Введение
Маркеры на карте обозначают выбранные вами местоположения. По умолчанию для маркера используется стандартный значок. Его можно заменить на другое, собственное изображение. Маркеры и значки – это объекты типа Marker
. Собственный значок можно установить в конструкторе маркера или вызвав в маркере метод setIcon()
. Подробнее о персонализации значков читайте ниже.
В более широком смысле маркеры представляют собой один из типов наложений. Подробнее о наложениях читайте в разделе Рисование на карте.
Маркеры – это интерактивные элементы. Например, они по умолчанию получают события 'click'
. Поэтому, добавив к ним прослушиватель событий, вы можете показывать пользователям информационное окно со своим текстом. Если свойству маркера draggable
присвоено значение true
, пользователи смогут изменять положение маркера на карте. Подробнее о перетаскиваемых маркерах читайте ниже.
Добавление маркера
Конструктор google.maps.Marker
получает один литерал объекта Marker options
, в котором заданы исходные свойства маркера.
Следующие поля особенно важны и обычно задаются при создании маркера:
-
position
(обязательное) – задает значениеLatLng
, они же координаты начального положения маркера. Получить координатыLatLng
можно, например, с помощью службы геокодирования. -
map
(необязательно) – задает объектMap
, на который следует поместить маркер. Если при создании маркера вы не укажете карту, то маркер не будет к ней прикреплен (и не будет на ней отображаться). Вы можете добавить маркер позже, вызвав для него методsetMap()
.
В примере ниже на карту добавляется простой маркер, отмечающий гору Улуру в центре Австралии.
TypeScript
function initMap(): void { const myLatLng = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: myLatLng, } ); new google.maps.Marker({ position: myLatLng, map, title: "Hello World!", }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
function initMap() { const myLatLng = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: myLatLng, }); new google.maps.Marker({ position: myLatLng, map, title: "Hello World!", }); } window.initMap = initMap;
Примеры кода
В примере выше был создан объект Marker со свойством map
.
Маркер также можно добавить прямо на карту с помощью метода setMap()
, как показано в примере ниже:
var myLatlng = new google.maps.LatLng(-25.363882,131.044922); var mapOptions = { zoom: 4, center: myLatlng } var map = new google.maps.Map(document.getElementById("map"), mapOptions); var marker = new google.maps.Marker({ position: myLatlng, title:"Hello World!" }); // To add the marker to the map, call setMap(); marker.setMap(map);
Название объекта title
будет отображаться в качестве подсказки.
Если вы не хотите задавать значения Marker options
в конструкторе маркера, задайте в последнем аргументе конструктора объект {}
.
Удаление маркера
Чтобы удалить маркер с карты, вызовите метод setMap()
и передайте с его помощью аргумент null
.
marker.setMap(null);
Учтите, что этот метод не удаляет маркер полностью, а просто убирает его с карты. Чтобы полностью удалить маркер, необходимо также задать для его объекта значение null
.
Если вы работаете с разными маркерами, создайте массив для их хранения. Вы сможете удалять маркеры из массива, по очереди вызывая метод setMap()
для каждого из них. Также маркеры можно удалить, убрав их с карты и присвоив переменной length
значение 0
(чтобы удалить все ссылки на маркеры).
Кастомизация значков маркера
Вместо стандартного значка маркера в Google Картах можно использовать графический файл или векторный значок. Вы можете добавлять на маркеры текстовые ярлыки, использовать сложные значки для выделения кликабельной области карты и задавать очередность наложения маркеров на карту.
Маркеры с растровыми изображениями
Обычно переменная icon указывает, где находится изображение, которое нужно использовать вместо стандартного значка булавки. Для этого в свойстве маркера icon
нужно задать URL изображения. Maps JavaScript API задаст нужный размер значка автоматически.
