简介
InfoWindow
用于在地图上的给定位置以弹出式窗口的形式显示内容(通常为文本或图片)。信息窗口包含一个内容区域和一个锥形柄,柄的尖端与地图上的指定位置相连。信息窗口以对话框的形式显示给屏幕阅读器。
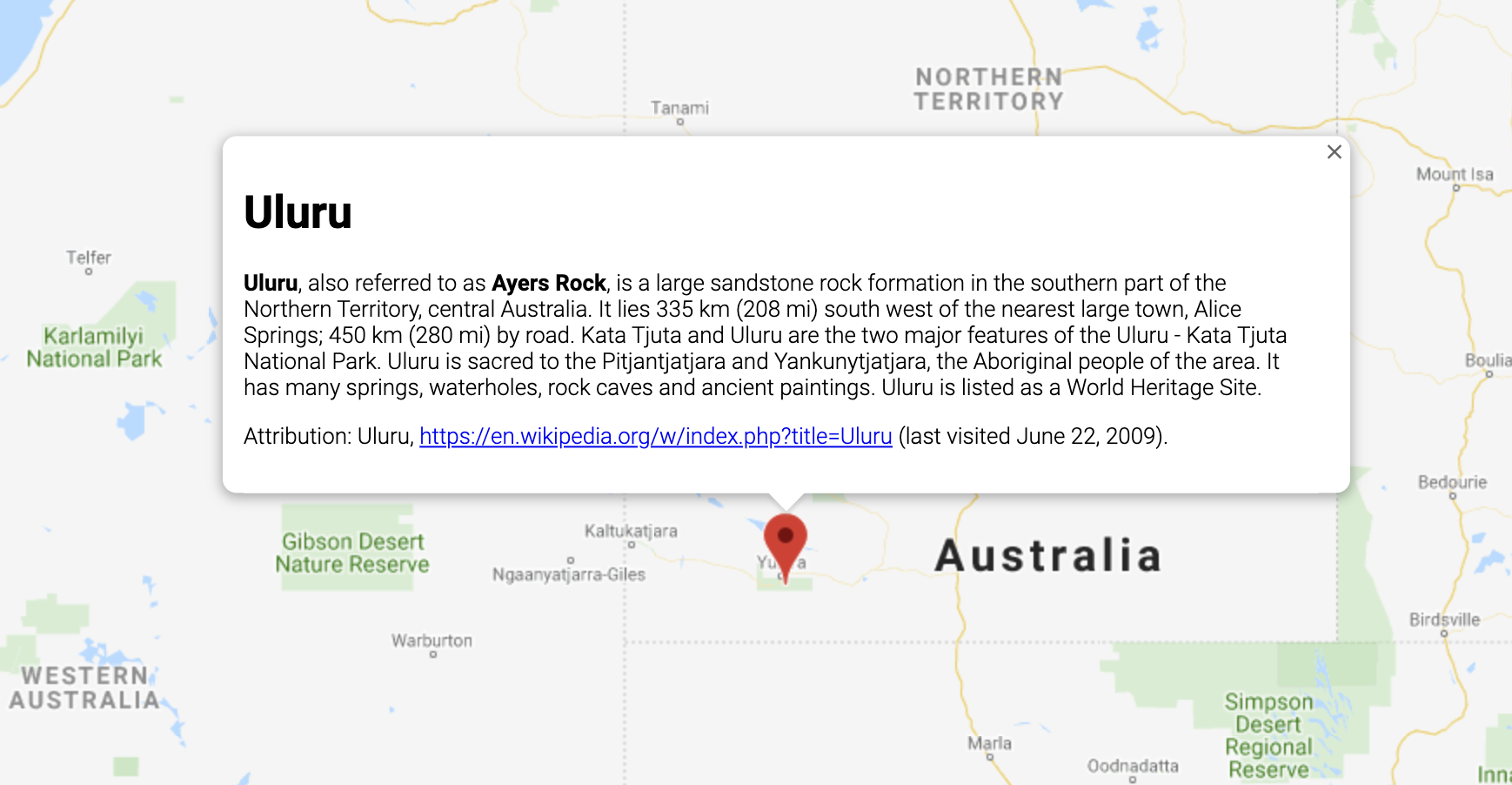
通常情况下,您需要将信息窗口附加到标记,但也可以将信息窗口附加到特定纬度/经度,具体说明见下文中的“添加信息窗口”部分。
从广义上来讲,信息窗口属于一种叠加层。如需了解其他类型的叠加层,请参阅在地图上绘图。
添加信息窗口
InfoWindow
构造函数采用
InfoWindowOptions
对象字面量作为参数,该对象字面量用于指定用来显示信息窗口的初始参数。
InfoWindowOptions
对象字面量包含下列字段:
content
,包含要在信息窗口中显示的文本字符串或 DOM 节点。pixelOffset
,包含从信息窗口尖端到信息窗口锚定位置的偏移量。在实际使用时,您不必指定此字段,可以保留其默认值。position
,包含此信息窗口锚定位置的LatLng
。注意:InfoWindow
可以附加到一个Marker
对象(在此情况下,信息窗口的位置取决于标记的位置),也可附加到地图本身上的指定LatLng
位置。若要获取LatLng
,一种方式是使用地理编码服务。在标记上打开信息窗口后,系统会自动更新position
。maxWidth
,用于指定信息窗口的最大宽度(以像素为单位)。默认情况下,信息窗口会根据其中包含的内容进行扩展,如果信息窗口填满地图,文本将会自动换行。如果您添加maxWidth
,信息窗口将会自动换行以强制适应指定宽度。如果信息窗口达到最大宽度,但屏幕上仍有纵向空间,信息窗口可能会纵向扩展。
InfoWindow
的内容可包含文本字符串、HTML 代码段或 DOM 元素。若要设置具体包含的内容,可以在 InfoWindowOptions
中指定相应内容,或者对 InfoWindow
显式调用 setContent()
。
如果您想要显式指定内容的大小,可以将内容放入 <div>
元素中,并使用 CSS 设置 <div>
的样式。您还可以使用 CSS 启用滚动功能。请注意,如果您不启用滚动功能,且内容超出信息窗口中可用的空间,内容可能会溢出信息窗口。
打开信息窗口
创建信息窗口后,它不会自动显示在地图上。若要显示信息窗口,您必须对 InfoWindow
调用 open()
方法,并传递 InfoWindowOpenOptions
对象字面量来指定以下选项:
map
用于指定要在哪张地图或街景全景图片上打开信息窗口。anchor
包含一个定位点(例如Marker
)。如果anchor
选项为null
或未指定,信息窗口将在其position
属性对应的位置打开。
TypeScript
// This example displays a marker at the center of Australia. // When the user clicks the marker, an info window opens. function initMap(): void { const uluru = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: uluru, } ); const contentString = '<div id="content">' + '<div id="siteNotice">' + "</div>" + '<h1 id="firstHeading" class="firstHeading">Uluru</h1>' + '<div id="bodyContent">' + "<p><b>Uluru</b>, also referred to as <b>Ayers Rock</b>, is a large " + "sandstone rock formation in the southern part of the " + "Northern Territory, central Australia. It lies 335 km (208 mi) " + "south west of the nearest large town, Alice Springs; 450 km " + "(280 mi) by road. Kata Tjuta and Uluru are the two major " + "features of the Uluru - Kata Tjuta National Park. Uluru is " + "sacred to the Pitjantjatjara and Yankunytjatjara, the " + "Aboriginal people of the area. It has many springs, waterholes, " + "rock caves and ancient paintings. Uluru is listed as a World " + "Heritage Site.</p>" + '<p>Attribution: Uluru, <a href="https://en.wikipedia.org/w/index.php?title=Uluru&oldid=297882194">' + "https://en.wikipedia.org/w/index.php?title=Uluru</a> " + "(last visited June 22, 2009).