Cette page explique comment votre application Chat peut ouvrir des boîtes de dialogue pour répondre aux utilisateurs.
Les boîtes de dialogue sont des interfaces basées sur des cartes qui s'ouvrent à partir d'un espace ou d'un message Chat. La boîte de dialogue et son contenu ne sont visibles que par l'utilisateur qui l'a ouverte.
Les applications Chat peuvent utiliser des boîtes de dialogue pour demander et collecter des informations auprès des utilisateurs de Chat, y compris des formulaires en plusieurs étapes. Pour en savoir plus sur les entrées de formulaire de création, consultez Collecter et traiter les informations des utilisateurs.
Prérequis
Node.js
Une application Google Chat pour laquelle les fonctionnalités interactives sont activées. Pour créer une application Chat interactive à l'aide d'un service HTTP, suivez ce guide de démarrage rapide.
Python
Une application Google Chat pour laquelle les fonctionnalités interactives sont activées. Pour créer une application Chat interactive à l'aide d'un service HTTP, suivez ce guide de démarrage rapide.
Java
Une application Google Chat pour laquelle les fonctionnalités interactives sont activées. Pour créer une application Chat interactive à l'aide d'un service HTTP, suivez ce guide de démarrage rapide.
Apps Script
Une application Google Chat pour laquelle les fonctionnalités interactives sont activées. Pour créer une application Chat interactive dans Apps Script, suivez ce guide de démarrage rapide.
Ouvrir une boîte de dialogue
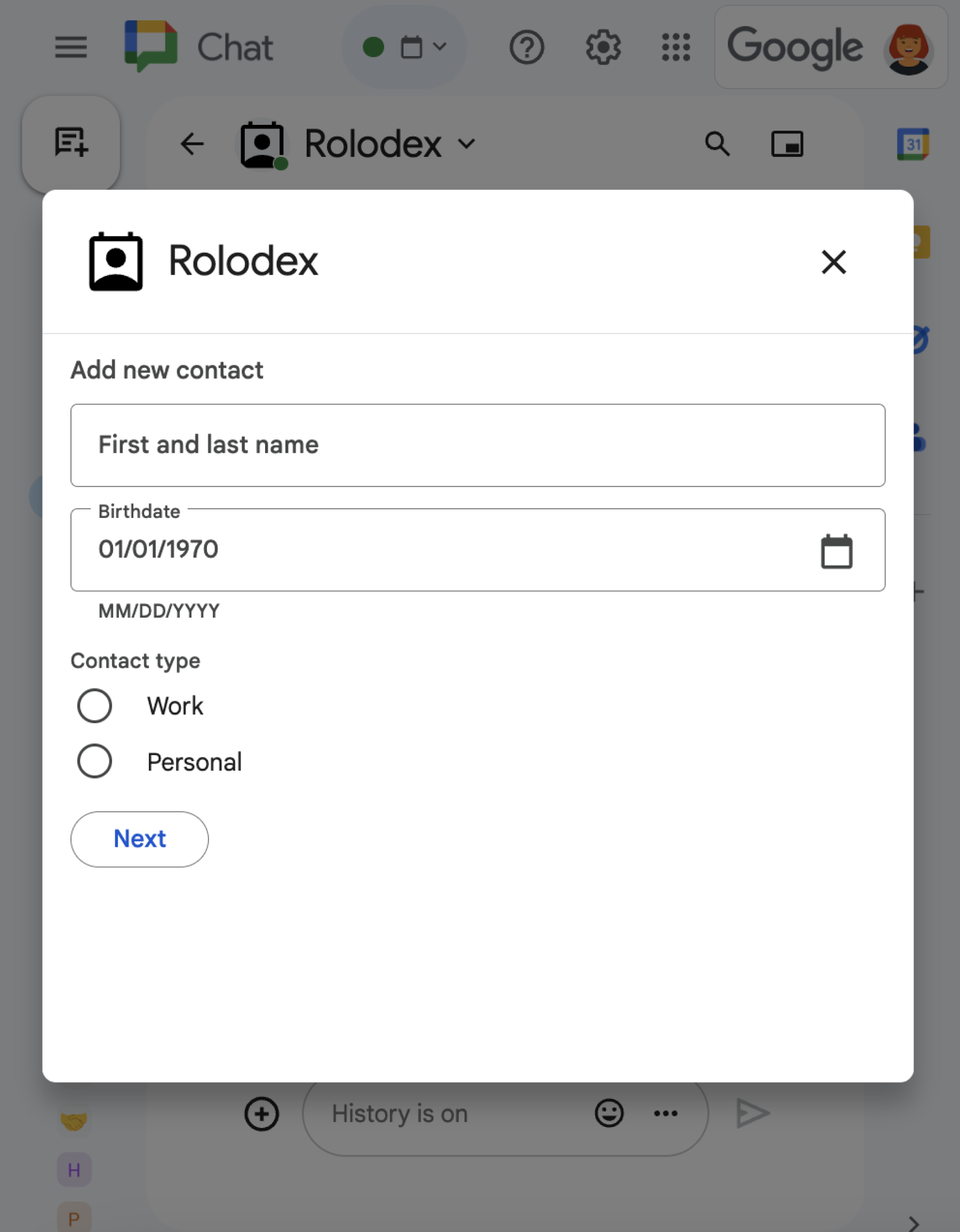
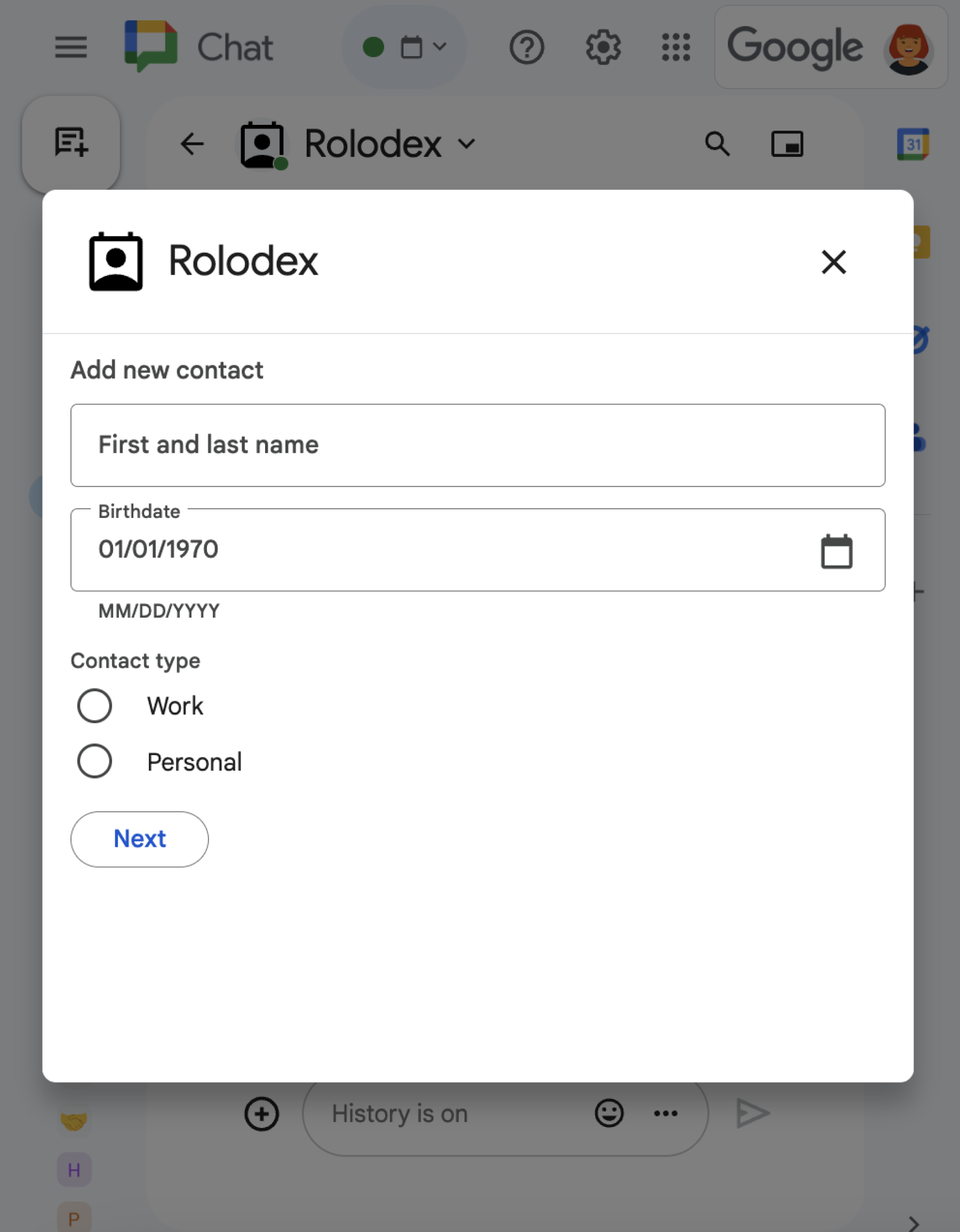
Cette section explique comment répondre et configurer une boîte de dialogue en procédant comme suit:
- Déclenchez la requête de boîte de dialogue à partir d'une interaction utilisateur.