TypeScript
// This example adds a marker to indicate the position of Bondi Beach in Sydney, // Australia. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: { lat: -33, lng: 151 }, } ); const image = "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"; const beachMarker = new google.maps.Marker({ position: { lat: -33.89, lng: 151.274 }, map, icon: image, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example adds a marker to indicate the position of Bondi Beach in Sydney, // Australia. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: { lat: -33, lng: 151 }, }); const image = "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"; const beachMarker = new google.maps.Marker({ position: { lat: -33.89, lng: 151.274 }, map, icon: image, }); } window.initMap = initMap;
Примеры кода
Маркеры с векторными значками
Задать внешний вид маркера можно также с помощью векторного пути SVG. Для этого передайте литерал объекта Symbol
с векторным путем свойству маркера icon
. Собственный путь можно задать в объекте path или выбрав один из готовых путей в google.maps.SymbolPath. Свойство anchor
необходимо задавать, чтобы маркер отображался корректно при изменении масштаба. Прочитайте, как создавать маркеры и ломаные линии с помощью векторных значков (символов).
TypeScript
// This example uses SVG path notation to add a vector-based symbol // as the icon for a marker. The resulting icon is a marker-shaped // symbol with a blue fill and no border. function initMap(): void { const center = new google.maps.LatLng(-33.712451, 150.311823); const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 9, center: center, } ); const svgMarker = { path: "M-1.547 12l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM0 0q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z", fillColor: "blue", fillOpacity: 0.6, strokeWeight: 0, rotation: 0, scale: 2, anchor: new google.maps.Point(0, 20), }; new google.maps.Marker({ position: map.getCenter(), icon: svgMarker, map: map, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example uses SVG path notation to add a vector-based symbol // as the icon for a marker. The resulting icon is a marker-shaped // symbol with a blue fill and no border. function initMap() { const center = new google.maps.LatLng(-33.712451, 150.311823); const map = new google.maps.Map(document.getElementById("map"), { zoom: 9, center: center, }); const svgMarker = { path: "M-1.547 12l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM0 0q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z", fillColor: "blue", fillOpacity: 0.6, strokeWeight: 0, rotation: 0, scale: 2, anchor: new google.maps.Point(0, 20), }; new google.maps.Marker({ position: map.getCenter(), icon: svgMarker, map: map, }); } window.initMap = initMap;
Примеры кода
Метки маркеров
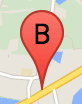
Метка маркера – это буква или число, показанное внутри маркера. На картинке рядом показан маркер с меткой в виде буквы В. Метки маркера можно задавать в виде текстовой строки или объекта MarkerLabel
, содержащего текстовую строку и другие свойства метки.
При создании маркера вы можете задать свойство label
в объекте MarkerOptions
. Другой способ — вызвать метод setLabel()
для существующего объекта Marker и установить для него метку.
В примере ниже маркеры с метками появляются, когда пользователь нажимает на карту.
TypeScript
// In the following example, markers appear when the user clicks on the map. // Each marker is labeled with a single alphabetical character. const labels = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let labelIndex = 0; function initMap(): void { const bangalore = { lat: 12.97, lng: 77.59 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 12, center: bangalore, } ); // This event listener calls addMarker() when the map is clicked. google.maps.event.addListener(map, "click", (event) => { addMarker(event.latLng, map); }); // Add a marker at the center of the map. addMarker(bangalore, map); } // Adds a marker to the map. function addMarker(location: google.maps.LatLngLiteral, map: google.maps.Map) { // Add the marker at the clicked location, and add the next-available label // from the array of alphabetical characters. new google.maps.Marker({ position: location, label: labels[labelIndex++ % labels.length], map: map, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// In the following example, markers appear when the user clicks on the map. // Each marker is labeled with a single alphabetical character. const labels = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let labelIndex = 0; function initMap() { const bangalore = { lat: 12.97, lng: 77.59 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 12, center: bangalore, }); // This event listener calls addMarker() when the map is clicked. google.maps.event.addListener(map, "click", (event) => { addMarker(event.latLng, map); }); // Add a marker at the center of the map. addMarker(bangalore, map); } // Adds a marker to the map. function addMarker(location, map) { // Add the marker at the clicked location, and add the next-available label // from the array of alphabetical characters. new google.maps.Marker({ position: location, label: labels[labelIndex++ % labels.length], map: map, }); } window.initMap = initMap;
Примеры кода
Сложные значки
Вы можете использовать сложные фигуры для очерчивания областей карты, которые должны реагировать на нажатие, и указывать, как значки должны отрисовываться относительно других слоев (порядок наложения). Для заданных таким образом значков в качестве свойства icon
следует устанавливать объекты типа Icon
.
