Zobacz pełny przykładowy kod źródłowy
W tym przykładzie pokazano, jak dostosować znaczniki na różne sposoby: dodawać tekst tytułowy, skalować znacznik, zmieniać kolor tła, zmieniać kolor obramowania, zmieniać kolor glifu, ukrywać glif.
TypeScript
const parser = new DOMParser(); async function initMap() { // Request needed libraries. const { Map } = await google.maps.importLibrary("maps") as google.maps.MapsLibrary; const { AdvancedMarkerElement, PinElement } = await google.maps.importLibrary("marker") as google.maps.MarkerLibrary; const map = new Map(document.getElementById('map') as HTMLElement, { center: { lat: 37.419, lng: -122.02 }, zoom: 14, mapId: '4504f8b37365c3d0', }); // Each PinElement is paired with a MarkerView to demonstrate setting each parameter. // Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: 'Title text for the marker at lat: 37.419, lng: -122.03', }); // Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, }); // Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, }); // Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, }); // Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, }); const pinTextGlyph = new PinElement({ glyph: 'T', glyphColor: 'white', }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, }); // Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, }); } initMap();
JavaScript
const parser = new DOMParser(); async function initMap() { // Request needed libraries. const { Map } = await google.maps.importLibrary("maps"); const { AdvancedMarkerElement, PinElement } = await google.maps.importLibrary( "marker", ); const map = new Map(document.getElementById("map"), { center: { lat: 37.419, lng: -122.02 }, zoom: 14, mapId: "4504f8b37365c3d0", }); // Each PinElement is paired with a MarkerView to demonstrate setting each parameter. // Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", }); // Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, }); // Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, }); // Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, }); // Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, }); const pinTextGlyph = new PinElement({ glyph: "T", glyphColor: "white", }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, }); // Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, }); } initMap();
CSS
/* * Always set the map height explicitly to define the size of the div element * that contains the map. */ #map { height: 100%; } /* * Optional: Makes the sample page fill the window. */ html, body { height: 100%; margin: 0; padding: 0; }
HTML
<html> <head> <title>Advanced Marker Basic Customization</title> <link rel="stylesheet" type="text/css" href="./style.css" /> <script type="module" src="./index.js"></script> </head> <body> <div id="map"></div> <!-- prettier-ignore --> <script>(g=>{var h,a,k,p="The Google Maps JavaScript API",c="google",l="importLibrary",q="__ib__",m=document,b=window;b=b[c]||(b[c]={});var d=b.maps||(b.maps={}),r=new Set,e=new URLSearchParams,u=()=>h||(h=new Promise(async(f,n)=>{await (a=m.createElement("script"));e.set("libraries",[...r]+"");for(k in g)e.set(k.replace(/[A-Z]/g,t=>"_"+t[0].toLowerCase()),g[k]);e.set("callback",c+".maps."+q);a.src=`https://maps.${c}apis.com/maps/api/js?`+e;d[q]=f;a.onerror=()=>h=n(Error(p+" could not load."));a.nonce=m.querySelector("script[nonce]")?.nonce||"";m.head.append(a)}));d[l]?console.warn(p+" only loads once. Ignoring:",g):d[l]=(f,...n)=>r.add(f)&&u().then(()=>d[l](f,...n))}) ({key: "AIzaSyB41DRUbKWJHPxaFjMAwdrzWzbVKartNGg", v: "weekly"});</script> </body> </html>
Zobacz próbkę
Zaawansowane znaczniki używają 2 klas do ich definiowania: klasa AdvancedMarkerElement
zawiera parametry podstawowe (position
, title
i map
), a klasa PinElement
zawiera opcje umożliwiające dalsze dostosowanie.
Aby dodać znaczniki do mapy, musisz najpierw wczytać bibliotekę marker
, która udostępnia klasy AdvancedMarkerElement
i PinElement
.
Poniższy fragment kodu służy do tworzenia nowych elementów PinElement
, a potem do ich stosowania do znacznika.
