См. полный исходный код примера
В этом примере показано, как настроить маркеры следующими способами: добавить текст заголовка, масштабировать маркер, изменить цвет фона, изменить цвет границы, изменить цвет глифа, скрыть глиф.
Машинопись
const parser = new DOMParser(); async function initMap() { // Request needed libraries. const { Map } = await google.maps.importLibrary("maps") as google.maps.MapsLibrary; const { AdvancedMarkerElement, PinElement } = await google.maps.importLibrary("marker") as google.maps.MarkerLibrary; const map = new Map(document.getElementById('map') as HTMLElement, { center: { lat: 37.419, lng: -122.02 }, zoom: 14, mapId: '4504f8b37365c3d0', }); // Each PinElement is paired with a MarkerView to demonstrate setting each parameter. // Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: 'Title text for the marker at lat: 37.419, lng: -122.03', }); // Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, }); // Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, }); // Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, }); // Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, }); const pinTextGlyph = new PinElement({ glyph: 'T', glyphColor: 'white', }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, }); // Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, }); } initMap();
JavaScript
const parser = new DOMParser(); async function initMap() { // Request needed libraries. const { Map } = await google.maps.importLibrary("maps"); const { AdvancedMarkerElement, PinElement } = await google.maps.importLibrary( "marker", ); const map = new Map(document.getElementById("map"), { center: { lat: 37.419, lng: -122.02 }, zoom: 14, mapId: "4504f8b37365c3d0", }); // Each PinElement is paired with a MarkerView to demonstrate setting each parameter. // Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", }); // Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, }); // Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, }); // Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, }); // Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, }); const pinTextGlyph = new PinElement({ glyph: "T", glyphColor: "white", }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, }); // Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, }); } initMap();
CSS
/* * Always set the map height explicitly to define the size of the div element * that contains the map. */ #map { height: 100%; } /* * Optional: Makes the sample page fill the window. */ html, body { height: 100%; margin: 0; padding: 0; }
HTML
<html> <head> <title>Advanced Marker Basic Customization</title> <link rel="stylesheet" type="text/css" href="./style.css" /> <script type="module" src="./index.js"></script> </head> <body> <div id="map"></div> <!-- prettier-ignore --> <script>(g=>{var h,a,k,p="The Google Maps JavaScript API",c="google",l="importLibrary",q="__ib__",m=document,b=window;b=b[c]||(b[c]={});var d=b.maps||(b.maps={}),r=new Set,e=new URLSearchParams,u=()=>h||(h=new Promise(async(f,n)=>{await (a=m.createElement("script"));e.set("libraries",[...r]+"");for(k in g)e.set(k.replace(/[A-Z]/g,t=>"_"+t[0].toLowerCase()),g[k]);e.set("callback",c+".maps."+q);a.src=`https://maps.${c}apis.com/maps/api/js?`+e;d[q]=f;a.onerror=()=>h=n(Error(p+" could not load."));a.nonce=m.querySelector("script[nonce]")?.nonce||"";m.head.append(a)}));d[l]?console.warn(p+" only loads once. Ignoring:",g):d[l]=(f,...n)=>r.add(f)&&u().then(()=>d[l](f,...n))}) ({key: "AIzaSyB41DRUbKWJHPxaFjMAwdrzWzbVKartNGg", v: "weekly"});</script> </body> </html>
Попробуйте образец
Расширенные маркеры используют два класса для определения маркеров: класс AdvancedMarkerElement
предоставляет основные параметры ( position
, title
и map
), а класс PinElement
содержит параметры для дальнейшей настройки.
Чтобы добавить маркеры на карту, необходимо сначала загрузить библиотеку marker
, которая предоставляет классы AdvancedMarkerElement
и PinElement
.
В следующем фрагменте показан код для создания нового PinElement
и его применения к маркеру.
