本指南介绍了如何对 Google Chat API 的 Message
资源使用 create()
方法执行以下任一操作:
- 发送包含文本、卡片和互动式微件的邮件。
- 向特定 Chat 用户私下发送消息。
- 发起或回复消息串。
- 为消息命名,以便您在其他 Chat API 请求中指定该消息。
消息大小(包括所有文本或卡片)上限为 32,000 字节。 如需发送超出此大小的消息,Chat 应用必须改为发送多条消息。
除了调用 Chat API 来创建消息之外,Chat 应用还可以创建和发送消息来回复用户互动,例如在用户将 Chat 应用添加到聊天室后发布欢迎消息。在响应互动时,Chat 应用可以使用其他类型的消息功能,包括交互式对话框和链接预览界面。如需回复用户,Chat 应用会同步返回消息,而无需调用 Chat API。如需了解如何发送消息来回复互动,请参阅接收和回复 Google Chat 应用中的互动。
Chat 如何显示和归因使用 Chat API 创建的消息
您可以使用应用身份验证和用户身份验证调用 create()
方法。Chat 会根据您使用的身份验证类型,以不同的方式为消息发件人分配属性。
当您以 Chat 应用的身份进行身份验证时,Chat 应用会发送消息。
App
。当您以用户身份进行身份验证时,Chat 应用会代表该用户发送消息。Chat 还会显示 Chat 应用的名称,以将该应用归因于消息。
身份验证类型还决定了您可以在消息中包含哪些消息功能和界面。借助应用身份验证,Chat 应用可以发送包含富文本、基于卡片的界面和互动式 widget 的消息。由于 Chat 用户只能在消息中发送文本,因此您只能在使用用户身份验证创建消息时添加文本。如需详细了解 Chat API 提供的消息功能,请参阅 Google Chat 消息概览。
本指南介绍了如何使用任一身份验证类型通过 Chat API 发送消息。
前提条件
Node.js
- 拥有对 Google Chat 访问权限的商务版或企业版 Google Workspace 账号。
- 设置环境:
- 创建 Google Cloud 项目。
- 配置 OAuth 同意屏幕。
- 启用和配置 Google Chat API,为 Chat 应用提供名称、图标和说明。
- 安装 Node.js Cloud 客户端库。
- 根据您要在 Google Chat API 请求中采用的身份验证方式创建访问凭据:
- 如需以 Chat 用户身份进行身份验证,请创建 OAuth 客户端 ID 凭据,并将凭据保存为名为
client_secrets.json
的 JSON 文件,保存到本地目录中。 - 如需以 Chat 应用的身份进行身份验证,请创建服务账号凭据,并将凭据保存为名为
credentials.json
的 JSON 文件。
- 如需以 Chat 用户身份进行身份验证,请创建 OAuth 客户端 ID 凭据,并将凭据保存为名为
- 根据您是要以用户身份还是以 Chat 应用的身份进行身份验证, 选择授权范围。
- 已通过身份验证的用户或发起调用的 Chat 应用所属的 Google Chat 聊天室。如需以 Chat 应用的身份进行身份验证,请将 Chat 应用添加到聊天室。
Python
- 拥有对 Google Chat 访问权限的商务版或企业版 Google Workspace 账号。
- 设置环境:
- 创建 Google Cloud 项目。
- 配置 OAuth 同意屏幕。
- 启用和配置 Google Chat API,为 Chat 应用提供名称、图标和说明。
- 安装 Python Cloud 客户端库。
- 根据您要在 Google Chat API 请求中采用的身份验证方式创建访问凭据:
- 如需以 Chat 用户身份进行身份验证,请创建 OAuth 客户端 ID 凭据,并将凭据保存为名为
client_secrets.json
的 JSON 文件,保存到本地目录中。 - 如需以 Chat 应用的身份进行身份验证,请创建服务账号凭据,并将凭据保存为名为
credentials.json
的 JSON 文件。
- 如需以 Chat 用户身份进行身份验证,请创建 OAuth 客户端 ID 凭据,并将凭据保存为名为
- 根据您是要以用户身份还是以 Chat 应用的身份进行身份验证, 选择授权范围。
- 已通过身份验证的用户或发起调用的 Chat 应用所属的 Google Chat 聊天室。如需以 Chat 应用的身份进行身份验证,请将 Chat 应用添加到聊天室。
Java
- 拥有对 Google Chat 访问权限的商务版或企业版 Google Workspace 账号。
- 设置环境:
- 创建 Google Cloud 项目。
- 配置 OAuth 同意屏幕。
- 启用和配置 Google Chat API,为 Chat 应用提供名称、图标和说明。
- 安装 Java Cloud 客户端库。
- 根据您要在 Google Chat API 请求中采用的身份验证方式创建访问凭据:
- 如需以 Chat 用户身份进行身份验证,请创建 OAuth 客户端 ID 凭据,并将凭据保存为名为
client_secrets.json
的 JSON 文件,保存到本地目录中。 - 如需以 Chat 应用的身份进行身份验证,请创建服务账号凭据,并将凭据保存为名为
