סמנים מתקדמים משתמשים בשתי מחלקות כדי להגדיר סמנים: המחלקה AdvancedMarkerElement
מספקת ברירת מחדל לפונקציונליות של סמנים, ו-PinElement
מכילה אפשרויות להתאמה אישית נוספת. דף זה מסביר כיצד להתאים אישית סמנים בדרכים הבאות:
- הוספת טקסט לכותרת
- שינוי גודל הסמן
- איך משנים את צבע הרקע
- שינוי צבע הגבול
- שינוי צבע הגליף
- הסתרת הגליף
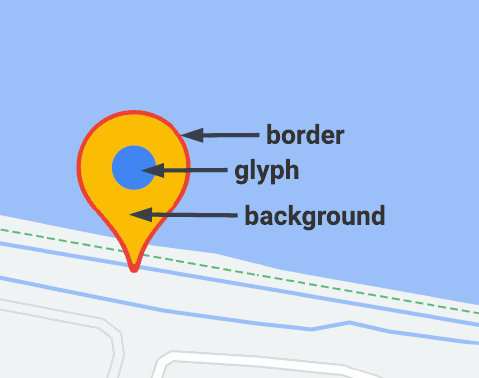
הוספת טקסט לכותרת
טקסט הכותרת מופיע כשהסמן מעביר את העכבר מעל לסמן. קוראי מסך יכולים לקרוא את טקסט הכותרת. השתמש באפשרות AdvancedMarkerElement.title
כדי להוסיף טקסט כותרת לסמן:
TypeScript
// Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: 'Title text for the marker at lat: 37.419, lng: -122.03', });
JavaScript
// Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
שינוי גודל הסמן
שימוש באפשרות PinElement.scale
כדי לשנות את קנה המידה של סמן:
TypeScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
JavaScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
איך משנים את צבע הרקע
שימוש באפשרות PinElement.background
כדי לשנות את צבע הרקע של סמן:
TypeScript
// Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
JavaScript
// Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
שינוי צבע הגבול
השתמש באפשרות PinElement.borderColor
כדי לשנות את צבע הגבול של סמן:
TypeScript
// Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.03 }, content: pinBorder.element, });
JavaScript
// Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.03 }, content: pinBorder.element, });
שינוי צבע הגליף
השתמש באפשרות PinElement.glyphColor
כדי לשנות את צבע הגליף של סמן:
TypeScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.02 }, content: pinGlyph.element, });
JavaScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.02 }, content: pinGlyph.element, });
הסתרת הגליף
יש להגדיר את האפשרות PinElement.glyph
למחרוזת ריקה כדי להסתיר את הגליף של הסמן:
TypeScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.01 }, content: pinNoGlyph.element, });
JavaScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.01 }, content: pinNoGlyph.element, });
לחלופין, מגדירים את PinElement.glyphColor
לאותו ערך כמו PinElement.background
.
כך התוצאה של הסתרה חזותית של הגליף.