簡介
InfoWindow
會在地圖上方特定位置的彈出式視窗中顯示內容 (通常為文字或圖片)。資訊視窗是由一個內容區域和一個錐形柄所組成,錐形柄的尖端會連接地圖上的指定位置。資訊視窗會以對話方塊的形式顯示在螢幕閱讀器中。
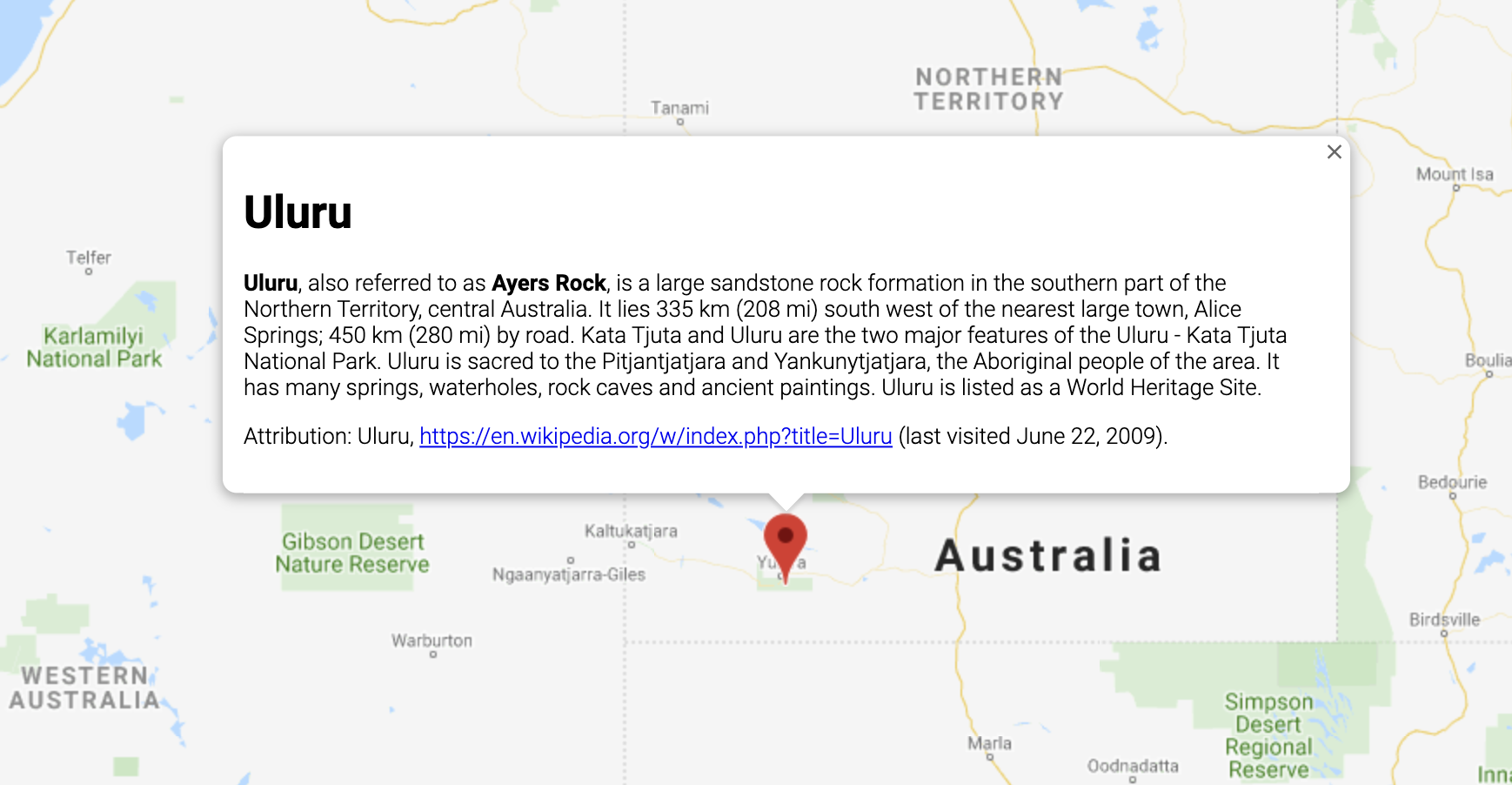
一般來說,您可以將資訊視窗附加至標記,但也可以附加至特定經緯度,方法請參閱下方有關新增資訊視窗的章節。
概括而言,資訊視窗是一種疊加層。如要進一步瞭解其他類型的疊加層,請參閱「在地圖上繪圖」一文。
新增資訊視窗
InfoWindow
建構函式會採用
InfoWindowOptions
物件常值,指定用於顯示資訊視窗的初始參數。
InfoWindowOptions
物件常值包含下列欄位:
content
:包含要在資訊視窗中顯示的一串文字或一個 DOM 節點。pixelOffset
:包含從資訊視窗尖端到資訊視窗錨定位置之間的位移值。實際上,您不必指定這個欄位,保留預設值即可。position
:包含資訊視窗錨定位置的LatLng
。請注意,InfoWindow
可以附加至Marker
物件 (這種情況下,資訊視窗位置取決於標記位置),或是附加至地圖上指定的LatLng
。其中一個擷取LatLng
方式就是使用地理編碼服務。在標記上開啟資訊視窗時,系統會自動更新position
。maxWidth
:指定資訊視窗的寬度上限 (以像素為單位)。根據預設,資訊視窗會隨內容擴展,如果資訊視窗填滿整張地圖,文字會自動換行。如果您加入maxWidth
,資訊視窗就會自動換行,強制執行指定的寬度。資訊視窗尺寸達到寬度上限後,如果畫面還有多餘的垂直空間,資訊視窗可能就會垂直擴展。
InfoWindow
的內容可以包含一串文字、一段 HTML 程式碼或一個 DOM 元素。如要設定內容,請在 InfoWindowOptions
內指定,或是在 InfoWindow
上明確呼叫 setContent()
。
如要明確調整內容尺寸,您可以將內容放入 <div>
元素中,然後使用 CSS 設定 <div>
的樣式。此外,您還可以使用 CSS 啟用捲動功能。請注意,如果沒有啟用捲動功能,則當內容超過資訊視窗的可用空間時,可能就會「溢出」資訊視窗。
開啟資訊視窗
資訊視窗建立後,不會自動顯示在地圖上。如要顯示資訊視窗,您必須在 InfoWindow
上呼叫 open()
方法,並傳遞 InfoWindowOpenOptions
物件常值來指定下列選項:
map
:指定要開啟資訊視窗的地圖或街景服務全景。anchor
:包含錨點 (例如Marker
)。如果anchor
選項為null
或未定義,資訊視窗就會在position
屬性指定的位置開啟。
TypeScript
// This example displays a marker at the center of Australia. // When the user clicks the marker, an info window opens. function initMap(): void { const uluru = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: uluru, } ); const contentString = '<div id="content">' + '<div id="siteNotice">' + "</div>" + '<h1 id="firstHeading" class="firstHeading">Uluru</h1>' + '<div id="bodyContent">' + "<p><b>Uluru</b>, also referred to as <b>Ayers Rock</b>, is a large " + "sandstone rock formation in the southern part of the " + "Northern Territory, central Australia. It lies 335 km (208 mi) " + "south west of the nearest large town, Alice Springs; 450 km " + "(280 mi) by road. Kata Tjuta and Uluru are the two major " + "features of the Uluru - Kata Tjuta National Park. Uluru is " + "sacred to the Pitjantjatjara and Yankunytjatjara, the " + "Aboriginal people of the area. It has many springs, waterholes, " + "rock caves and ancient paintings. Uluru is listed as a World " + "Heritage Site.</p>" + '<p>Attribution: Uluru, <a href="https://en.wikipedia.org/w/index.php?title=Uluru&oldid=297882194">' + "https://en.wikipedia.org/w/index.php?title=Uluru</a> " + "(last visited June 22, 2009).