credentials.json
的 JSON 文件。
- 如需以 Chat 用户身份进行身份验证,请创建 OAuth 客户端 ID 凭据,并将凭据保存为名为
- 根据您是要以用户身份还是以 Chat 应用的身份进行身份验证, 选择授权范围。
- 已通过身份验证的用户或发起调用的 Chat 应用所属的 Google Chat 聊天室。如需以 Chat 应用的身份进行身份验证,请将 Chat 应用添加到聊天室。
Apps 脚本
- 拥有对 Google Chat 访问权限的商务版或企业版 Google Workspace 账号。
- 设置环境:
- 创建 Google Cloud 项目。
- 配置 OAuth 同意屏幕。
- 启用和配置 Google Chat API,为 Chat 应用提供名称、图标和说明。
- 创建一个独立的 Apps 脚本项目,然后开启高级聊天服务。
- 在本指南中,您必须使用用户身份验证或应用身份验证。如需以 Chat 应用的身份进行身份验证,请创建服务账号凭据。如需了解具体步骤,请参阅以 Google Chat 应用的身份进行身份验证和授权。
- 根据您是要以用户身份还是以 Chat 应用的身份进行身份验证, 选择授权范围。
- 已通过身份验证的用户或发起调用的 Chat 应用所属的 Google Chat 聊天室。如需以 Chat 应用的身份进行身份验证,请将 Chat 应用添加到聊天室。
以 Chat 应用的身份发送消息
本部分介绍了如何使用应用身份验证发送包含文本、卡片和互动式配件 widget 的消息。
如需使用应用身份验证调用 CreateMessage()
方法,您必须在请求中指定以下字段:
chat.bot
授权范围。- 您要发布消息的
Space
资源。Chat 应用必须是聊天室的成员。 - 要创建的
Message
资源。如需定义消息的内容,您可以添加富文本 (text
)、一个或多个卡片界面 (cardsV2
),或同时添加这两者。
(可选)您可以添加以下内容:
accessoryWidgets
字段,用于添加消息底部的互动按钮。privateMessageViewer
字段,用于向指定用户私下发送消息。messageId
字段,用于为消息命名,以便在其他 API 请求中使用。thread.threadKey
和messageReplyOption
字段,用于发起或回复会话。如果聊天室不使用线程,系统会忽略此字段。
以下代码示例展示了 Chat 应用如何发送作为 Chat 应用发布的消息,其中包含文本、卡片以及消息底部的可点击按钮:
Node.js
Python
Java
Apps 脚本
如需运行此示例,请将 SPACE_NAME
替换为聊天室的 name
字段中的 ID。您可以通过调用 ListSpaces()
方法或从聊天室的网址中获取此 ID。
在邮件底部添加互动式微件
在本指南的第一个代码示例中,Chat 应用消息会在消息底部显示一个可点击的按钮,称为配件 widget。配件微件会显示在消息中的任何文本或卡片后面。您可以使用这些 widget 以多种方式提示用户与您的消息互动,包括:
- 评分消息的准确性或满意度。
- 举报与信息或 Chat 应用相关的问题。
- 打开指向相关内容(例如文档)的链接。
- 在特定时间段内关闭或暂停 Chat 应用中的类似消息。
如需添加配件 widget,请在请求正文中添加 accessoryWidgets[]
字段,并指定要添加的一个或多个 widget。
下图显示了一个 Chat 应用,该应用会在文本消息后附加配件 widget,以便用户对 Chat 应用的使用体验进行评分。
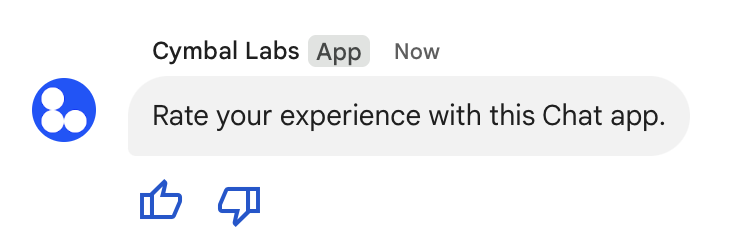
以下是用于创建包含两个配件按钮的文本消息的请求正文。当用户点击按钮时,相应的函数(例如 doUpvote
)会处理互动:
{
text: "Rate your experience with this Chat app.",
accessoryWidgets: [{ buttonList: { buttons: [{
icon: { material_icon: {
name: "thumb_up"
}},
color: { red: 0, blue: 255, green: 0 },
onClick: { action: {
function: "doUpvote"
}}
}, {
icon: { material_icon: {
name: "thumb_down"
}},
color: { red: 0, blue: 255, green: 0 },
onClick: { action: {
function: "doDownvote"
}}
}]}}]
}
私下发送消息
Chat 扩展应用可以私下发送消息,这样消息只会向聊天室中的特定用户显示。当 Chat 应用发送私信时,消息会显示一个标签,告知用户该消息只对他可见。
如需使用 Chat API 私下发送消息,请在请求正文中指定 privateMessageViewer
字段。如需指定用户,请将该值设置为代表 Chat 用户的 User
资源。您还可以使用 User
资源的 name
字段,如以下示例所示:
{
text: "Hello private world!",