// Create a pin element.
const pin = new PinElement({
scale: 1.5,
});
// Create a marker and apply the element.
const marker = new AdvancedMarkerElement({
map,
position: { lat: 37.419, lng: -122.02 },
content: pin.element,
});
В картах, созданных с использованием HTML, основные параметры маркера объявляются с помощью HTML-элемента gmp-advanced-marker
; любая настройка, использующая класс PinElement
, должна применяться программно. Для этого ваш код должен получить элементы gmp-advanced-marker
со страницы HTML. В следующем фрагменте показан код для запроса коллекции элементов gmp-advanced-marker
, а затем перебор результатов для применения настроек, объявленных в PinElement
.
// Return an array of markers.
const advancedMarkers = [...document.querySelectorAll('gmp-advanced-marker')];
// Loop through the markers
for (let i = 0; i < advancedMarkers.length; i++) {
const pin = new PinElement({
scale: 2.0,
});
marker.appendChild(pin.element);
}
На этой странице показано, как настроить маркеры следующими способами:
- Добавить текст заголовка
- Масштабировать маркер
- Изменить цвет фона
- Изменить цвет границы
- Изменить цвет глифа
- Использование текста в глифе
- Скрыть глиф
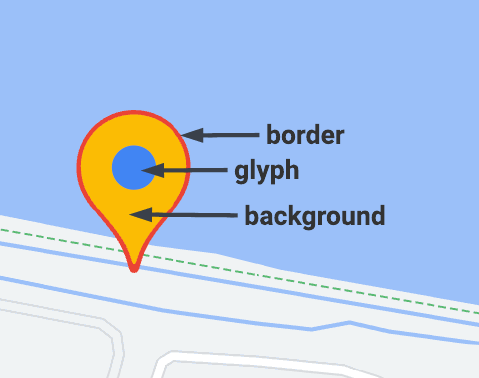
Добавить текст заголовка
Текст заголовка появляется при наведении курсора на маркер. Текст заголовка доступен для чтения программами чтения с экрана.
Чтобы добавить текст заголовка программно, используйте опцию AdvancedMarkerElement.title
:
// Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
Чтобы добавить текст заголовка к маркеру, созданному с помощью HTML, используйте атрибут title
:
<gmp-map center="43.4142989,-124.2301242" zoom="4" map-id="DEMO_MAP_ID" style="height: 400px" > <gmp-advanced-marker position="37.4220656,-122.0840897" title="Mountain View, CA" ></gmp-advanced-marker> <gmp-advanced-marker position="47.648994,-122.3503845" title="Seattle, WA" ></gmp-advanced-marker> </gmp-map>
Масштабировать маркер
Чтобы масштабировать маркер, используйте опцию scale
.
Машинопись
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
JavaScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
Изменить цвет фона
Используйте параметр PinElement.background
, чтобы изменить цвет фона маркера:
Машинопись
// Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
JavaScript
// Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
Изменить цвет границы
Используйте параметр PinElement.borderColor
, чтобы изменить цвет границы маркера:
Машинопись
// Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, });
JavaScript
// Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, });
Изменение цвета глифа
Используйте параметр PinElement.glyphColor
, чтобы изменить цвет глифа маркера:
Машинопись
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, });
JavaScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, });
Добавление текста в глиф
Используйте параметр PinElement.glyph
, чтобы заменить глиф по умолчанию текстовым символом. Текстовый глиф PinElement
масштабируется вместе с PinElement
, а его цвет по умолчанию соответствует glyphColor
по умолчанию PinElement
.
Машинопись
const pinTextGlyph = new PinElement({ glyph: 'T', glyphColor: 'white', }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, });
JavaScript
const pinTextGlyph = new PinElement({ glyph: "T", glyphColor: "white", }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, });
Скрыть глиф
Установите для параметра PinElement.glyph
пустую строку, чтобы скрыть глиф маркера:
Машинопись
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, });
JavaScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, });
Альтернативно, установите для PinElement.glyphColor
то же значение, что и для PinElement.background
. Это позволяет визуально скрыть глиф.