- Gérez la requête en renvoyant et en ouvrant une boîte de dialogue.
- Une fois que les utilisateurs ont envoyé des informations, traitez-les en fermant la boîte de dialogue ou en renvoyant une autre boîte de dialogue.
Déclencher une requête de boîte de dialogue
Une application Chat ne peut ouvrir des boîtes de dialogue que pour répondre à une interaction utilisateur, telle qu'une commande à barre oblique ou un clic sur un bouton à partir d'un message dans une fiche.
Pour répondre aux utilisateurs avec une boîte de dialogue, une application Chat doit créer une interaction qui déclenche la requête de boîte de dialogue, par exemple:
- Répondez à une commande à barre oblique. Pour déclencher la requête à partir d'une commande à barre oblique, vous devez cocher la case Opens a dialog (Ouvre une boîte de dialogue) lorsque vous configurez la commande.
- Répondez à un clic sur un bouton dans un message, que ce soit dans une fiche ou en bas du message. Pour déclencher la requête à partir d'un bouton dans un message, vous devez configurer l'action
onClick
du bouton en définissant soninteraction
surOPEN_DIALOG
. - Répondre à un clic sur un bouton sur la page d'accueil d'une application Chat Pour en savoir plus sur l'ouverture de boîtes de dialogue à partir de pages d'accueil, consultez Créer une page d'accueil pour votre application Google Chat.
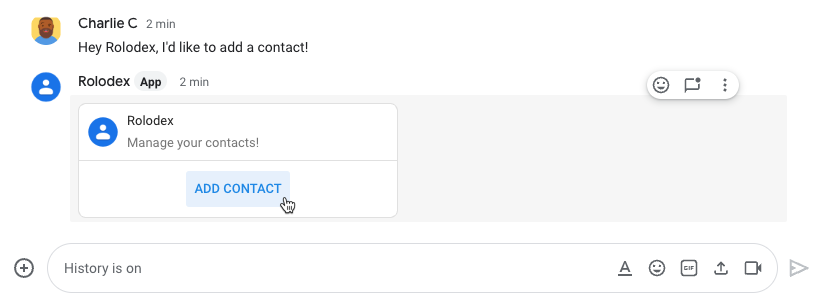
/addContact
. Le message inclut également un bouton sur lequel les utilisateurs peuvent cliquer pour déclencher la commande.
L'exemple de code suivant montre comment déclencher une requête de boîte de dialogue à partir d'un bouton dans un message de carte. Pour ouvrir la boîte de dialogue, le champ button.interaction
est défini sur OPEN_DIALOG
:
Node.js
Python
Java
Apps Script
Cet exemple envoie un message de carte en renvoyant un fichier JSON de carte. Vous pouvez également utiliser le service de carte Apps Script.
Ouvrir la boîte de dialogue initiale
Lorsqu'un utilisateur déclenche une requête de boîte de dialogue, votre application Chat reçoit un événement d'interaction, représenté par un type event
dans l'API Chat. Si l'interaction déclenche une requête de boîte de dialogue, le champ dialogEventType
de l'événement est défini sur REQUEST_DIALOG
.
Pour ouvrir une boîte de dialogue, votre application Chat peut répondre à la requête en renvoyant un objet actionResponse
avec type
défini sur DIALOG
et l'objet Message
. Pour spécifier le contenu de la boîte de dialogue, vous devez inclure les objets suivants:
- Objet
actionResponse
, avec sontype
défini surDIALOG
. - Un objet
dialogAction
. Le champbody
contient les éléments d'interface utilisateur (UI) à afficher dans la fiche, y compris un ou plusieurssections
de widgets. Pour collecter des informations auprès des utilisateurs, vous pouvez spécifier des widgets de saisie de formulaire et un widget de bouton. Pour en savoir plus sur la conception des entrées de formulaire, consultez la section Collecter et traiter les informations des utilisateurs.
L'exemple de code suivant montre comment une application Chat renvoie une réponse qui ouvre une boîte de dialogue:
Node.js
Python
Java
Apps Script
Cet exemple envoie un message de carte en renvoyant un fichier JSON de carte. Vous pouvez également utiliser le service de carte Apps Script.
Gérer l'envoi de la boîte de dialogue
Lorsque les utilisateurs cliquent sur un bouton qui envoie une boîte de dialogue, votre application Chat reçoit un événement d'interaction CARD_CLICKED
où dialogEventType
est SUBMIT_DIALOG
.
Votre application Chat doit gérer l'événement d'interaction en effectuant l'une des opérations suivantes:
- Renvoyer une autre boîte de dialogue pour renseigner une autre fiche ou un autre formulaire.