privateMessageViewer: {
name: "users/USER_ID"
}
}
如需使用此示例,请将 USER_ID
替换为用户的唯一 ID,例如 12345678987654321
或 hao@cymbalgroup.com
。如需详细了解如何指定用户,请参阅识别和指定 Google Chat 用户。
如需私下发送消息,您必须在请求中省略以下内容:
代表用户发送短信
本部分介绍了如何使用用户身份验证代表用户发送消息。在用户进行身份验证后,消息内容只能包含文本,并且必须省略仅适用于 Chat 应用的消息功能,包括卡片界面和交互式 widget。
如需使用用户身份验证调用 CreateMessage()
方法,您必须在请求中指定以下字段:
- 支持此方法的用户身份验证的授权范围。以下示例使用
chat.messages.create
作用域。 - 您要发布消息的
Space
资源。经过身份验证的用户必须是聊天室的成员。 - 要创建的
Message
资源。如需定义消息内容,您必须添加text
字段。
(可选)您可以添加以下内容:
messageId
字段,用于为消息命名,以便在其他 API 请求中使用。thread.threadKey
和messageReplyOption
字段,用于发起或回复会话。如果聊天室不使用线程,系统会忽略此字段。
以下代码展示了 Chat 应用如何代表已通过身份验证的用户在给定聊天室中发送短信的示例:
Node.js
Python
Java
Apps 脚本
如需运行此示例,请将 SPACE_NAME
替换为聊天室的 name
字段中的 ID。您可以通过调用 ListSpaces()
方法或从聊天室的网址中获取此 ID。
发起或在消息串中回复
对于使用话题的聊天室,您可以指定新消息是发起新话题,还是回复现有话题。
默认情况下,您使用 Chat API 创建的消息会启动新会话。为便于您日后识别会话并回复,您可以在请求中指定会话键:
- 在请求正文中,指定
thread.threadKey
字段。 - 指定查询参数
messageReplyOption
,以确定如果键已存在,会发生什么情况。
如需创建回复现有会话的消息,请执行以下操作:
以下代码示例展示了聊天应用如何代表已通过身份验证的用户发送文本消息,以启动或回复由给定聊天室的键标识的给定会话:
Node.js
Python
Java
Apps 脚本
如需运行此示例,请替换以下内容:
THREAD_KEY
:聊天室中的现有会话键,或者要创建新会话时,会话的唯一名称。SPACE_NAME
:聊天室的name
字段中的 ID。您可以通过调用ListSpaces()
方法或从聊天室的网址中获取此 ID。
为消息命名
如需在日后的 API 调用中检索或指定消息,您可以通过在请求中设置 messageId
字段来为消息命名。为消息命名后,您无需存储消息资源名称(以 name
字段表示)中系统分配的 ID,即可指定消息。
例如,如需使用 get()
方法检索消息,您可以使用资源名称指定要检索的消息。资源名称的格式为 spaces/{space}/messages/{message}
,其中 {message}
表示系统分配的 ID 或您在创建消息时设置的自定义名称。
如需为消息命名,请在创建消息时在 messageId
字段中指定自定义 ID。messageId
字段用于为 Message
资源的 clientAssignedMessageId
字段设置值。
您只能在创建消息时为其命名。您无法为现有消息命名或修改自定义 ID。自定义 ID 必须符合以下要求:
- 以
client-
开头。例如,client-custom-name
是有效的自定义 ID,但custom-name
不是。 - 最多可包含 63 个字符,且只能包含小写字母、数字和连字符。
- 在聊天室中是唯一的。Chat 应用无法为不同的消息使用相同的自定义 ID。
以下代码展示了 Chat 应用如何代表已通过身份验证的用户向给定聊天室发送包含 ID 的文本消息的示例:
Node.js
Python
Java
Apps 脚本
如需运行此示例,请替换以下内容:
SPACE_NAME
:聊天室的name
字段中的 ID。您可以通过调用ListSpaces()
方法或从聊天室的网址中获取此 ID。MESSAGE-ID
:消息的名称,以custom-
开头。必须与 Chat 应用在指定聊天室中创建的任何其他消息名称不同。
问题排查
当 Google Chat 应用或卡片返回错误时,Chat 界面会显示“出了点问题”消息。或“无法处理您的请求”。有时,Chat 界面不会显示任何错误消息,但 Chat 应用或卡片会产生意外结果;例如,卡片消息可能不会显示。
虽然 Chat 界面中可能不会显示错误消息,但当您为 Chat 应用开启错误日志记录功能时,描述性错误消息和日志数据可帮助您修正错误。如需有关查看、调试和修复错误的帮助,请参阅排查和修复 Google Chat 错误。
相关主题
- 使用卡片制作工具为 Chat 应用设计和预览 JSON 卡片消息。
- 设置邮件格式。
- 获取有关消息的详细信息。
- 列出聊天室中的消息。
- 更新消息。
- 删除消息。
- 识别 Google Chat 消息中的用户。
- 使用传入的网络钩子向 Google Chat 发送消息。