Advanced Markers API 使用兩個類別來定義標記:AdvancedMarkerView
類別提供預設標記功能,PinView
則包含進一步的自訂選項。本頁說明如何透過下列方式自訂標記:
- 加入標題文字
- 縮放標記
- 變更背景顏色
- 變更邊框顏色
- 變更字符顏色
- 隱藏字符
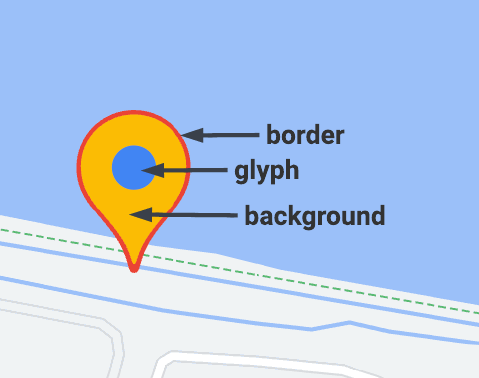
加入標題文字
標題文字會在滑鼠游標懸停在標記上時出現。標題文字會向螢幕閱讀器顯示。使用 AdvancedMarkerView.title
選項即可在標記中加入標題文字:
TypeScript
// Default marker with title text (no PinView). const markerViewWithText = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.03 }, title: 'Title text for the marker at lat: 37.419, lng: -122.03', });
JavaScript
// Default marker with title text (no PinView). const markerViewWithText = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
縮放標記
使用 PinView.scale
選項即可調整標記的縮放:
TypeScript
// Adjust the scale. const pinViewScaled = new google.maps.marker.PinView({ scale: 1.5, }); const markerViewScaled = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.02 }, content: pinViewScaled.element, });
JavaScript
// Adjust the scale. const pinViewScaled = new google.maps.marker.PinView({ scale: 1.5, }); const markerViewScaled = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.02 }, content: pinViewScaled.element, });
變更背景顏色
使用 PinView.background
選項即可變更標記的背景顏色:
TypeScript
// Change the background color. const pinViewBackground = new google.maps.marker.PinView({ background: '#FBBC04', }); const markerViewBackground = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.01 }, content: pinViewBackground.element, });
JavaScript
// Change the background color. const pinViewBackground = new google.maps.marker.PinView({ background: "#FBBC04", }); const markerViewBackground = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.01 }, content: pinViewBackground.element, });
變更邊框顏色
使用 PinView.borderColor
選項即可變更標記的邊框顏色:
TypeScript
// Change the border color. const pinViewBorder = new google.maps.marker.PinView({ borderColor: '#137333', }); const markerViewBorder = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.03 }, content: pinViewBorder.element, });
JavaScript
// Change the border color. const pinViewBorder = new google.maps.marker.PinView({ borderColor: "#137333", }); const markerViewBorder = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.03 }, content: pinViewBorder.element, });
變更字符顏色
使用 PinView.glyphColor
選項即可變更標記的字符顏色:
TypeScript
// Change the glyph color. const pinViewGlyph = new google.maps.marker.PinView({ glyphColor: 'white', }); const markerViewGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.02 }, content: pinViewGlyph.element, });
JavaScript
// Change the glyph color. const pinViewGlyph = new google.maps.marker.PinView({ glyphColor: "white", }); const markerViewGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.02 }, content: pinViewGlyph.element, });
隱藏字符
將 PinView.glyph
選項設為空白字串,即可隱藏標記字符:
TypeScript
// Hide the glyph. const pinViewNoGlyph = new google.maps.marker.PinView({ glyph: '', }); const markerViewNoGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.01 }, content: pinViewNoGlyph.element, });
JavaScript
// Hide the glyph. const pinViewNoGlyph = new google.maps.marker.PinView({ glyph: "", }); const markerViewNoGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.01 }, content: pinViewNoGlyph.element, });