Объекты Icon
определяют изображение. Также они определяют размер (size
), источник (origin
) значка, например когда он берется из более крупного графического файла, и место расположения значка anchor
(зависит от значения переменной origin).
Если в вашем маркере есть метка, то вы можете задать ее расположение в свойстве labelOrigin
объекта Icon
.
TypeScript
// The following example creates complex markers to indicate beaches near // Sydney, NSW, Australia. Note that the anchor is set to (0,32) to correspond // to the base of the flagpole. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 10, center: { lat: -33.9, lng: 151.2 }, } ); setMarkers(map); } // Data for the markers consisting of a name, a LatLng and a zIndex for the // order in which these markers should display on top of each other. const beaches: [string, number, number, number][] = [ ["Bondi Beach", -33.890542, 151.274856, 4], ["Coogee Beach", -33.923036, 151.259052, 5], ["Cronulla Beach", -34.028249, 151.157507, 3], ["Manly Beach", -33.80010128657071, 151.28747820854187, 2], ["Maroubra Beach", -33.950198, 151.259302, 1], ]; function setMarkers(map: google.maps.Map) { // Adds markers to the map. // Marker sizes are expressed as a Size of X,Y where the origin of the image // (0,0) is located in the top left of the image. // Origins, anchor positions and coordinates of the marker increase in the X // direction to the right and in the Y direction down. const image = { url: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png", // This marker is 20 pixels wide by 32 pixels high. size: new google.maps.Size(20, 32), // The origin for this image is (0, 0). origin: new google.maps.Point(0, 0), // The anchor for this image is the base of the flagpole at (0, 32). anchor: new google.maps.Point(0, 32), }; // Shapes define the clickable region of the icon. The type defines an HTML // <area> element 'poly' which traces out a polygon as a series of X,Y points. // The final coordinate closes the poly by connecting to the first coordinate. const shape = { coords: [1, 1, 1, 20, 18, 20, 18, 1], type: "poly", }; for (let i = 0; i < beaches.length; i++) { const beach = beaches[i]; new google.maps.Marker({ position: { lat: beach[1], lng: beach[2] }, map, icon: image, shape: shape, title: beach[0], zIndex: beach[3], }); } } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates complex markers to indicate beaches near // Sydney, NSW, Australia. Note that the anchor is set to (0,32) to correspond // to the base of the flagpole. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 10, center: { lat: -33.9, lng: 151.2 }, }); setMarkers(map); } // Data for the markers consisting of a name, a LatLng and a zIndex for the // order in which these markers should display on top of each other. const beaches = [ ["Bondi Beach", -33.890542, 151.274856, 4], ["Coogee Beach", -33.923036, 151.259052, 5], ["Cronulla Beach", -34.028249, 151.157507, 3], ["Manly Beach", -33.80010128657071, 151.28747820854187, 2], ["Maroubra Beach", -33.950198, 151.259302, 1], ]; function setMarkers(map) { // Adds markers to the map. // Marker sizes are expressed as a Size of X,Y where the origin of the image // (0,0) is located in the top left of the image. // Origins, anchor positions and coordinates of the marker increase in the X // direction to the right and in the Y direction down. const image = { url: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png", // This marker is 20 pixels wide by 32 pixels high. size: new google.maps.Size(20, 32), // The origin for this image is (0, 0). origin: new google.maps.Point(0, 0), // The anchor for this image is the base of the flagpole at (0, 32). anchor: new google.maps.Point(0, 32), }; // Shapes define the clickable region of the icon. The type defines an HTML // <area> element 'poly' which traces out a polygon as a series of X,Y points. // The final coordinate closes the poly by connecting to the first coordinate. const shape = { coords: [1, 1, 1, 20, 18, 20, 18, 1], type: "poly", }; for (let i = 0; i < beaches.length; i++) { const beach = beaches[i]; new google.maps.Marker({ position: { lat: beach[1], lng: beach[2] }, map, icon: image, shape: shape, title: beach[0], zIndex: beach[3], }); } } window.initMap = initMap;
Примеры кода
Преобразование объекта MarkerImage
в Icon
В Maps JavaScript API версий ниже 3.10 сложные значки задавались как объекты MarkerImage
. В версии 3.10 появился литерал объекта Icon
, который заменил собой MarkerImage
в версиях 3.11 и выше.
