W tym przewodniku opisano, jak aplikacje Google Chat mogą zbierać i przetwarzać informacje od użytkowników dzięki tworzeniu formularzy w interfejsach opartych na kartach.
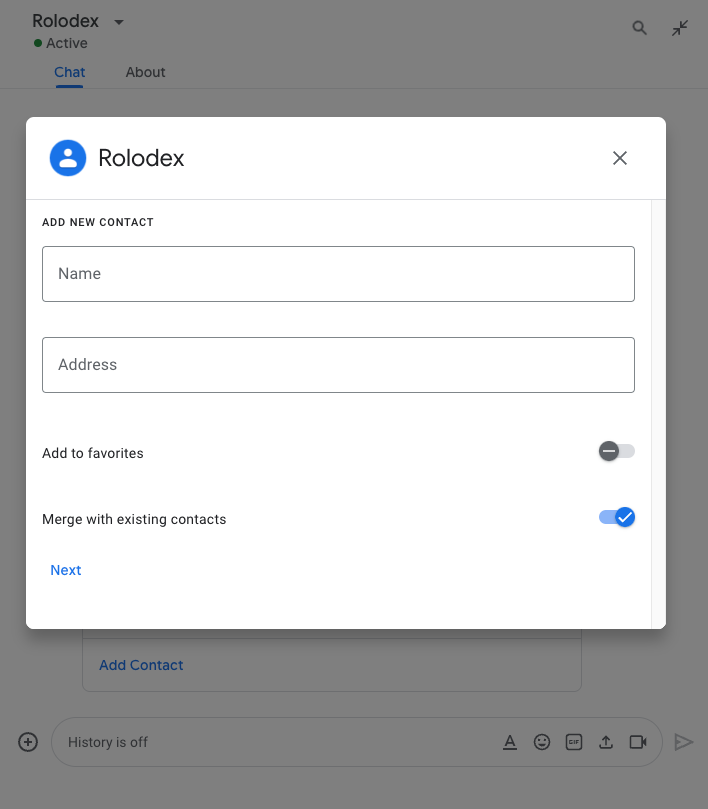
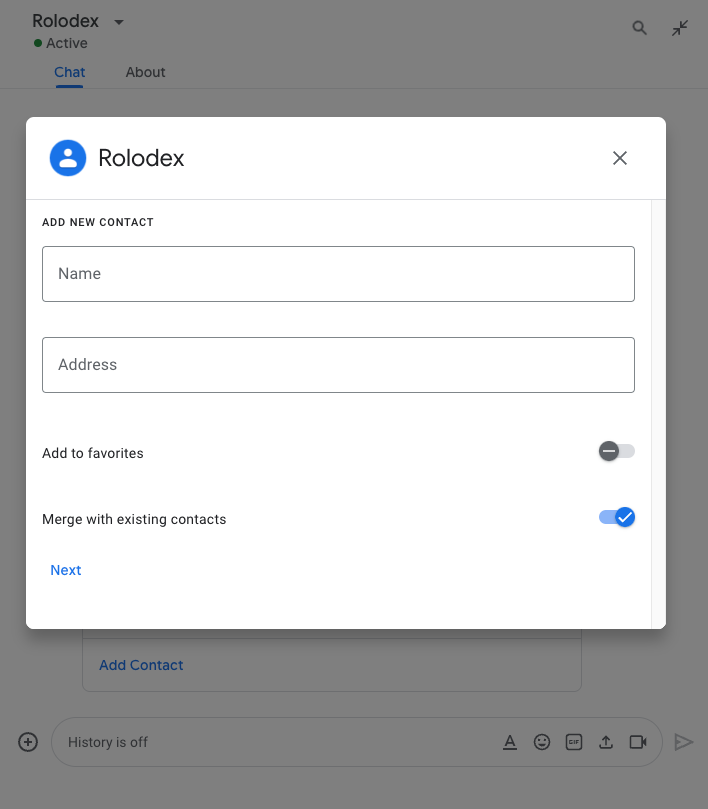
Aplikacje do obsługi czatu wymagają od użytkowników informacji, aby wykonywać działania w Google Chat lub poza nim, w tym w następujący sposób:
- Skonfiguruj ustawienia. Na przykład, aby umożliwić użytkownikom dostosowywanie ustawień powiadomień lub skonfigurowanie i dodanie aplikacji Google Chat do jednego lub większej liczby pokoi.
- tworzyć i aktualizować informacje w innych aplikacjach Google Workspace. Na przykład możesz zezwolić użytkownikom na tworzenie wydarzeń w Kalendarzu Google.
