Halaman ini menjelaskan cara aplikasi Chat Anda membuka dialog untuk merespons pengguna.
Dialog adalah antarmuka berbasis kartu yang berjendela yang terbuka dari ruang atau pesan Chat. Dialog dan kontennya hanya dapat dilihat oleh pengguna yang membukanya.
Aplikasi Chat dapat menggunakan dialog untuk meminta dan mengumpulkan informasi dari pengguna Chat, termasuk formulir multi-langkah. Untuk mengetahui detail selengkapnya tentang cara membuat input formulir, lihat Mengumpulkan dan memproses informasi dari pengguna.
Prasyarat
Node.js
Aplikasi Google Chat yang diaktifkan untuk fitur interaktif. Untuk membuat aplikasi Chat interaktif menggunakan layanan HTTP, selesaikan panduan memulai ini.
Python
Aplikasi Google Chat yang diaktifkan untuk fitur interaktif. Untuk membuat aplikasi Chat interaktif menggunakan layanan HTTP, selesaikan panduan memulai ini.
Java
Aplikasi Google Chat yang diaktifkan untuk fitur interaktif. Untuk membuat aplikasi Chat interaktif menggunakan layanan HTTP, selesaikan panduan memulai ini.
Apps Script
Aplikasi Google Chat yang diaktifkan untuk fitur interaktif. Untuk membuat aplikasi Chat interaktif di Apps Script, selesaikan panduan memulai ini.
Membuka dialog
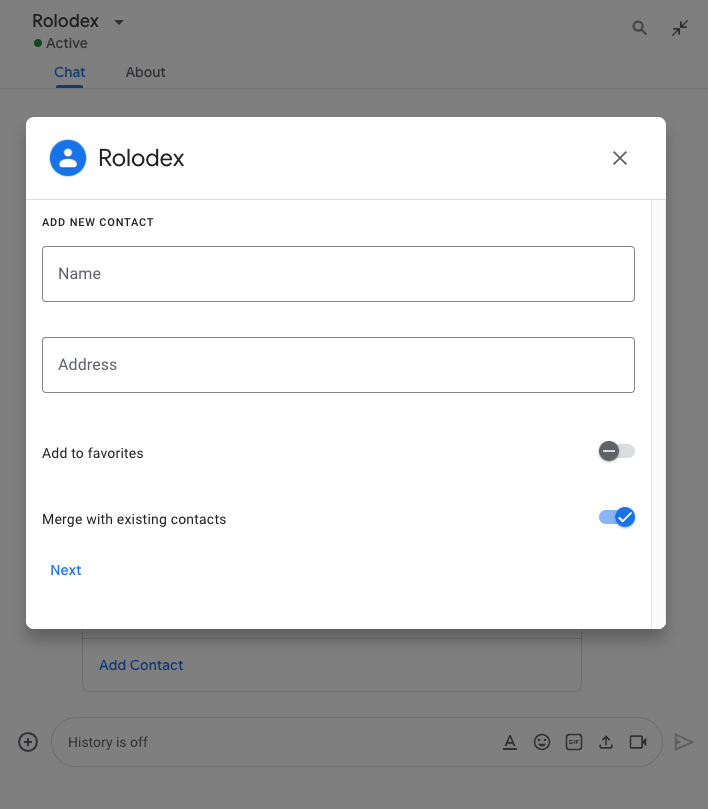
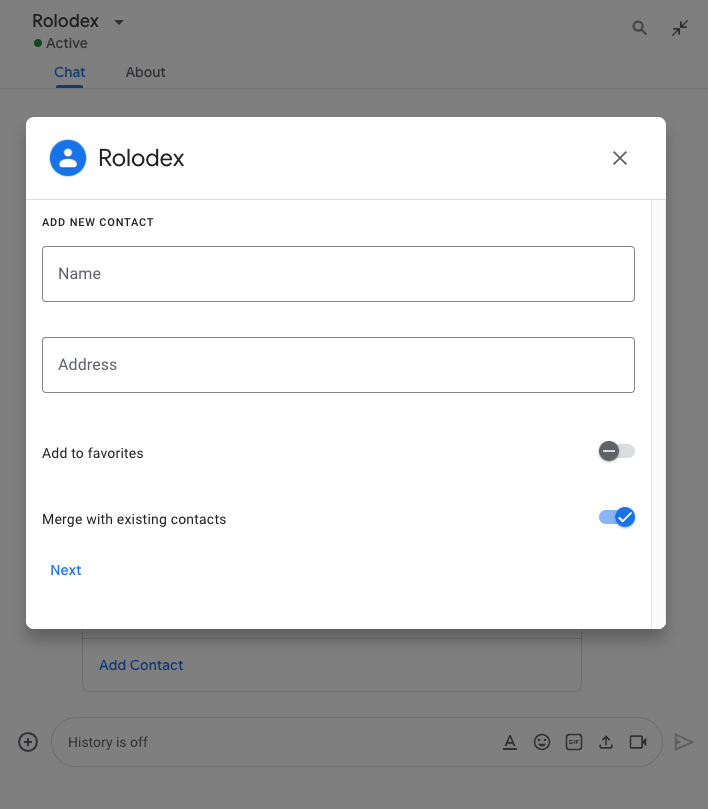
Bagian ini menjelaskan cara merespons dan menyiapkan dialog dengan melakukan hal berikut:
- Memicu permintaan dialog dari interaksi pengguna.
