查看完整的示例源代码
此示例展示了如何通过以下方式自定义标记:添加标题文本、缩放标记、更改背景颜色、更改边框颜色、更改字形颜色、隐藏字形。
TypeScript
const parser = new DOMParser(); async function initMap() { // Request needed libraries. const { Map } = await google.maps.importLibrary("maps") as google.maps.MapsLibrary; const { AdvancedMarkerElement, PinElement } = await google.maps.importLibrary("marker") as google.maps.MarkerLibrary; const map = new Map(document.getElementById('map') as HTMLElement, { center: { lat: 37.419, lng: -122.02 }, zoom: 14, mapId: '4504f8b37365c3d0', }); // Each PinElement is paired with a MarkerView to demonstrate setting each parameter. // Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: 'Title text for the marker at lat: 37.419, lng: -122.03', }); // Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, }); // Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, }); // Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, }); // Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, }); const pinTextGlyph = new PinElement({ glyph: 'T', glyphColor: 'white', }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, }); // Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, }); } initMap();
JavaScript
const parser = new DOMParser(); async function initMap() { // Request needed libraries. const { Map } = await google.maps.importLibrary("maps"); const { AdvancedMarkerElement, PinElement } = await google.maps.importLibrary( "marker", ); const map = new Map(document.getElementById("map"), { center: { lat: 37.419, lng: -122.02 }, zoom: 14, mapId: "4504f8b37365c3d0", }); // Each PinElement is paired with a MarkerView to demonstrate setting each parameter. // Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", }); // Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, }); // Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, }); // Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, }); // Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, }); const pinTextGlyph = new PinElement({ glyph: "T", glyphColor: "white", }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, }); // Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, }); } initMap();
CSS
/* * Always set the map height explicitly to define the size of the div element * that contains the map. */ #map { height: 100%; } /* * Optional: Makes the sample page fill the window. */ html, body { height: 100%; margin: 0; padding: 0; }
HTML
<html> <head> <title>Advanced Marker Basic Customization</title> <link rel="stylesheet" type="text/css" href="./style.css" /> <script type="module" src="./index.js"></script> </head> <body> <div id="map"></div> <!-- prettier-ignore --> <script>(g=>{var h,a,k,p="The Google Maps JavaScript API",c="google",l="importLibrary",q="__ib__",m=document,b=window;b=b[c]||(b[c]={});var d=b.maps||(b.maps={}),r=new Set,e=new URLSearchParams,u=()=>h||(h=new Promise(async(f,n)=>{await (a=m.createElement("script"));e.set("libraries",[...r]+"");for(k in g)e.set(k.replace(/[A-Z]/g,t=>"_"+t[0].toLowerCase()),g[k]);e.set("callback",c+".maps."+q);a.src=`https://maps.${c}apis.com/maps/api/js?`+e;d[q]=f;a.onerror=()=>h=n(Error(p+" could not load."));a.nonce=m.querySelector("script[nonce]")?.nonce||"";m.head.append(a)}));d[l]?console.warn(p+" only loads once. Ignoring:",g):d[l]=(f,...n)=>r.add(f)&&u().then(()=>d[l](f,...n))}) ({key: "AIzaSyB41DRUbKWJHPxaFjMAwdrzWzbVKartNGg", v: "weekly"});</script> </body> </html>
试用示例
高级标记使用两个类来定义标记:AdvancedMarkerElement
类提供基本参数(position
、title
和 map
),PinElement
类包含进一步自定义的选项。
如需向地图添加标记,您必须先加载 marker
库,该库提供了 AdvancedMarkerElement
和 PinElement
类。
以下代码段展示了用于创建新的 PinElement
,然后将其应用于标记的代码。
// Create a pin element.
const pin = new PinElement({
scale: 1.5,
});
// Create a marker and apply the element.
const marker = new AdvancedMarkerElement({
map,
position: { lat: 37.419, lng: -122.02 },
content: pin.element,
});
在使用 HTML 创建的地图中,标记的基本参数是使用 gmp-advanced-marker
HTML 元素声明的;使用 PinElement
类的任何自定义都必须以编程方式应用。为此,您的代码必须从 HTML 页面检索 gmp-advanced-marker
元素。以下代码段展示了用于查询 gmp-advanced-marker
元素集合的代码,然后迭代结果以应用 PinElement
中声明的自定义设置。
// Return an array of markers.
const advancedMarkers = [...document.querySelectorAll('gmp-advanced-marker')];
// Loop through the markers
for (let i = 0; i < advancedMarkers.length; i++) {
const pin = new PinElement({
scale: 2.0,
});
marker.appendChild(pin.element);
}
本页介绍了如何通过以下方式自定义标记:
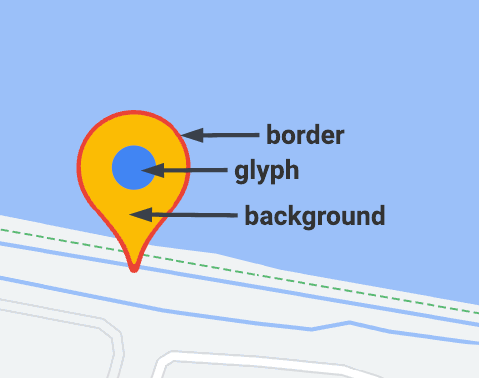
添加标题文字
将光标悬停在标记上时会显示标题文字。标题文本可由屏幕阅读器读出。
如需以程序化方式添加标题文本,请使用 AdvancedMarkerElement.title
选项:
// Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
如需向使用 HTML 创建的标记添加标题文本,请使用 title
属性:
<gmp-map center="43.4142989,-124.2301242" zoom="4" map-id="DEMO_MAP_ID" style="height: 400px" > <gmp-advanced-marker position="37.4220656,-122.0840897" title="Mountain View, CA" ></gmp-advanced-marker> <gmp-advanced-marker position="47.648994,-122.3503845" title="Seattle, WA" ></gmp-advanced-marker> </gmp-map>
缩放标记
如需缩放标记,请使用 scale
选项。
TypeScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
JavaScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
更改背景颜色
使用 PinElement.background
选项可更改标记的背景颜色:
TypeScript
// Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
JavaScript
// Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
更改边框颜色
使用 PinElement.borderColor
选项可更改标记的边框颜色:
TypeScript
// Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, });
JavaScript
// Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, });
更改字形颜色
使用 PinElement.glyphColor
选项可更改标记的字形颜色:
TypeScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, });
JavaScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, });
为图形添加文本
使用 PinElement.glyph
选项可将默认字形替换为文本字符。PinElement
的文本字形会随 PinElement
一起放大,其默认颜色与 PinElement
的默认 glyphColor
一致。
TypeScript
const pinTextGlyph = new PinElement({ glyph: 'T', glyphColor: 'white', }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, });
JavaScript
const pinTextGlyph = new PinElement({ glyph: "T", glyphColor: "white", }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, });
隐藏字形
将 PinElement.glyph
选项设置为空字符串可隐藏标记的字形:
TypeScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, });
JavaScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, });
此外,还可以将 PinElement.glyphColor
设为与 PinElement.background
一样的值。此操作可实现隐藏字形的视觉效果。