// Create a pin element.
const pin = new PinElement({
scale: 1.5,
});
// Create a marker and apply the element.
const marker = new AdvancedMarkerElement({
map,
position: { lat: 37.419, lng: -122.02 },
content: pin.element,
});
W mapach utworzonych przy użyciu kodu HTML podstawowe parametry znacznika są deklarowane za pomocą elementu HTML gmp-advanced-marker
. Wszelkie dostosowania korzystające z klasy PinElement
muszą być stosowane automatycznie. Aby to zrobić, kod musi pobrać elementy gmp-advanced-marker
ze strony HTML. Ten fragment kodu zawiera kod do zapytania o kolekcję elementów gmp-advanced-marker
, a następnie przetwarza wyniki, aby zastosować do nich dostosowywanie zadeklarowane w elementach PinElement
.
// Return an array of markers.
const advancedMarkers = [...document.querySelectorAll('gmp-advanced-marker')];
// Loop through the markers
for (let i = 0; i < advancedMarkers.length; i++) {
const pin = new PinElement({
scale: 2.0,
});
marker.appendChild(pin.element);
}
Na tej stronie opisano następujące sposoby dostosowywania znaczników:
- Dodawanie tekstu tytułu
- Skalowanie znacznika
- Zmienianie koloru tła
- Zmiana koloru obramowania
- Zmienianie koloru glifu
- Używanie tekstu w glifie
- Ukrywanie glifu
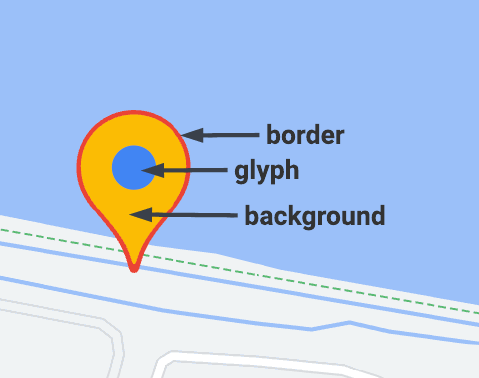
Dodaj tekst tytułu
Tekst tytułu pojawia się, gdy kursor najedzie na znacznik. Tekst tytułu jest czytelny dla czytników ekranu.
Aby dodać tekst tytułu programowo, użyj opcji AdvancedMarkerElement.title
:
// Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
Aby dodać tekst tytułu do znacznika utworzonego za pomocą kodu HTML, użyj atrybutu title
:
<gmp-map center="43.4142989,-124.2301242" zoom="4" map-id="DEMO_MAP_ID" style="height: 400px" > <gmp-advanced-marker position="37.4220656,-122.0840897" title="Mountain View, CA" ></gmp-advanced-marker> <gmp-advanced-marker position="47.648994,-122.3503845" title="Seattle, WA" ></gmp-advanced-marker> </gmp-map>
Skalowanie znacznika
Aby zmienić skalę znacznika, użyj opcji scale
.
TypeScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
JavaScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
Zmiana koloru tła
Aby zmienić kolor tła znacznika, użyj opcji PinElement.background
:
TypeScript
// Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
JavaScript
// Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
Zmiana koloru obramowania
Aby zmienić kolor obramowania znacznika, użyj opcji PinElement.borderColor
:
TypeScript
// Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, });
JavaScript
// Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, });
Zmiana koloru glifu
Aby zmienić kolor glifu znacznika, użyj opcji PinElement.glyphColor
:
TypeScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, });
JavaScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, });
Dodawanie tekstu do glifu
Użyj opcji PinElement.glyph
, aby zastąpić domyślny glif znakiem tekstowym. Glif tekstu elementu PinElement
skaluje się do wartości PinElement
, a jej kolor domyślny odpowiada domyślnemu kolorowi glyphColor
elementu PinElement
.
TypeScript
const pinTextGlyph = new PinElement({ glyph: 'T', glyphColor: 'white', }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, });
JavaScript
const pinTextGlyph = new PinElement({ glyph: "T", glyphColor: "white", }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, });
Ukrywanie znaku
Aby ukryć symbol znacznika, ustaw opcję PinElement.glyph
na pusty ciąg znaków:
TypeScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, });
JavaScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, });
Możesz też ustawić wartość PinElement.glyphColor
na taką samą jak wartość PinElement.background
.
W efekcie glif jest niewidoczny.