- Tangani permintaan dengan menampilkan dan membuka dialog.
- Setelah pengguna mengirimkan informasi, proses pengiriman dengan menutup dialog atau menampilkan dialog lainnya.
Memicu permintaan dialog
Aplikasi Chat hanya dapat membuka dialog untuk merespons interaksi pengguna, seperti perintah garis miring atau klik tombol dari pesan di kartu.
Untuk merespons pengguna dengan dialog, aplikasi Chat harus membangun interaksi yang memicu permintaan dialog, seperti berikut:
- Respons perintah garis miring. Untuk memicu permintaan dari perintah garis miring, Anda harus mencentang kotak Opens a dialog saat mengonfigurasi perintah.
- Merespons klik tombol dalam
pesan,
baik sebagai bagian dari kartu maupun di bagian bawah pesan. Untuk memicu
permintaan dari tombol dalam pesan, konfigurasi
tindakan
onClick
tombol dengan menetapkaninteraction
-nya keOPEN_DIALOG
. - Merespons klik tombol di halaman beranda aplikasi Chat. Untuk mempelajari cara membuka dialog dari halaman beranda, lihat Membuat halaman beranda untuk aplikasi Google Chat.
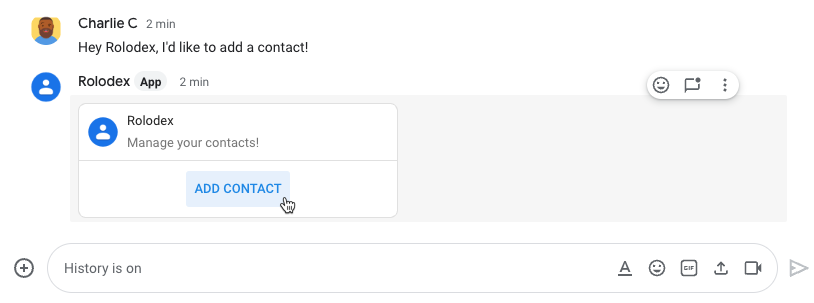
/addContact
. Pesan ini juga menyertakan tombol yang dapat diklik pengguna untuk memicu perintah.
Contoh kode berikut menunjukkan cara memicu permintaan dialog dari tombol dalam
pesan kartu. Untuk membuka dialog, kolom
button.interaction
disetel ke OPEN_DIALOG
:
Node.js
Python
Java
Apps Script
Contoh ini mengirim pesan kartu dengan menampilkan JSON kartu. Anda juga dapat menggunakan layanan kartu Apps Script.
Membuka dialog awal
Saat pengguna memicu permintaan dialog, aplikasi Chat Anda
akan menerima peristiwa interaksi, yang direpresentasikan sebagai
jenis event
di
Chat API. Jika interaksi memicu permintaan dialog, kolom
dialogEventType
peristiwa ditetapkan ke REQUEST_DIALOG
.
Untuk membuka dialog, aplikasi Chat Anda dapat merespons
permintaan dengan menampilkan objek
actionResponse
dengan type
yang ditetapkan ke DIALOG
dan
Message
. Untuk menentukan konten dialog, sertakan objek
berikut:
- Objek
actionResponse
, dengantype
-nya disetel keDIALOG
. - Objek
dialogAction
. Kolombody
berisi elemen antarmuka pengguna (UI) untuk ditampilkan di kartu, termasuk satu atau beberapa widgetsections
. Untuk mengumpulkan informasi dari pengguna, Anda dapat menentukan widget input formulir dan widget tombol. Untuk mempelajari lebih lanjut cara mendesain input formulir, lihat Mengumpulkan dan memproses informasi dari pengguna.
Contoh kode berikut menunjukkan cara aplikasi Chat menampilkan respons yang membuka dialog:
Node.js
Python
Java
Apps Script
Contoh ini mengirimkan pesan kartu dengan menampilkan JSON kartu. Anda juga dapat menggunakan layanan kartu Apps Script.
Menangani pengiriman dialog
Saat pengguna mengklik tombol yang mengirimkan dialog, aplikasi Chat Anda akan menerima peristiwa interaksi CARD_CLICKED
dengan dialogEventType
adalah SUBMIT_DIALOG
.
