如需运行此示例,请替换以下内容:
SPACE_NAME
:聊天室的 IDname
字段。可通过调用spaces.list()
方法 或者通过聊天室网址添加MESSAGE-ID
:以消息开头的消息的名称 和custom-
。该 ID 必须与 指定聊天室中的 Chat 应用。
问题排查
当 Google Chat 应用或 card 会返回错误, 聊天界面会显示一条内容为“出了点问题”的消息。 或“无法处理您的请求”。有时,Chat 界面 不会显示任何错误消息,但 Chat 应用或 卡片会产生意外结果;例如,卡片消息 。
虽然 Chat 界面中可能不会显示错误消息, 提供描述性错误消息和日志数据,以帮助您修正错误 启用 Chat 应用的错误日志记录时。如需观看方面的帮助, 请参阅 排查并修正 Google Chat 错误。
相关主题
- 使用卡片制作工具 设计和预览 Chat 应用的 JSON 卡片消息。
- 设置邮件格式。
- 获取消息的详细信息。
- 在聊天室中列出消息。
- 更新消息。
- 删除消息。
- 在 Google Chat 消息中识别用户。
- 使用传入的网络钩子向 Google Chat 发送消息。