</p>" + "</div>" + "</div>"; const infowindow = new google.maps.InfoWindow({ content: contentString, ariaLabel: "Uluru", }); const marker = new google.maps.Marker({ position: uluru, map, title: "Uluru (Ayers Rock)", }); marker.addListener("click", () => { infowindow.open({ anchor: marker, map, }); }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example displays a marker at the center of Australia. // When the user clicks the marker, an info window opens. function initMap() { const uluru = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: uluru, }); const contentString = '<div id="content">' + '<div id="siteNotice">' + "</div>" + '<h1 id="firstHeading" class="firstHeading">Uluru</h1>' + '<div id="bodyContent">' + "<p><b>Uluru</b>, also referred to as <b>Ayers Rock</b>, is a large " + "sandstone rock formation in the southern part of the " + "Northern Territory, central Australia. It lies 335 km (208 mi) " + "south west of the nearest large town, Alice Springs; 450 km " + "(280 mi) by road. Kata Tjuta and Uluru are the two major " + "features of the Uluru - Kata Tjuta National Park. Uluru is " + "sacred to the Pitjantjatjara and Yankunytjatjara, the " + "Aboriginal people of the area. It has many springs, waterholes, " + "rock caves and ancient paintings. Uluru is listed as a World " + "Heritage Site.</p>" + '<p>Attribution: Uluru, <a href="https://en.wikipedia.org/w/index.php?title=Uluru&oldid=297882194">' + "https://en.wikipedia.org/w/index.php?title=Uluru</a> " + "(last visited June 22, 2009).</p>" + "</div>" + "</div>"; const infowindow = new google.maps.InfoWindow({ content: contentString, ariaLabel: "Uluru", }); const marker = new google.maps.Marker({ position: uluru, map, title: "Uluru (Ayers Rock)", }); marker.addListener("click", () => { infowindow.open({ anchor: marker, map, }); }); } window.initMap = initMap;
试用示例
以下示例设置了信息窗口的 maxWidth
:查看示例。
将焦点置于信息窗口中
如需将焦点置于信息窗口中,请调用其 focus()
方法。在设置焦点之前,不妨考虑将此方法与 visible
事件搭配使用。对不显示的信息窗口调用此方法不会产生任何效果。调用 open()
即可显示信息窗口。
关闭信息窗口
默认情况下,信息窗口保持打开状态,直至用户点击关闭控件(信息窗口右上角的叉号)或按下 Esc 键。您还可以通过调用信息窗口的 close()
方法来显式关闭它。
关闭信息窗口后,焦点会移回到在信息窗口打开前焦点所在的元素。如果该元素已消失,焦点会移回到地图上。如需重写此行为,您可以监听 closeclick
事件并手动管理焦点,如下例中所示:
infoWindow.addListener('closeclick', ()=>{ // Handle focus manually. });
移动信息窗口
可以通过以下两种方式更改信息窗口的位置:
- 对信息窗口调用
setPosition()
,或者 - 使用
InfoWindow.open()
方法将信息窗口附加到新标记。注意:如果您调用open()
而不传递标记,InfoWindow
将使用构造时通过InfoWindowOptions
对象字面量指定的位置。
自定义
InfoWindow
类不提供自定义功能。如需了解如何创建完全自定义的弹出式窗口,请参阅自定义弹出式窗口示例。