</p>" + "</div>" + "</div>"; const infowindow = new google.maps.InfoWindow({ content: contentString, ariaLabel: "Uluru", }); const marker = new google.maps.Marker({ position: uluru, map, title: "Uluru (Ayers Rock)", }); marker.addListener("click", () => { infowindow.open({ anchor: marker, map, }); }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example displays a marker at the center of Australia. // When the user clicks the marker, an info window opens. function initMap() { const uluru = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: uluru, }); const contentString = '<div id="content">' + '<div id="siteNotice">' + "</div>" + '<h1 id="firstHeading" class="firstHeading">Uluru</h1>' + '<div id="bodyContent">' + "<p><b>Uluru</b>, also referred to as <b>Ayers Rock</b>, is a large " + "sandstone rock formation in the southern part of the " + "Northern Territory, central Australia. It lies 335 km (208 mi) " + "south west of the nearest large town, Alice Springs; 450 km " + "(280 mi) by road. Kata Tjuta and Uluru are the two major " + "features of the Uluru - Kata Tjuta National Park. Uluru is " + "sacred to the Pitjantjatjara and Yankunytjatjara, the " + "Aboriginal people of the area. It has many springs, waterholes, " + "rock caves and ancient paintings. Uluru is listed as a World " + "Heritage Site.</p>" + '<p>Attribution: Uluru, <a href="https://en.wikipedia.org/w/index.php?title=Uluru&oldid=297882194">' + "https://en.wikipedia.org/w/index.php?title=Uluru</a> " + "(last visited June 22, 2009).</p>" + "</div>" + "</div>"; const infowindow = new google.maps.InfoWindow({ content: contentString, ariaLabel: "Uluru", }); const marker = new google.maps.Marker({ position: uluru, map, title: "Uluru (Ayers Rock)", }); marker.addListener("click", () => { infowindow.open({ anchor: marker, map, }); }); } window.initMap = initMap;
測試範例程式碼
下例會設定資訊視窗的 maxWidth
:查看範例。
將焦點放在資訊視窗
如要將焦點放在資訊視窗,請呼叫 focus()
方法。建議先搭配 visible
事件使用這個方法,再設定焦點。在未顯示的資訊視窗中呼叫這個方法,不會產生任何效果。呼叫 open()
即可顯示資訊視窗。
關閉資訊視窗
根據預設,資訊視窗會保持開啟狀態,直到使用者按下關閉控制項 (資訊視窗右上角的十字圖示) 或 ESC 鍵為止。此外,您也可以呼叫 close()
方法來明確關閉資訊視窗。
資訊視窗關閉時,焦點會移回資訊視窗開啟前位於焦點的元素。如果沒有這類元素,焦點就會移回地圖。如要覆寫這個行為,您可以監聽 closeclick
事件並手動管理焦點,如下例所示:
infoWindow.addListener('closeclick', ()=>{ // Handle focus manually. });
移動資訊視窗
您可以透過下列幾種方法變更資訊視窗的位置:
- 在資訊視窗上呼叫
setPosition()
- 使用
InfoWindow.open()
方法將資訊視窗附加至新標記。注意:如果您在呼叫open()
時未傳遞任何標記,InfoWindow
就會使用建構時透過InfoWindowOptions
物件常值指定的位置。
自訂
InfoWindow
類別不提供自訂功能。如要瞭解如何建立完全自訂的彈出式視窗,請參閱自訂彈出式視窗範例。