- Zezwalanie użytkownikom na dostęp do zasobów w innych aplikacjach lub usługach internetowych oraz ich aktualizowanie. Na przykład aplikacja Google Chat może pomóc użytkownikom zaktualizować stan zgłoszenia do zespołu pomocy bezpośrednio w pokoju czatu.
Wymagania wstępne
Node.js
Aplikacja Google Chat, w której włączono funkcje interaktywne. Aby utworzyć interaktywną aplikację Google Chat przy użyciu usługi HTTP, wykonaj to krótkie wprowadzenie.
Python
Aplikacja Google Chat, w której włączono funkcje interaktywne. Aby utworzyć interaktywną aplikację Google Chat przy użyciu usługi HTTP, wykonaj to krótkie wprowadzenie.
Java
Aplikacja Google Chat, w której włączono funkcje interaktywne. Aby utworzyć interaktywną aplikację Google Chat przy użyciu usługi HTTP, wykonaj to krótkie wprowadzenie.
Google Apps Script
Aplikacja Google Chat, w której włączono funkcje interaktywne. Aby utworzyć interaktywną aplikację Google Chat w Apps Script, wykonaj te czynności.
Tworzenie formularzy za pomocą kart
Aby zbierać informacje, aplikacje Google Chat projektują formularze i ich pola, a następnie umieszczają je na kartach. Aby wyświetlać karty użytkownikom, aplikacje na czacie mogą korzystać z tych interfejsów czatu:
- Wiadomości zawierające co najmniej 1 kartę.
- Strony główne – karta, która pojawia się na karcie Strona główna w wiadomościach bezpośrednich w aplikacji Google Chat.
- Dialogi, czyli karty otwierane w nowym oknie z wiadomości i stron głównych.
Aplikacje do czatu mogą tworzyć karty za pomocą tych widżetów:
widżety formularzy, które wymagają od użytkowników podania informacji; Opcjonalnie możesz dodać weryfikację do formularzy z elementami wprowadzania danych, aby zapewnić prawidłowe wprowadzanie i formatowanie informacji przez użytkowników. Aplikacje do czatu mogą używać tych widżetów wprowadzania danych:
- Tekst do wpisania (
textInput
) w postaci tekstu niesformatowanego lub sugerowanego. - Elementy wyboru (
selectionInput
) to elementy interfejsu, które można wybrać, takie jak pola wyboru, przyciski opcji i menu. Widgety z danymi wyboru mogą też wypełniać elementy z źródeł danych statycznych lub dynamicznych. Użytkownicy mogą na przykład wybrać pokój czatu z listy pokoi, do których należą. - Selektory daty i godziny (
dateTimePicker
) do wpisów z datą i godziną.
- Tekst do wpisania (
Przycisk, dzięki któremu użytkownicy mogą przesyłać wartości wprowadzone na karcie. Gdy użytkownik kliknie przycisk, aplikacja Czat może przetworzyć otrzymane informacje.
W tym przykładzie karta zbiera informacje kontaktowe za pomocą pola tekstowego, selektora daty i godziny oraz pola wyboru:
Przykład aplikacji czatu, która korzysta z tego formularza kontaktowego, znajdziesz w tym kodzie:
Node.js
Python
Java
Google Apps Script
Więcej przykładów interaktywnych widżetów, które możesz wykorzystać do zbierania informacji, znajdziesz w artykule Projektowanie interaktywnej karty lub dialogu.
Otrzymywanie danych z interaktywnych widżetów
Gdy użytkownicy klikają przycisk, aplikacje do czatu otrzymują zdarzenie interakcji CARD_CLICKED
, które zawiera informacje o tej interakcji. Ładunek zdarzeń interakcji CARD_CLICKED
zawiera obiekt common.formInputs
z dowolnymi wartościami wprowadzonymi przez użytkownika.
Wartości możesz pobrać z obiektu common.formInputs.WIDGET_NAME
, gdzie WIDGET_NAME to pole name
określone dla widżetu.
Wartości są zwracane jako określony typ danych dla widżetu (przedstawiany jako obiekt Inputs
).