- Fermez la boîte de dialogue après avoir validé les données envoyées par l'utilisateur et, si vous le souhaitez, envoyez un message de confirmation.
Facultatif: renvoyer une autre boîte de dialogue
Une fois que les utilisateurs ont envoyé la boîte de dialogue initiale, les applications Chat peuvent renvoyer une ou plusieurs boîtes de dialogue supplémentaires pour les aider à examiner les informations avant de les envoyer, à remplir des formulaires en plusieurs étapes ou à renseigner le contenu des formulaires de manière dynamique.
Pour traiter les données saisies par les utilisateurs, l'application Chat utilise l'objet event.common.formInputs
. Pour en savoir plus sur la récupération de valeurs à partir de widgets de saisie, consultez la section Collecter et traiter les informations des utilisateurs.
Pour suivre les données que les utilisateurs saisissent à partir de la boîte de dialogue initiale, vous devez ajouter des paramètres au bouton qui ouvre la boîte de dialogue suivante. Pour en savoir plus, consultez Transférer des données vers une autre carte.
Dans cet exemple, une application Chat ouvre une boîte de dialogue initiale qui mène à une deuxième boîte de dialogue de confirmation avant l'envoi:
Node.js
Python
Java
Apps Script
Cet exemple envoie un message de carte en renvoyant un fichier JSON de carte. Vous pouvez également utiliser le service de carte Apps Script.
Fermer la boîte de dialogue
Lorsque les utilisateurs cliquent sur un bouton d'une boîte de dialogue, votre application Chat exécute l'action associée et fournit à l'objet d'événement les informations suivantes:
eventType
estCARD_CLICKED
.dialogEventType
estSUBMIT_DIALOG
.
L'application Chat doit renvoyer un objet ActionResponse
avec son type
défini sur DIALOG
et dialogAction
.
Facultatif: Afficher une notification
Lorsque vous fermez la boîte de dialogue, vous pouvez également afficher une notification textuelle.
L'application Chat peut répondre par une notification de réussite ou d'erreur en renvoyant un ActionResponse
avec actionStatus
défini.
L'exemple suivant vérifie que les paramètres sont valides et ferme la boîte de dialogue avec une notification textuelle en fonction du résultat:
Node.js
Python
Java
Apps Script
Cet exemple envoie un message de carte en renvoyant un fichier JSON de carte. Vous pouvez également utiliser le service de carte Apps Script.
Pour en savoir plus sur la transmission de paramètres entre les boîtes de dialogue, consultez la section Transférer des données vers une autre fiche.
Facultatif: Envoyer un message de confirmation
Lorsque vous fermez la boîte de dialogue, vous pouvez également envoyer un nouveau message ou modifier un message existant.
Pour envoyer un nouveau message, renvoyez un objet ActionResponse
avec type
défini sur NEW_MESSAGE
. L'exemple suivant ferme la boîte de dialogue avec une notification et un message de confirmation par SMS:
Node.js
Python
Java
Apps Script
Cet exemple envoie un message de carte en renvoyant un fichier JSON de carte. Vous pouvez également utiliser le service de carte Apps Script.
Pour mettre à jour un message, renvoyez un objet actionResponse
contenant le message mis à jour et définissez type
sur l'un des éléments suivants:
UPDATE_MESSAGE
: met à jour le message qui a déclenché la requête de boîte de dialogue.UPDATE_USER_MESSAGE_CARDS
: met à jour la fiche à partir d'un aperçu de lien.
Résoudre les problèmes
Lorsqu'une application ou une fiche Google Chat renvoie une erreur, l'interface Chat affiche le message "Un problème est survenu". ou "Impossible de traiter votre demande". Il arrive que l'interface utilisateur de Chat n'affiche aucun message d'erreur, mais que l'application ou la fiche Chat produise un résultat inattendu. Par exemple, un message de fiche peut ne pas s'afficher.
Bien qu'un message d'erreur ne s'affiche pas dans l'interface utilisateur de Chat, des messages d'erreur descriptifs et des données de journal sont disponibles pour vous aider à corriger les erreurs lorsque la journalisation des erreurs pour les applications Chat est activée. Pour savoir comment afficher, déboguer et corriger les erreurs, consultez Résoudre et corriger les erreurs Google Chat.
Articles associés
- Affichez l'exemple de Gestionnaire de contacts, une application Chat qui utilise des boîtes de dialogue pour collecter des coordonnées.
- Ouvrir des boîtes de dialogue à partir de la page d'accueil d'une application Google Chat
- Configurer et répondre aux commandes à barre oblique
- Traiter les informations saisies par les utilisateurs