Литералы Icon
поддерживают те же параметры, что и литералы объекта MarkerImage
. Поэтому вы можете легко конвертировать объект MarkerImage
в Icon
, удалив конструктор, упаковав предыдущие параметры в {}
и присвоив каждому параметру имя. Пример:
var image = new google.maps.MarkerImage( place.icon, new google.maps.Size(71, 71), new google.maps.Point(0, 0), new google.maps.Point(17, 34), new google.maps.Size(25, 25));
Результат:
var image = { url: place.icon, size: new google.maps.Size(71, 71), origin: new google.maps.Point(0, 0), anchor: new google.maps.Point(17, 34), scaledSize: new google.maps.Size(25, 25) };
Оптимизация маркеров
Вы можете сделать так, чтобы все маркеры отрисовывались как один статический элемент, и повысить за счет этого производительность карты. Это особенно полезно для карт с большим количеством маркеров. По умолчанию за оптимизацию отвечает Maps JavaScript API. Когда на карте много маркеров, эта функция начинает применяться при их отрисовке. Если какие-либо маркеры оптимизировать не удалось, Maps JavaScript API будет отрисовывать их без оптимизации. Отключайте оптимизированную отрисовку для анимированных изображений GIF или PNG, а также в случаях, когда нужно отрисовывать каждый маркер в виде отдельного элемента DOM. В примере ниже показано, как создать оптимизированный маркер:
var marker = new google.maps.Marker({ position: myLatlng, title:"Hello World!", optimized: true });
Как открыть доступ к маркеру
Вы можете сделать маркер доступным, добавив прослушиватель кликов и присвоив переменной optimized
значение false
. За счет этого в маркер будет добавлена семантика кнопки, что сделает его доступным для команд клавиатуры, программ чтения с экрана и т. д. Чтобы открыть доступ к тексту маркера, используйте объект title
.
В примере ниже первый маркер выделяется, когда пользователь нажимает клавишу табуляции. Затем между маркерами можно перемещаться нажатием кнопок со стрелками. Повторное нажатие клавиши табуляции вызывает перемещение к следующему элементу управления карты. Если маркер выбран и у него есть информационное окно, то его можно открыть кнопкой мыши, клавишей ввода или пробелом. После закрытия информационного окна снова выделяется связанный с ним маркер.
TypeScript
// The following example creates five accessible and // focusable markers. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 12, center: { lat: 34.84555, lng: -111.8035 }, } ); // Set LatLng and title text for the markers. The first marker (Boynton Pass) // receives the initial focus when tab is pressed. Use arrow keys to // move between markers; press tab again to cycle through the map controls. const tourStops: [google.maps.LatLngLiteral, string][] = [ [{ lat: 34.8791806, lng: -111.8265049 }, "Boynton Pass"], [{ lat: 34.8559195, lng: -111.7988186 }, "Airport Mesa"], [{ lat: 34.832149, lng: -111.7695277 }, "Chapel of the Holy Cross"], [{ lat: 34.823736, lng: -111.8001857 }, "Red Rock Crossing"], [{ lat: 34.800326, lng: -111.7665047 }, "Bell Rock"], ]; // Create an info window to share between markers. const infoWindow = new google.maps.InfoWindow(); // Create the markers. tourStops.forEach(([position, title], i) => { const marker = new google.maps.Marker({ position, map, title: `${i + 1}. ${title}`, label: `${i + 1}`, optimized: false, }); // Add a click listener for each marker, and set up the info window. marker.addListener("click", () => { infoWindow.close(); infoWindow.setContent(marker.getTitle()); infoWindow.open(marker.getMap(), marker); }); }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates five accessible and // focusable markers. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 12, center: { lat: 34.84555, lng: -111.8035 }, }); // Set LatLng and title text for the markers. The first marker (Boynton Pass) // receives the initial focus when tab is pressed. Use arrow keys to // move between markers; press tab again to cycle through the map controls. const tourStops = [ [{ lat: 34.8791806, lng: -111.8265049 }, "Boynton Pass"], [{ lat: 34.8559195, lng: -111.7988186 }, "Airport Mesa"], [{ lat: 34.832149, lng: -111.7695277 }, "Chapel of the Holy Cross"], [{ lat: 34.823736, lng: -111.8001857 }, "Red Rock Crossing"], [{ lat: 34.800326, lng: -111.7665047 }, "Bell Rock"], ]; // Create an info window to share between markers. const infoWindow = new google.maps.InfoWindow(); // Create the markers. tourStops.forEach(([position, title], i) => { const marker = new google.maps.Marker({ position, map, title: `${i + 1}. ${title}`, label: `${i + 1}`, optimized: false, }); // Add a click listener for each marker, and set up the info window. marker.addListener("click", () => { infoWindow.close(); infoWindow.setContent(marker.getTitle()); infoWindow.open(marker.getMap(), marker); }); }); } window.initMap = initMap;
Примеры кода
Анимация маркера
Вы можете анимировать маркеры в ответ на определенные пользовательские действия. Задать настройки анимации можно в свойстве маркера animation
типа google.maps.Animation
. Поддерживаются следующие значения Animation
:
-
DROP
– указывает, что новый маркер должен "падать" на карту сверху. Анимация завершается, когда маркер попадает на свое место иanimation
вновь принимает значениеnull
. Этот тип анимации обычно задается при создании объектаMarker
. -
BOUNCE
– указывает, что маркер должен "подпрыгивать" на месте. Маркер будет делать это до тех пор, пока для его свойстваanimation
не будет явно установлено значениеnull
.
Чтобы запустить анимацию для существующего маркера, вызовите метод setAnimation()
для объекта Marker
.
TypeScript
// The following example creates a marker in Stockholm, Sweden using a DROP // animation. Clicking on the marker will toggle the animation between a BOUNCE // animation and no animation. let marker: google.maps.Marker; function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 13, center: { lat: 59.325, lng: 18.07 }, } ); marker = new google.maps.Marker({ map, draggable: true, animation: google.maps.Animation.DROP, position: { lat: 59.327, lng: 18.067 }, }); marker.addListener("click", toggleBounce); } function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates a marker in Stockholm, Sweden using a DROP // animation. Clicking on the marker will toggle the animation between a BOUNCE // animation and no animation. let marker; function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 13, center: { lat: 59.325, lng: 18.07 }, }); marker = new google.maps.Marker({ map, draggable: true, animation: google.maps.Animation.DROP, position: { lat: 59.327, lng: 18.067 }, }); marker.addListener("click", toggleBounce); } function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } window.initMap = initMap;
Примеры кода
Если у вас много маркеров, будет неудобно, если все они начнут "падать" на карту одновременно. Функция setTimeout()
позволяет разнести во времени анимацию маркеров. Для этого воспользуйтесь приемом из примера ниже:
function drop() { for (var i =0; i < markerArray.length; i++) { setTimeout(function() { addMarkerMethod(); }, i * 200); } }
Как сделать маркер перетаскиваемым
Пользователи смогут перетаскивать маркер по карте, если для его свойства draggable
установить значение true
.
var myLatlng = new google.maps.LatLng(-25.363882,131.044922); var mapOptions = { zoom: 4, center: myLatlng } var map = new google.maps.Map(document.getElementById("map"), mapOptions); // Place a draggable marker on the map var marker = new google.maps.Marker({ position: myLatlng, map: map, draggable:true, title:"Drag me!" });
Другие возможности кастомизации маркеров
Полные возможности кастомизации маркеров описаны в этой статье.
Узнать больше о других расширениях класса Marker, кластеризации и управлении этим классом и кастомизации наложений можно в библиотеках с открытым исходным кодом.