Poniżej widać fragment zdarzenia interakcji CARD_CLICKED
, w którym użytkownik wprowadził wartości dla każdego widżetu:
HTTP
{
"type": "CARD_CLICKED",
"common": { "formInputs": {
"contactName": { "stringInputs": {
"value": ["Kai 0"]
}},
"contactBirthdate": { "dateInput": {
"msSinceEpoch": 1000425600000
}},
"contactType": { "stringInputs": {
"value": ["Personal"]
}}
}}
}
Google Apps Script
{
"type": "CARD_CLICKED",
"common": { "formInputs": {
"contactName": { "": { "stringInputs": {
"value": ["Kai 0"]
}}},
"contactBirthdate": { "": { "dateInput": {
"msSinceEpoch": 1000425600000
}}},
"contactType": { "": { "stringInputs": {
"value": ["Personal"]
}}}
}}
}
Aby otrzymywać dane, aplikacja Google Chat obsługuje zdarzenie interakcji, aby pobierać wartości wprowadzane przez użytkowników w widżetach. Poniższa tabela pokazuje, jak uzyskać wartość danego widżetu formularza. W przypadku każdego widżetu tabela zawiera typ danych, który akceptuje, miejsce przechowywania wartości w zdarzeniu interakcji oraz przykładową wartość.
Widżet pola formularza | Typ danych wejściowych | Wartość wejściowa z zdarzenia interakcji | Przykładowa wartość |
---|---|---|---|
textInput |
stringInputs |
event.common.formInputs.contactName.stringInputs.value[0] |
Kai O |
selectionInput |
stringInputs |
Aby uzyskać pierwszą lub jedyną wartość, event.common.formInputs.contactType.stringInputs.value[0] |
Personal |
dateTimePicker , który akceptuje tylko daty. |
dateInput |
event.common.formInputs.contactBirthdate.dateInput.msSinceEpoch . |
1000425600000 |
Przenoszenie danych na inną kartę
Gdy użytkownik prześle informacje z karty, może być konieczne zwrócenie dodatkowych kart, aby wykonać jedną z tych czynności:
- Ułatwiaj użytkownikom wypełnianie dłuższych formularzy, tworząc odrębne sekcje.
- Pozwól użytkownikom wyświetlić i potwierdzić informacje z pierwotnej karty, aby mogli sprawdzić swoje odpowiedzi przed przesłaniem.
- dynamicznie wypełnić pozostałe części formularza; Aby na przykład zachęcić użytkowników do utworzenia spotkania, aplikacja Chat może wyświetlić kartę z prośbą o podanie powodu spotkania, a następnie wypełnić kolejną kartę z dostępnymi godzinami na podstawie typu spotkania.
Aby przenieść dane z pierwotnej karty, możesz utworzyć widżet button
z actionParameters
, który zawiera name
widżetu i wartość wprowadzoną przez użytkownika, jak pokazano w tym przykładzie:
Node.js
Python
Java
Google Apps Script
Gdy użytkownik kliknie przycisk, aplikacja Google Chat otrzyma zdarzenie interakcji CARD_CLICKED
, z którego możesz otrzymać dane.
Odpowiedź na przesłanie formularza
Po otrzymaniu danych z wiadomości na karcie lub z dialogu aplikacja Google Chat potwierdza otrzymanie danych lub zwraca błąd.
W tym przykładzie aplikacja Google Chat wysyła wiadomość tekstową z potwierdzeniem, że otrzymała formularz przesłany z poziomu okna dialogowego lub wiadomości na karcie.
Node.js
Python
Java
Google Apps Script
Aby przetworzyć i zamknąć okno, zwracasz obiekt ActionResponse
, który określa, czy chcesz wysłać wiadomość z potwierdzeniem, zaktualizować pierwotną wiadomość lub kartę, czy po prostu zamknąć okno. Instrukcje znajdziesz w artykule Zamykanie okna dialogowego.
Rozwiązywanie problemów
Gdy aplikacja Google Chat lub karta zwraca błąd, interfejs Google Chat wyświetla komunikat „Coś poszło nie tak”. lub „Nie udało się przetworzyć Twojej prośby”. Czasami interfejs czatu nie wyświetla żadnego komunikatu o błędzie, ale aplikacja lub karta czatu powoduje nieoczekiwany wynik, na przykład wiadomość na karcie może się nie wyświetlić.
Komunikat o błędzie może się nie wyświetlać w interfejsie czatu, ale gdy włączone jest rejestrowanie błędów w przypadku aplikacji czatu, dostępne są opisowe komunikaty o błędach i dane dziennika, które pomogą Ci je naprawić. Aby dowiedzieć się, jak wyświetlać, debugować i naprawiać błędy, przeczytaj artykuł Rozwiązywanie problemów z błędami Google Chat.
Powiązane artykuły
- Wyświetl przykładowy Menedżer kontaktów, czyli aplikację do czatu, która zachęca użytkowników do wypełniania formularza kontaktowego z wiadomości na karcie i dialogów.
- Otwieranie interaktywnych dialogów