В Advanced Markers API используется два класса для определения маркеров: AdvancedMarkerView
обеспечивает стандартные функции, а PinView
содержит параметры для дальнейшей настройки. В этой статье рассказывается, как выполнить следующие действия:
- добавить название;
- масштабировать маркер;
- изменить цвет фона;
- изменить цвет контура;
- изменить цвет глифа;
- скрыть глиф.
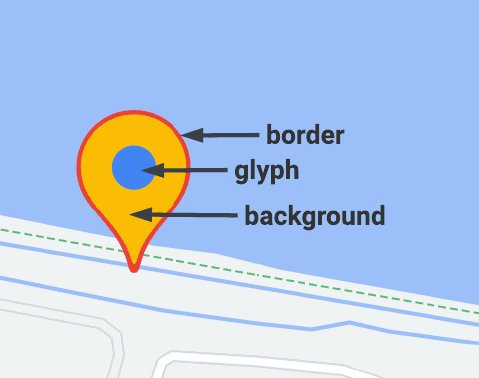
Как добавить название
Название показывается при наведении указателя мыши на маркер. Этот текст доступен для программ чтения с экрана. Чтобы добавить для маркера название, используйте атрибут AdvancedMarkerView.title
, как показано ниже.
TypeScript
// Default marker with title text (no PinView). const markerViewWithText = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.03 }, title: 'Title text for the marker at lat: 37.419, lng: -122.03', });
JavaScript
// Default marker with title text (no PinView). const markerViewWithText = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
Как масштабировать маркер
Чтобы масштабировать маркер, используйте атрибут PinView.scale
.
TypeScript
// Adjust the scale. const pinViewScaled = new google.maps.marker.PinView({ scale: 1.5, }); const markerViewScaled = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.02 }, content: pinViewScaled.element, });
JavaScript
// Adjust the scale. const pinViewScaled = new google.maps.marker.PinView({ scale: 1.5, }); const markerViewScaled = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.02 }, content: pinViewScaled.element, });
Как изменить цвет фона
Чтобы изменить цвет фона маркера, используйте атрибут PinView.background
.
TypeScript
// Change the background color. const pinViewBackground = new google.maps.marker.PinView({ background: '#FBBC04', }); const markerViewBackground = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.01 }, content: pinViewBackground.element, });
JavaScript
// Change the background color. const pinViewBackground = new google.maps.marker.PinView({ background: "#FBBC04", }); const markerViewBackground = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.01 }, content: pinViewBackground.element, });
Как изменить цвет контура
Чтобы изменить цвет контура маркера, используйте атрибут PinView.borderColor
.
TypeScript
// Change the border color. const pinViewBorder = new google.maps.marker.PinView({ borderColor: '#137333', }); const markerViewBorder = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.03 }, content: pinViewBorder.element, });
JavaScript
// Change the border color. const pinViewBorder = new google.maps.marker.PinView({ borderColor: "#137333", }); const markerViewBorder = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.03 }, content: pinViewBorder.element, });
Как изменить цвет глифа
Чтобы изменить цвет глифа маркера, используйте атрибут PinView.glyphColor
.
TypeScript
// Change the glyph color. const pinViewGlyph = new google.maps.marker.PinView({ glyphColor: 'white', }); const markerViewGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.02 }, content: pinViewGlyph.element, });
JavaScript
// Change the glyph color. const pinViewGlyph = new google.maps.marker.PinView({ glyphColor: "white", }); const markerViewGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.02 }, content: pinViewGlyph.element, });
Как скрыть глиф
Чтобы скрыть глиф маркера, укажите в качестве значения атрибута PinView.glyph
пустую строку.
TypeScript
// Hide the glyph. const pinViewNoGlyph = new google.maps.marker.PinView({ glyph: '', }); const markerViewNoGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.01 }, content: pinViewNoGlyph.element, });
JavaScript
// Hide the glyph. const pinViewNoGlyph = new google.maps.marker.PinView({ glyph: "", }); const markerViewNoGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.01 }, content: pinViewNoGlyph.element, });