Aplikasi Chat Anda harus menangani peristiwa interaksi dengan melakukan salah satu hal berikut:
- Tampilkan dialog lain untuk mengisi kartu atau formulir lain.
- Tutup dialog setelah memvalidasi data yang dikirimkan pengguna, dan jika perlu, kirim pesan konfirmasi.
Opsional: Menampilkan dialog lain
Setelah pengguna mengirimkan dialog awal, aplikasi Chat dapat menampilkan satu atau beberapa dialog tambahan untuk membantu pengguna meninjau informasi sebelum mengirimkan, menyelesaikan formulir multi-langkah, atau mengisi konten formulir secara dinamis.
Untuk memproses data yang dimasukkan pengguna, aplikasi Chat
menggunakan
objek
event.common.formInputs
. Untuk mempelajari lebih lanjut cara mengambil nilai dari widget input, lihat
Mengumpulkan dan memproses informasi dari pengguna.
Untuk melacak data apa pun yang dimasukkan pengguna dari dialog awal, Anda harus menambahkan parameter ke tombol yang membuka dialog berikutnya. Untuk mengetahui detailnya, lihat Mentransfer data ke kartu lain.
Dalam contoh ini, aplikasi Chat membuka dialog awal yang mengarah ke dialog kedua untuk konfirmasi sebelum dikirim:
Node.js
Python
Java
Apps Script
Contoh ini mengirim pesan kartu dengan menampilkan JSON kartu. Anda juga dapat menggunakan layanan kartu Apps Script.
Tutup dialog
Saat pengguna mengklik tombol pada dialog, aplikasi Chat Anda akan menjalankan tindakan terkait dan memberikan informasi berikut ke objek peristiwa:
eventType
adalahCARD_CLICKED
.dialogEventType
adalahSUBMIT_DIALOG
.
Aplikasi Chat akan menampilkan
objek ActionResponse
dengan type
-nya ditetapkan ke DIALOG
dan dialogAction
.
Opsional: Menampilkan notifikasi
Saat menutup dialog, Anda juga dapat menampilkan notifikasi teks.
Aplikasi Chat dapat merespons dengan notifikasi berhasil atau error
dengan menampilkan
ActionResponse
dengan actionStatus
yang ditetapkan.
Contoh berikut memeriksa apakah parameter valid dan menutup dialog dengan notifikasi teks bergantung pada hasilnya:
Node.js
Python
Java
Apps Script
Contoh ini mengirim pesan kartu dengan menampilkan JSON kartu. Anda juga dapat menggunakan layanan kartu Apps Script.
Untuk mengetahui detail tentang meneruskan parameter antar-dialog, lihat Mentransfer data ke kartu lain.
Opsional: Mengirim pesan konfirmasi
Saat menutup dialog, Anda juga dapat mengirim pesan baru, atau memperbarui pesan yang sudah ada.
Untuk mengirim pesan baru, tampilkan objek ActionResponse
dengan type
yang ditetapkan ke NEW_MESSAGE
. Contoh berikut menutup
dialog dengan notifikasi teks dan pesan teks konfirmasi:
Node.js
Python
Java
Apps Script
Contoh ini mengirim pesan kartu dengan menampilkan JSON kartu. Anda juga dapat menggunakan layanan kartu Apps Script.
Untuk memperbarui pesan, tampilkan objek actionResponse
yang berisi pesan yang diperbarui dan tetapkan type
ke salah satu nilai berikut:
UPDATE_MESSAGE
: Memperbarui pesan yang memicu permintaan dialog.UPDATE_USER_MESSAGE_CARDS
: Memperbarui kartu dari pratinjau link.
Memecahkan masalah
Saat aplikasi atau kartu Google Chat menampilkan error, antarmuka Chat akan menampilkan pesan yang menyatakan "Terjadi error". atau "Tidak dapat memproses permintaan Anda". Terkadang UI Chat tidak menampilkan pesan error, tetapi aplikasi atau kartu Chat menghasilkan hasil yang tidak terduga; misalnya, pesan kartu mungkin tidak muncul.
Meskipun pesan error mungkin tidak ditampilkan di UI Chat, pesan error dan data log deskriptif akan tersedia untuk membantu Anda memperbaiki error saat logging error untuk aplikasi Chat diaktifkan. Untuk mendapatkan bantuan dalam melihat, melakukan proses debug, dan memperbaiki error, lihat Memecahkan masalah dan memperbaiki error Google Chat.
Topik terkait
- Lihat contoh Contact Manager, yang merupakan aplikasi Chat yang menggunakan dialog untuk mengumpulkan informasi kontak.
- Membuka dialog dari halaman beranda aplikasi Google Chat.
- Menyiapkan dan merespons perintah garis miring
- Memproses informasi yang dimasukkan oleh pengguna