Advanced Markers API는 두 가지 클래스를 사용하여 마커를 정의합니다. AdvancedMarkerView
클래스는 기본 마커 기능을 제공하고 PinView
는 추가 맞춤설정을 위한 옵션을 제공합니다. 이 페이지에서는 다음과 같은 방법으로 마커를 맞춤설정하는 방법을 설명합니다.
- 제목 텍스트 추가
- 마커 크기 조정
- 배경 색상 변경
- 테두리 색상 변경
- 글리프 색상 변경
- 글리프 숨기기
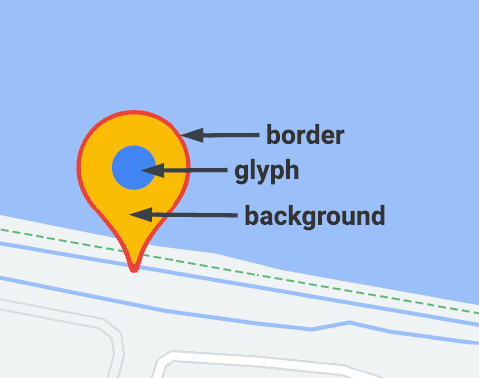
제목 텍스트 추가
커서를 마커 위로 가져가면 제목 텍스트가 표시됩니다. 제목 텍스트는 스크린 리더에 표시됩니다. 마커에 제목 텍스트를 추가하려면 AdvancedMarkerView.title
옵션을 사용합니다.
TypeScript
// Default marker with title text (no PinView). const markerViewWithText = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.03 }, title: 'Title text for the marker at lat: 37.419, lng: -122.03', });
자바스크립트
// Default marker with title text (no PinView). const markerViewWithText = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
마커 크기 조정
마커의 크기를 조정하려면 PinView.scale
옵션을 사용합니다.
TypeScript
// Adjust the scale. const pinViewScaled = new google.maps.marker.PinView({ scale: 1.5, }); const markerViewScaled = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.02 }, content: pinViewScaled.element, });
자바스크립트
// Adjust the scale. const pinViewScaled = new google.maps.marker.PinView({ scale: 1.5, }); const markerViewScaled = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.02 }, content: pinViewScaled.element, });
배경 색상 변경
마커의 배경 색상을 변경하려면 PinView.background
옵션을 사용합니다.
TypeScript
// Change the background color. const pinViewBackground = new google.maps.marker.PinView({ background: '#FBBC04', }); const markerViewBackground = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.01 }, content: pinViewBackground.element, });
자바스크립트
// Change the background color. const pinViewBackground = new google.maps.marker.PinView({ background: "#FBBC04", }); const markerViewBackground = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.419, lng: -122.01 }, content: pinViewBackground.element, });
테두리 색상 변경
마커의 테두리 색상을 변경하려면 PinView.borderColor
옵션을 사용합니다.
TypeScript
// Change the border color. const pinViewBorder = new google.maps.marker.PinView({ borderColor: '#137333', }); const markerViewBorder = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.03 }, content: pinViewBorder.element, });
자바스크립트
// Change the border color. const pinViewBorder = new google.maps.marker.PinView({ borderColor: "#137333", }); const markerViewBorder = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.03 }, content: pinViewBorder.element, });
글리프 색상 변경
마커의 글리프 색상을 변경하려면 PinView.glyphColor
옵션을 사용합니다.
TypeScript
// Change the glyph color. const pinViewGlyph = new google.maps.marker.PinView({ glyphColor: 'white', }); const markerViewGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.02 }, content: pinViewGlyph.element, });
자바스크립트
// Change the glyph color. const pinViewGlyph = new google.maps.marker.PinView({ glyphColor: "white", }); const markerViewGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.02 }, content: pinViewGlyph.element, });
글리프 숨기기
마커의 글리프를 숨기려면 PinView.glyph
옵션을 빈 문자열로 설정합니다.
TypeScript
// Hide the glyph. const pinViewNoGlyph = new google.maps.marker.PinView({ glyph: '', }); const markerViewNoGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.01 }, content: pinViewNoGlyph.element, });
자바스크립트
// Hide the glyph. const pinViewNoGlyph = new google.maps.marker.PinView({ glyph: "", }); const markerViewNoGlyph = new google.maps.marker.AdvancedMarkerView({ map, position: { lat: 37.415, lng: -122.01 }, content: pinViewNoGlyph.element, });